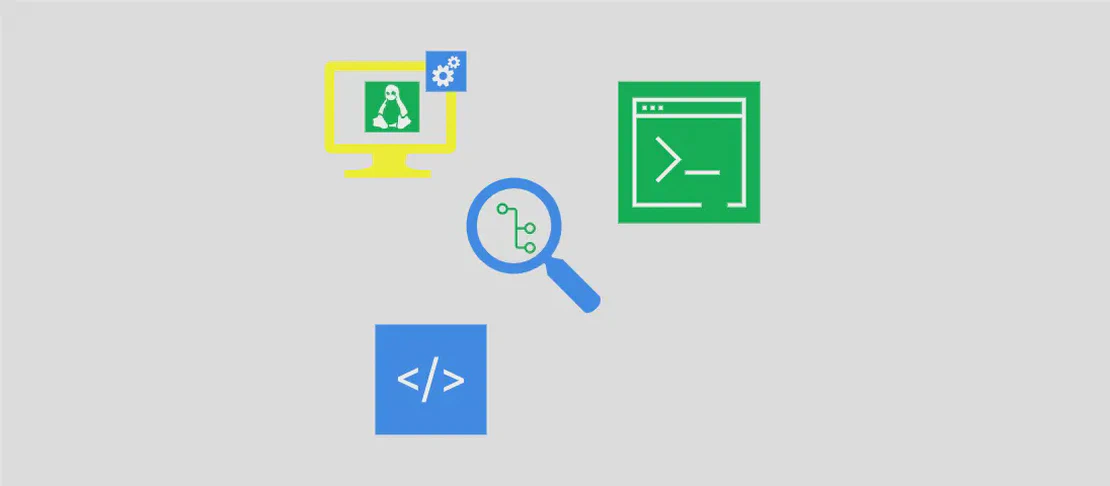
How to Use the `fd` Command (with examples)
The fd
command is a modern and user-friendly alternative to the classic find
command, designed to search for files and directories. Its goal is to be faster and more intuitive through simplified syntax and optimized performance. Unlike find
, fd
uses sensible defaults, which makes it easier for casual users while still providing powerful search capabilities for more advanced users. It’s especially appreciated for its ability to handle multiple types of filters and its smart handling of path and pattern inputs.
Use case 1: Recursively find files matching a specific pattern in the current directory
Code:
fd "string|regex"
Motivation:
At times, you may need to search through an entire directory tree for files that contain a certain string or match a regular expression. This is a common task for software developers who are attempting to locate function definitions, log files, or custom patterns within a codebase or configuration files. Traditional methods might involve complex UNIX commands, but fd
simplifies this process, making it accessible to anyone with basic command-line familiarity.
Explanation:
"string|regex"
: A required argument indicating the pattern you’re searching for. This can be a simple string or a more complex regular expression.- By default,
fd
searches recursively from the current directory, which means it will look into every subdirectory without additional flags or arguments.
Example Output:
Suppose you have a project directory with various files including logs, scripts, and documents. Running the command with a regex to find all .log
files might yield:
errors.log
access.log
query_results.log
Use case 2: Find files that begin with a specific string
Code:
fd "^string"
Motivation:
When organizing files, it’s often important to identify files that begin with a common prefix. This can be useful, for instance, when handling batches of files named by date or by specific identifiers, a process frequently used in data processing workflows, batch renaming tasks, or archival projects.
Explanation:
"^string"
: The caret symbol^
in regex denotes the start of a line. Using it before a string instructsfd
to find files whose names start with the specific string provided.
Example Output:
Executing the command in a directory of documents looking for files starting with “report” could deliver:
report_summary.pdf
report_data.csv
report_figures.docx
Use case 3: Find files with a specific extension
Code:
fd --extension txt
Motivation:
Often, you need to isolate files based on their extension, such as when organizing plaintext data files, editing code, or transferring specific media types. This capability is essential for developers, data analysts, and system administrators who routinely work with multiple file types and need a quick way to filter them.
Explanation:
--extension txt
: This option tellsfd
to filter the results based on the file extension provided. It simplifies the task of isolating files like.txt
or other specified types.
Example Output:
If run in a directory with mixed file types, the output could be:
notes.txt
todo_list.txt
meeting-agenda.txt
Use case 4: Find files in a specific directory
Code:
fd "string|regex" path/to/directory
Motivation:
Sometimes, your search needs to be confined to a specific directory instead of the entire filesystem. This could be useful when narrowing down searches to certain disks, projects, or containerized environments, optimizing for both speed and specificity.
Explanation:
"string|regex"
: Your search pattern, which can be as general or specific as necessary.path/to/directory
: Specifies the directory in which to begin searching, ensuring thatfd
only examines files within the confines of this path.
Example Output:
Using the command on a specific project directory might produce:
lib/function.js
doc/readme.md
test/spec_helper.js
Use case 5: Include ignored and hidden files in the search
Code:
fd --hidden --no-ignore "string|regex"
Motivation:
By default, fd
respects .gitignore
rules and ignores hidden files, which is convenient for many tasks. However, advanced users might need to include these files to ensure comprehensive searches, especially when dealing with nuanced debugging, thorough code auditing, or configuration file edits.
Explanation:
--hidden
: Instructsfd
to include hidden files and directories (those beginning with a dot.
).--no-ignore
: Forcesfd
to ignore rules outlined in.gitignore
,.ignore
, or other similar files, providing a complete view of all files in a directory.
Example Output:
Searching through a configured project directory might reveal files like:
.git/config
.env
.hidden_file.txt
Use case 6: Execute a command on each search result returned
Code:
fd "string|regex" --exec command
Motivation:
Automating repetitive tasks can save significant time and reduces error margins, especially in bulk file operations. By executing commands directly on search results, users can streamline workflows, applying edits, copies, backups, or transfers en masse.
Explanation:
"string|regex"
: The search pattern targeting specific files.--exec command
: Specifies the command to execute on each file found. This allows automation, like renaming files, changing permissions, or moving them to another directory.
Example Output:
If you need to count lines in all .log
files found, running a command like wc -l
via --exec
will output:
1234 errors.log
56789 access.log
42 query_results.log
Conclusion:
The fd
command transforms and streamlines file searching and manipulation tasks within the command line. Its speed and simplicity open up its capabilities to users ranging from beginner hobbyists to seasoned IT professionals. With versatile options to search by pattern, extension, and directory, and execute actions on results, fd
exemplifies modern command-line functionality tailored for efficiency and user-friendliness.