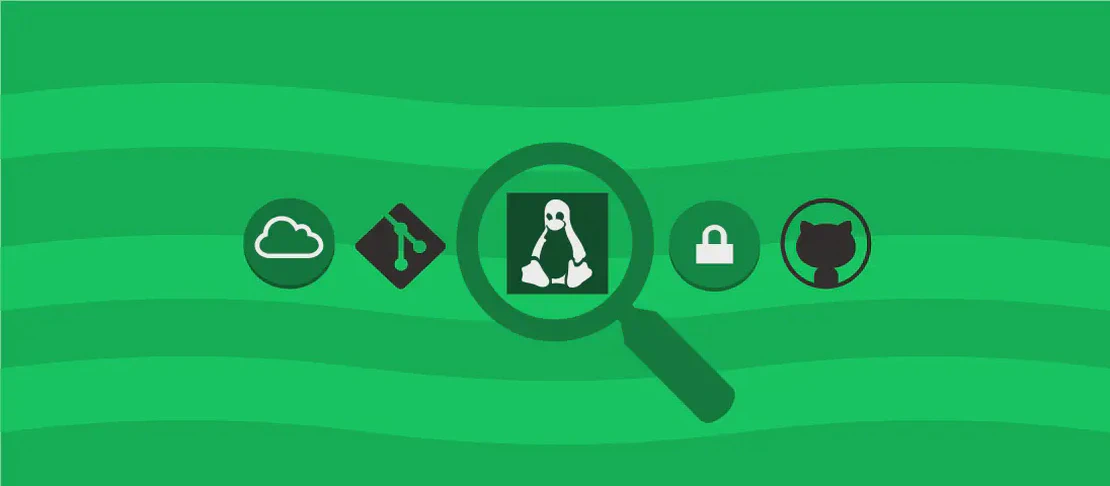
How to use the command 'ffmpeg' (with examples)
FFmpeg is a powerful multimedia framework widely used for handling video, audio, and other multimedia files and streams. It is a key tool for video conversion, manipulation, compression, and streaming across various platforms. Command-line based, it allows for precise control over a wide range of parameters when processing media files. FFmpeg is highly popular due to its flexibility, speed, and comprehensive support for different formats.
Use case 1: Extract the sound from a video and save it as MP3
Code:
ffmpeg -i path/to/video.mp4 -vn path/to/sound.mp3
Motivation:
Often, you might want to extract audio from a video file to create a soundtrack or to save data by discarding video content, especially when only the audio portion is of interest. This could be useful for creating podcasts from a recorded video interview, or extracting songs from a concert video.
Explanation:
-i path/to/video.mp4
: Specifies the input video file from which audio will be extracted. The path and filename represent the source media file.-vn
: Tells ffmpeg to ignore the video stream, effectively focusing only on the audio components during the extraction.path/to/sound.mp3
: Indicates the destination file with the output format as MP3, a common audio file format supported by most devices and platforms.
Example output:
A separate audio file in MP3 format containing the sound previously embedded in the input video file, devoid of any video data.
Use case 2: Transcode a FLAC file to Red Book CD format (44100kHz, 16bit)
Code:
ffmpeg -i path/to/input_audio.flac -ar 44100 -sample_fmt s16 path/to/output_audio.wav
Motivation:
This is necessary when you want to convert a high-quality FLAC audio file into a CD compatible format. It is very useful for producing audio CDs from high-resolution music files that are stored in FLAC, enabling playback on traditional CD players.
Explanation:
-i path/to/input_audio.flac
: Denotes the input audio file in FLAC format, renowned for its lossless audio compression.-ar 44100
: Specifies the audio sampling rate to 44,100 Hz, which is the standard for audio CDs according to the Red Book specification.-sample_fmt s16
: Sets the audio sample format to 16-bit, ensuring compatibility with the CD format’s bit depth requirements.path/to/output_audio.wav
: Provides the output path and file name for the newly transcoded audio in WAV format, which is compatible with audio CDs.
Example output:
A WAV file with CD-standard audio quality transformed from a high-definition FLAC file.
Use case 3: Save a video as GIF, scaling the height to 1000px and setting framerate to 15
Code:
ffmpeg -i path/to/video.mp4 -vf 'scale=-1:1000' -r 15 path/to/output.gif
Motivation:
Creating GIFs from video clips is common for social media use, embedding simple animations in websites, or sharing short clips in messaging apps. Scaling and adjusting the frame rate are important for optimizing the size and quality of GIFs.
Explanation:
-i path/to/video.mp4
: Points to the input video file intended for conversion into a GIF.-vf 'scale=-1:1000'
: Uses a video filter to scale the video, maintaining the aspect ratio by setting the width to auto (-1
) while adjusting the height to 1000 pixels.-r 15
: Sets the frame rate to 15 frames per second, balancing quality and file size for smooth playback in GIF format.path/to/output.gif
: Specifies the file name and format for the resulting GIF.
Example output:
A GIF file created from the original video with a height of 1000 pixels, playing at a frame rate of 15 fps.
Use case 4: Combine numbered images (frame_1.jpg
, frame_2.jpg
, etc) into a video or GIF
Code:
ffmpeg -i path/to/frame_%d.jpg -f image2 video.mpg|video.gif
Motivation:
This method is handy when you have a sequence of images from time-lapse photography or animation frames that you want to combine into a single video or animation. It automates the process of converting static images to a dynamic presentation.
Explanation:
-i path/to/frame_%d.jpg
: Uses numbered images as inputs, where%d
is a placeholder for an incrementing number corresponding to the image sequence.-f image2
: Indicates the image file demuxer, preparing the input for video creation.video.mpg|video.gif
: Sets the output video format to either MPG or GIF, allowing flexibility in the type of video or animation required.
Example output:
A video file (MPG) or animation (GIF) smoothly showcasing the progression defined by the sequence of input images.
Use case 5: Trim a video from a given start time mm:ss to an end time mm2:ss2
Code:
ffmpeg -i path/to/input_video.mp4 -ss mm:ss -to mm2:ss2 -codec copy path/to/output_video.mp4
Motivation:
Trimming video files is useful for creating highlights, removing unwanted sections, or shortening files for specific use. It’s especially beneficial for creating teasers from full-length videos or ensuring content fits within platform time limits.
Explanation:
-i path/to/input_video.mp4
: Specifies which video file is to be trimmed.-ss mm:ss
: Indicates the start time for trimming, enabling the extraction of content from a specific point in the video.-to mm2:ss2
: Marks the endpoint for the trim, defining how much of the video should be retained in the cut.-codec copy
: Ensures that the video and audio streams are copied directly without re-encoding, preserving quality and speeding up processing.path/to/output_video.mp4
: Names the resultant video file where the trimmed content will be saved.
Example output:
A video file containing only the segment between the defined start and end times, extracted from the source video.
Use case 6: Convert AVI video to MP4, with AAC Audio @ 128kbit and h264 Video @ CRF 23
Code:
ffmpeg -i path/to/input_video.avi -codec:a aac -b:a 128k -codec:v libx264 -crf 23 path/to/output_video.mp4
Motivation:
Converting AVI files, which are less compatible across modern devices, to MP4 format ensures greater accessibility and utility. Adjusting audio and video codecs optimizes file size and quality, especially for web or personal use.
Explanation:
-i path/to/input_video.avi
: Specifies the input file in AVI format for conversion.-codec:a aac
: Transcodes the audio to AAC, a versatile audio codec noted for its quality and compression efficiency.-b:a 128k
: Sets the audio bitrate to 128 kbps, balancing quality and size.-codec:v libx264
: Uses the H.264 codec for video, a widely-supported format offering high compression without sacrificing quality.-crf 23
: Dictates the video quality setting; a default or medium quality setting providing a solid balance between visual fidelity and file size.path/to/output_video.mp4
: Outputs the converted file in MP4 format.
Example output:
A smaller yet high-quality MP4 file converted from an original AVI format, with improved compatibility and optimized media settings.
Use case 7: Remux MKV video to MP4 without re-encoding audio or video streams
Code:
ffmpeg -i path/to/input_video.mkv -codec copy path/to/output_video.mp4
Motivation:
Remuxing is crucial for changing the container format without altering the codec, maintaining original quality while enhancing playback compatibility. This is helpful when dealing with network policies or device-specific format constraints that necessitate MP4 files.
Explanation:
-i path/to/input_video.mkv
: Recognizes the MKV file needing conversion into another container format.-codec copy
: Copies the audio and video streams exactly as they are found in the input, bypassing the need for time-intensive re-encoding processes.path/to/output_video.mp4
: The target container format and filename, which increases the range of devices capable of playback.
Example output:
An MP4 file with the same audio and video streams as the MKV input, allowing for immediate playback without compromising quality.
Use case 8: Convert MP4 video to VP9 codec, using a CRF value and bitrate
Code:
ffmpeg -i path/to/input_video.mp4 -codec:v libvpx-vp9 -crf 30 -b:v 0 -codec:a libopus -vbr on -threads number_of_threads path/to/output_video.webm
Motivation:
This conversion is particularly advantageous for compatibility with web-based streaming platforms that prefer the VP9 codec for its efficient compression and quality retention. VP9 reduces size without high-quality loss, making it perfect for online video sharing.
Explanation:
-i path/to/input_video.mp4
: Sets the input file in MP4 format for conversion to VP9.-codec:v libvpx-vp9
: Specifies the use of the VP9 codec for video, optimal for web streaming due to improved compression.-crf 30
: Establishes the quality level. CRF 30 offers a decent quality-to-size ratio, useful for many situations.-b:v 0
: Signals to use constant quality instead of a fixed bitrate, emphasizing quality over exact file size.-codec:a libopus
: Chooses Opus for the audio codec, known for its superior performance at low bitrates.-vbr on
: Enables variable bitrate for audio, ensuring dynamic adjustment of bitrate for better audio quality.-threads number_of_threads
: Holdups parallel processing through specified threads, allocating computing resources for faster conversion.path/to/output_video.webm
: Indicates the output filename and introduces the WEBM format, combining VP9 and Opus for efficient web streaming.
Example output:
A WEBM file with a VP9 video stream and Opus audio, optimized for size and compatible with web-based applications or content delivery networks.
Conclusion:
These use cases demonstrate the versatile capabilities of FFmpeg in processing a wide variety of media tasks. By managing different formats and settings, FFmpeg serves as an indispensable tool in the toolkit of anyone involved in multimedia production, distribution, or consumption. From simple tasks like audio extraction to complex format conversions, FFmpeg efficiently handles numerous scenarios, optimizing media files for playback, storage, and sharing across diverse platforms.