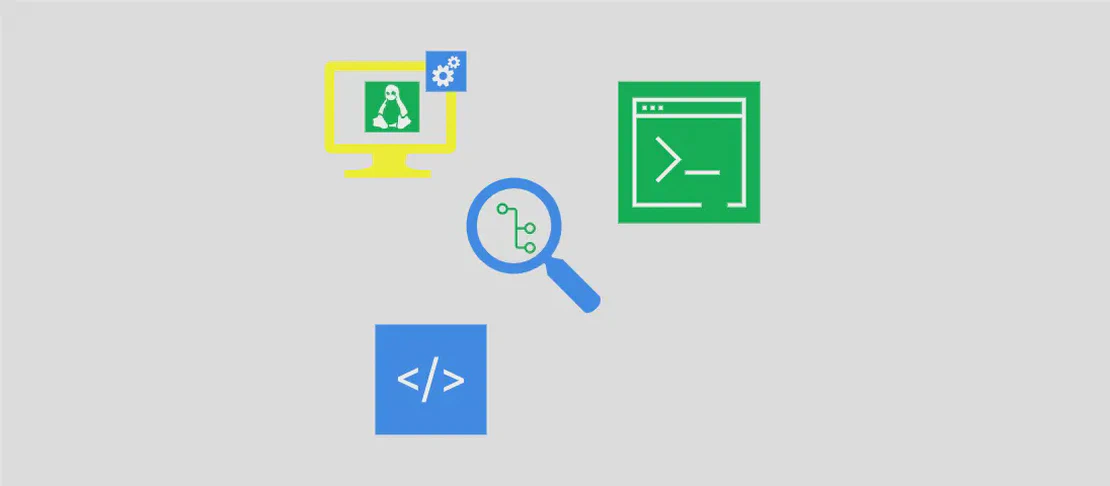
How to Use the 'findstr' Command (with examples)
- Windows
- December 17, 2024
The findstr
command in Windows is a versatile tool used for searching specific strings or patterns within files or command outputs. It offers powerful options for text searching, including case insensitivity, use of regular expressions, and recursive searches through directories. This command can be used by developers, system administrators, and data analysts to efficiently locate and filter data from large sets of files or outputs.
Use case 1: Find one or more strings in all files
Code:
findstr "string1 string2 ..." *
Motivation:
This use case is essential when you need to search multiple strings across all files in a directory. For instance, if you’re examining log files or codebases to find specific error messages or function calls, this command provides a simple, quick solution.
Explanation:
findstr
: The command used to start the search."string1 string2 ..."
: Represents the strings you are searching for. This input can include multiple strings separated by spaces.*
: Indicates that the search should be performed in all files within the current directory.
Example Output:
Suppose you’re searching for the strings “error” and “warning” in a directory of log files. The output could be:
errorlog.txt:13:This is an error message.
warninglog.txt:27:A warning was triggered.
Use case 2: Find one or more strings in a piped command’s output
Code:
dir | findstr "string1 string2 ..."
Motivation:
When you need to filter output from other commands, such as dir
, which lists directory contents, this use case is helpful. It allows you to focus on files or directories that match your criteria without manually sifting through all output.
Explanation:
dir
: Command to list directory contents.|
: Used to pipe the output of thedir
command tofindstr
.findstr
: Command to match specified strings."string1 string2 ..."
: Strings being searched for within the piped output.
Example Output:
If you’re searching for files containing “report” and “summary” in the directory list, you might see:
04/07/2023 11:07 AM 123,456 report_2023.txt
01/15/2023 02:30 PM 11,235 summary.docx
Use case 3: Find one or more strings in all files recursively
Code:
findstr /s "string1 string2 ..." *
Motivation:
For projects with nested directories, a recursive search is necessary to ensure you don’t miss any files. This is especially useful in large projects where relevant information might be deeply nested in subfolders.
Explanation:
/s
: This flag ensures that the search is done recursively in all subdirectories.*
: A wildcard indicating all files in the specified directory tree.
Example Output:
When searching for “include” and “define” in a codebase:
src\main.c:49:#include "header.h"
include\config.h:15:#define VERSION 2.0
Use case 4: Find strings using a case-insensitive search
Code:
findstr /i "string1 string2 ..." *
Motivation:
Case-insensitive searches are crucial when you’re uncertain about the capitalization used in the files. This use case is beneficial when searching for user-generated data or language-specific keywords where capitalization might vary.
Explanation:
/i
: The option that switches the search to be case-insensitive, so both “string” and “STRING” will match.*
: To conduct the search in all files.
Example Output:
When looking for “admin” and “user” without case sensitivity:
UserData.txt:3:Admin rights granted.
logs.txt:22:user logged in.
Use case 5: Find strings in all files using regular expressions
Code:
findstr /r "expression" *
Motivation:
Regular expression searches are powerful for pattern matching, allowing complex search criteria. This is invaluable in data parsing, processing, or scripting where you need to find patterns, like date formats or email addresses.
Explanation:
/r
: Indicates the use of a regular expression."expression"
: The regular expression pattern to search for. This further allows for complex search terms.*
: A wildcard to search in all files.
Example Output:
When using a regex like \d{4}-\d{2}-\d{2}
to find date formats:
records.txt:5:Date of Birth: 1990-01-22
orders.csv:17:Order Date: 2023-04-11
Use case 6: Find a literal string (containing spaces) in all text files
Code:
findstr /c:"string1 string2 ..." *.txt
Motivation:
Literal string searches, including spaces, are helpful for phrases or multi-word identifiers. This is particularly useful in documentation files where you might be looking for specific sections or headings.
Explanation:
/c:"string1 string2 ..."
: Encapsulates the search string in quotes to handle spaces as literal characters.*.txt
: Limits the search to text files only.
Example Output:
Searching for the phrase “class declaration” in text files might result in:
notes.txt:41:Examples of a class declaration include...
Use case 7: Display the line number before each matching line
Code:
findstr /n "string1 string2 ..." *
Motivation:
Including line numbers is beneficial for code reviews or debugging, allowing you to quickly locate where issues or matching patterns occur in the files.
Explanation:
/n
: Prefixes each matching line with its line number in the file."string1 string2 ..."
: Strings to search for.*
: To search across all files.
Example Output:
For the strings “function” and “return”:
main.py:102:def function_example():
utils.py:45: return value
Use case 8: Display only the filenames that contain a match
Code:
findstr /m "string1 string2 ..." *
Motivation:
When you only need to know which files contain specific matches (rather than viewing every matching line), this option provides quick file-level insight without cluttering your output.
Explanation:
/m
: Restricts the output to filenames only, hiding individual matching lines."string1 string2 ..."
: The strings being searched for.*
: Wildcard indicating to search within all files.
Example Output:
Searching for “build” and “configure”:
build_log.txt
configuration.yaml
Conclusion:
The findstr
command in Windows provides extensive options for text searching and filtering, making it an indispensable tool for many computer users, particularly those dealing with large datasets or complex codebases. By harnessing its various use cases and options, users can efficiently locate and analyze text data for a vast array of needs and scenarios.