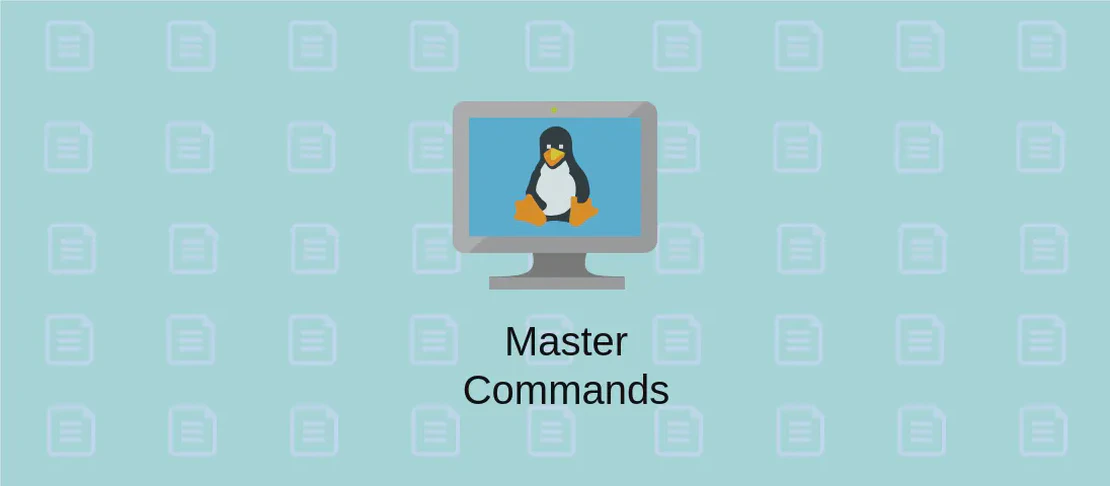
How to Use the Command 'find' (with examples)
The find
command is a powerful and versatile tool used in Unix and Unix-like operating systems to search for files and directories within a directory hierarchy. It searches through a directory tree, recursively, to locate files or directories based on various criteria such as name patterns, file types, sizes, modification dates, and more. This flexibility makes find
indispensable for anyone looking to efficiently manage files on their system.
Use case 1: Find files by extension
Code:
find root_path -name '*.ext'
Motivation:
You may often need to locate all files of a certain type across directories to perform subsequent operations, analyze data, or manage resources. For instance, finding all “.txt” files within a project directory enables you to quickly access or process text-based data without manually searching through multiple subfolders.
Explanation:
root_path
: The starting directory path where the search begins.-name '*.ext'
: This argument specifies that the command looks for files ending with the “.ext” extension. The asterisk ‘*’ is a wildcard that matches any string of characters.
Example output:
/path/to/directory/file1.ext
/path/to/anotherdirectory/file2.ext
Use case 2: Find files matching multiple path/name patterns
Code:
find root_path -path '**/path/**/*.ext' -or -name '*pattern*'
Motivation:
Sometimes, the criteria for finding files are not straightforward and might involve searches through specific paths and by name patterns concurrently. For example, you might want to find all “.log” files stored in “backup” directories or files containing a specific keyword across various directories.
Explanation:
root_path
: Path to start the search.-path '**/path/**/*.ext'
: This tellsfind
to match files with paths that have “path” in them and end with “.ext”.-or
: Logical OR operator combining search conditions.-name '*pattern*'
: Searches for files that have a name containing the string “pattern”.
Example output:
/path/to/match/path/morepath/file.ext
/path/to/pattern/filepattern.ext
Use case 3: Find directories matching a given name, in case-insensitive mode
Code:
find root_path -type d -iname '*lib*'
Motivation:
Locating specific directories by name, especially when ignoring case sensitivity, is useful in environments where directory naming conventions might vary. This is beneficial when organizing directories that might occasionally deviate from standard naming conventions or during migration processes where directory names are not consistently capitalized.
Explanation:
root_path
: Directory to start searching from.-type d
: Filters the search to directories only.-iname '*lib*'
: Searches for directories with names containing “lib”, ignoring case sensitivity.
Example output:
/usr/local/lib/
/home/user/Library/
Use case 4: Find files matching a given pattern, excluding specific paths
Code:
find root_path -name '*.py' -not -path '*/site-packages/*'
Motivation:
When dealing with large codebases or Python projects, it’s often necessary to locate Python files (*.py) while excluding specific directories such as “site-packages”, which typically holds third-party dependencies. This exclusion is crucial when you want to focus on your code rather than external libraries.
Explanation:
root_path
: Starting directory for the search.-name '*.py'
: Looks for Python files.-not
: Negates the search condition that follows.-path '*/site-packages/*'
: Specifies the path pattern to exclude from the search.
Example output:
/myproject/src/module1.py
/myproject/tests/test_suite.py
Use case 5: Find files matching a given size range, limiting the recursive depth to “1”
Code:
find root_path -maxdepth 1 -size +500k -size -10M
Motivation:
Disk space management is a crucial aspect of system administration. You might often need to find and possibly manage files within a specific size range to free up space or analyze storage usage. Limiting the search depth can quickly give insights into large files located directly within a given directory.
Explanation:
root_path
: Directory to start the search.-maxdepth 1
: Restricts the search to files directly within root_path, not going deeper into subdirectories.-size +500k
: Matches files larger than 500 kilobytes.-size -10M
: Matches files smaller than 10 megabytes.
Example output:
/path/to/directory/largefile.dat
/path/to/directory/report.docx
Use case 6: Run a command for each file (use {}
within the command to access the filename)
Code:
find root_path -name '*.ext' -exec wc -l {} \;
Motivation:
Automating actions on files found via search criteria streamlines many bulk processing tasks. For example, you might want to count the number of lines in all files with a particular extension to analyze content size or complexity and keep the process efficient through in-line execution commands.
Explanation:
root_path
: Path where the search begins.-name '*.ext'
: Finds files with the “.ext” extension.-exec
: Allows execution of a command on each found file.wc -l {}
: Runs the ‘word count’ command with the ‘-l’ option to count lines, using{}
to access each filename.\;
: Indicates the end of the command to execute for each file.
Example output:
47 /path/to/directory/file1.ext
53 /path/to/directory/file2.ext
Use case 7: Find all files modified today and pass the results to a single command as arguments
Code:
find root_path -daystart -mtime -1 -exec tar -cvf archive.tar {} \+
Motivation:
When managing system logs or incremental data backups, identifying files modified on a specific day and processing them collectively, like archiving, facilitates efficient daily logistics and snapshot creation without redundant manual efforts.
Explanation:
root_path
: Directory at which the search is initiated.-daystart
: Specifies the start of a day for use in calculating times.-mtime -1
: Matches files modified in the last 24 hours.-exec tar -cvf archive.tar {}
: Creates a tar archive of the files. The{}
passes all found files as arguments totar
.\+
: Signals that all results should be combined into a single argument list for the command.
Example output:
a file1.ext
a file2.ext
Use case 8: Find empty files (0 byte) or directories and delete them verbosely
Code:
find root_path -type f|d -empty -delete -print
Motivation:
Removing empty files and directories can help in organizing and optimizing storage, particularly in development environments or content repositories where redundant or in-progress artifacts get generated. Cleaning these enables smoother maintenance routines and increases operational efficiency.
Explanation:
root_path
: Base directory to start searching.-type f|d
: Checks both files and directories.-empty
: Looks for files or directories with no content (0 bytes or no entries).-delete
: Deletes the found empty items.-print
: Prints the names of deleted files and directories.
Example output:
/path/to/emptyfile.txt
/path/to/emptysubdirectory/
Conclusion
The find
command provides a comprehensive toolkit for searching and managing files and directories based on diverse criteria. Whether locating specific files, managing file sizes, handling modified content, or automating operations, each use case outlined leverages find
’s powerful features for efficient system management and data handling. With practice, users can master these capabilities to optimize workflows and improve productivity on Unix-like systems.