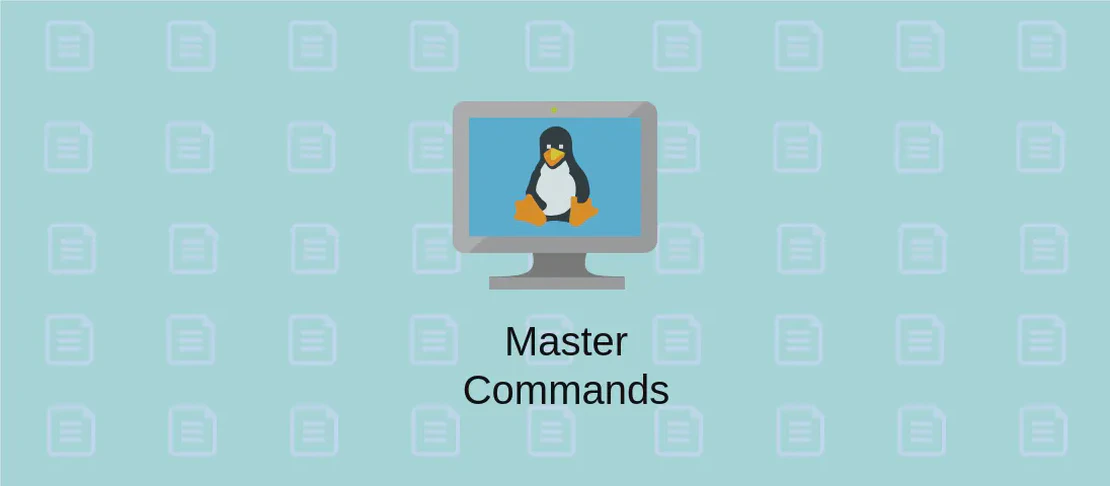
Understanding 'filecoordinationd' for macOS File Management (with examples)
- Osx
- December 17, 2024
filecoordinationd
is an essential daemon in macOS systems, designed to facilitate coordinated access to files across multiple processes. This is managed by leveraging the NSFileCoordinator
and NSFilePresenter
classes, ensuring that different applications or subprocesses can read from or write to a file without causing data corruption or conflicts. While this process is critical for maintaining file integrity in a multi-user or multi-process environment, it is not meant to be manually invoked by users.
Use case 1: Automatic Coordination by Daemon
Code:
# filecoordinationd
Motivation:
The automatic running of filecoordinationd
enhances the seamless operation of applications that require simultaneous access to shared files. For instance, consider a scenario where a document is edited in one application while being backed up by another. Without proper coordination, these simultaneous file operations could cause data loss or corruption. The daemon effortlessly manages these concurrent requests, ensuring data consistency and reliability across applications.
Explanation:
filecoordinationd
: This is the main process that operates in the background on macOS platforms. It is not to be manually initiated but is instead automatically activated by the system whenever needed. In practice, developers interact withNSFileCoordinator
andNSFilePresenter
interfaces to integrate file coordination into their applications, which in turn utilizefilecoordinationd
.
Example Output:
Since filecoordinationd
is a background process, there will be no direct user-facing output. Its presence is typically observed through its effects—smooth operation and improved file handling by macOS applications that rely on file coordination.
Use case 2: Developer Integration with NSFileCoordinator
Code:
NSFileCoordinator *coordinator = [[NSFileCoordinator alloc] initWithFilePresenter:nil];
[coordinator coordinateReadingItemAtURL:fileURL options:0 error:NULL byAccessor:^(NSURL *newURL) {
NSData *data = [NSData dataWithContentsOfURL:newURL];
// Process the data
}];
Motivation:
Developers often need to implement file coordination in their applications to manage file reading and writing, thus safeguarding against data corruption and access conflicts. By integrating NSFileCoordinator
, developers ensure that their application’s file access remains reliable, even when multiple applications are simultaneously accessing or modifying the same files.
Explanation:
NSFileCoordinator
: This class is initialized to manage read or write access to specific file URLs, ensuring that other processes are coordinated properly.coordinateReadingItemAtURL
: This method facilitates the reading of a file at a specified URL. ThebyAccessor
block allows the developer to define how the file’s content is processed after being accessed without any coordination conflict.NSURL *newURL
: Acts as the synchronized file URL, after coordination is applied.
Example Output:
Like the daemon itself, this form of file coordination doesn’t render visual output. However, successful implementation will be evident through applications smoothly accessing files without conflicts, and without the user experiencing any glitches or data corruption.
Conclusion:
The filecoordinationd
daemon plays an irreplaceable role in the macOS environment, allowing multiple processes to access files seamlessly and without conflict. Although users never directly interact with it, its operation is critical to maintaining the integrity and reliability of macOS applications. By using system-provided classes like NSFileCoordinator
, developers ensure their applications harmonize well with the broader ecosystem, ultimately providing a stable and reliable user experience.