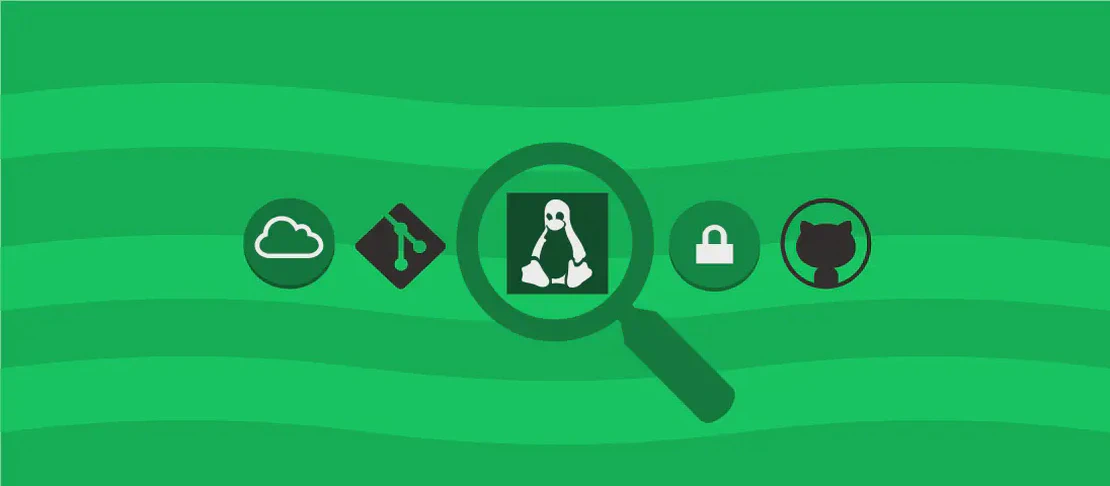
Mastering Firebase CLI Commands (with examples)
Firebase is a robust platform provided by Google that lets developers build high-quality apps quickly without managing infrastructure. It provides real-time databases, authentication services, hosting, and many other features. The Firebase CLI is a powerful command-line tool that allows developers to interact with Firebase services, providing functionalities to test, manage, and deploy Firebase projects efficiently. This article explores several practical use cases of the Firebase CLI, demonstrating its capabilities and how it streamlines various development tasks.
Use case 1: Logging in to Firebase Console
Code:
firebase login
Motivation:
Before accessing Firebase’s powerful tools and deploying your applications, you need to authenticate your identity. This step is crucial because it ensures that only authorized users can modify or deploy projects, keeping your Firebase projects secure and manageable.
Explanation:
The firebase login
command has no additional arguments because it uses a standard authentication mechanism to connect your local development environment with your Firebase account. It opens your web browser, guides you through the Google authentication process, and stores your credentials locally for subsequent operations.
Example Output:
Visit this URL on this device to log in:
<URL>
Waiting for authorization...
✔ Success! Logged in as your-email@example.com
Use case 2: Listing Existing Firebase Projects
Code:
firebase projects:list
Motivation:
This command is useful when managing multiple Firebase projects, offering a quick overview of all projects under your account. It’s particularly beneficial for developers working on multiple applications, as it helps them to quickly identify project IDs and names, streamlining project selection for further operations.
Explanation:
The firebase projects:list
command does not require any additional arguments. When executed, it queries your Firebase account and fetches all associated projects, displaying them in a concise list format. This functionality is a definitive asset for maintaining order and accessibility across projects.
Example Output:
✔ Projects in your Firebase account
┌────────────┬───────────────┬──────────────────┐
│ Project ID │ Project Name │ Resources │
├────────────┼───────────────┼──────────────────┤
│ my-first │ My First App │ 1 function │
│ my-second │ Second App │ 3 functions │
└────────────┴───────────────┴──────────────────┘
Use case 3: Initializing a Firebase Project
Code:
firebase init
Motivation:
Initial project setup can be a daunting task, especially for new developers. The firebase init
command simplifies this process by guiding users through an interactive setup wizard. It helps in configuring Firebase services such as Firestore, Functions, and Hosting, making it accessible to developers of all skill levels.
Explanation:
Running the firebase init
command launches an interactive wizard where users can select specific Firebase services they want to include in the project. It assists in creating essential configuration files and sets up the selected services seamlessly within your current directory.
Example Output:
? Which Firebase features do you want to set up for this directory? (Press Space to select)
Firestore: Configure security rules and indexes files for Firestore
Functions: Configure a Node.js environment to write Cloud Functions
Hosting: Configure and deploy Firebase Hosting sites
...
Use case 4: Deploying Code and Assets
Code:
firebase deploy
Motivation:
Deployment is a critical phase in the development lifecycle, allowing applications to be pushed from a development stage to live environments. The firebase deploy
command makes this process quick and efficient, ensuring that updated code and assets are smoothly transferred to your Firebase hosting environment.
Explanation:
This straightforward command deploys all configured services (like Hosting, Functions, Firestore rules) to your Firebase project. It reads the local configuration and code, then syncs it with the Firebase servers. This command does not require additional arguments for a general deployment but can be modified with flags to target specific services (not covered in this basic use case).
Example Output:
=== Deploying to 'my-first'...
i deploying hosting
Finished your deployment!
✔ Deploy complete!
Use case 5: Serving Locally
Code:
firebase serve
Motivation:
Developers often need to test their applications locally before deploying them. The firebase serve
command enables developers to run a local server, simulating deployment settings. This feature allows confident integration testing and debugging in a local setup, closely mimicking the live environment.
Explanation:
Executing firebase serve
starts a local server to host your project’s assets using the configurations specified in your firebase.json
file. No additional arguments are necessary, as the command fetches the pre-configured settings, providing an accurate local representation of your project’s environment.
Example Output:
✔ hosting: Local server: http://localhost:5000
Use case 6: Opening Project Links
Code:
firebase open
Motivation:
Quick access to various URLs associated with a Firebase project enhances efficiency, especially when switching between multiple Firebase services or project environments. This command offers a streamlined approach to open project-specific links directly from your command line, facilitating swift navigation within Firebase tools.
Explanation:
The firebase open
command starts an interactive wizard that presents various options, such as opening the Firebase Console, Authentication page, Database, Hosting, and more, directly in a web browser. This interactive capability eliminates the need to manually copy or remember URLs, significantly enhancing workflow efficiency.
Example Output:
Issues in your project:
- Firebase Console
- Authentication Dashboard
- Firestore Database
? Which project link would you like to open?
...
Conclusion:
The Firebase CLI provides powerful, streamlined tools crucial for modern web application development. Each command is designed to simplify a specific aspect of managing Firebase projects, from authentication to project listing, setup, deployment, local testing, and accessing online resources. By mastering these commands, developers can significantly enhance their productivity and ensure seamless project execution. Firebase continues to be an essential staple for developers invested in creating impactful, robust applications.