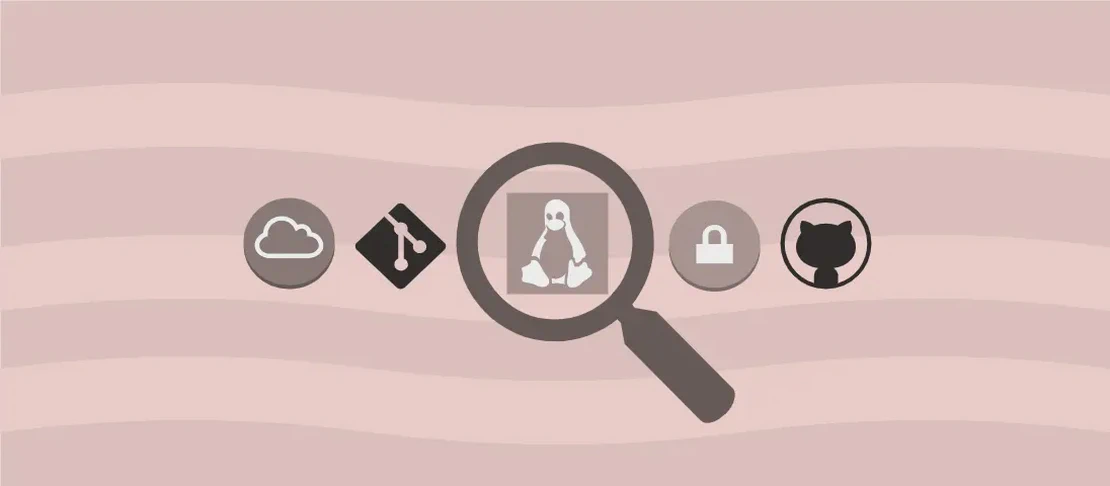
How to Use the Command 'flake8' (with Examples)
Flake8 is a powerful command-line utility used for checking the style and quality of Python code. It combines the functionalities of PyFlakes, pycodestyle, and McCabe script to provide Python developers with a comprehensive linting tool that checks for Python syntax errors, style guide enforcement, and code complexity. By using Flake8, developers can ensure their code is clean, readable, and adheres to PEP 8 standards, ultimately leading to high-quality software development.
Use case 1: Lint a File or Directory Recursively
Code:
flake8 path/to/file_or_directory
Motivation:
Performing lint checks on a single file or an entire directory is crucial in the initial stages of code development and review. This command helps ensure that all code adheres to the prescribed style guide, allowing developers to maintain consistency and readability. Running Flake8 recursively on a directory is particularly valuable as it ensures all files within the directory, regardless of depth, are checked, fostering a codebase with a uniform style.
Explanation:
flake8
: This is the command itself, invoking the Flake8 tool.path/to/file_or_directory
: This argument specifies the file or directory you want Flake8 to lint. Flake8 will check all files contained in the specified path for any style or quality violations.
Example Output:
path/to/file_or_directory/file1.py:10:1: F401 'os' imported but unused
path/to/file_or_directory/file2.py:22:5: E303 too many blank lines (3)
These results indicate specific lines where issues are detected, allowing developers to pinpoint and fix errors efficiently.
Use case 2: Lint a File or Directory Recursively and Show the Line on Which Each Error Occurred
Code:
flake8 --show-source path/to/file_or_directory
Motivation:
Understanding the context of a style or quality error can significantly aid in troubleshooting and resolving issues. By displaying the line of code where the error occurred, developers can quickly see what might be wrong, which improves the feedback loop during code review or development. This feature is especially useful for new developers, as it provides insight into what constitutes a style or syntax violation.
Explanation:
flake8
: Initiates the Flake8 tool.--show-source
: This option requests Flake8 to output the exact source code line where the error appears, giving context to the violation.path/to/file_or_directory
: The target upon which Flake8 is running its checks.
Example Output:
path/to/file_or_directory/file1.py:10:1: F401 'os' imported but unused
import os
path/to/file_or_directory/file2.py:22:5: E303 too many blank lines (3)
def function_name():
These outputs include the offending line of code itself, aiding in understanding and correcting the specific issues.
Use case 3: Lint a File or Directory Recursively and Ignore a List of Rules
Code:
flake8 --ignore rule1,rule2 path/to/file_or_directory
Motivation:
Sometimes, specific rules may not be applicable or desirable in a particular project context due to legacy code, existing team standards, or conscious deviations from default guidelines. Using Flake8’s ignore functionality allows teams to bypass certain checks, streamlining the linting process and making it more relevant to their specific coding environment without overwhelming the developer with irrelevant warnings.
Explanation:
flake8
: Commands the Flake8 tool to start.--ignore rule1,rule2
: This tells Flake8 to overlook the specified rule codes (e.g., E501 for line length), fitting the linting process to custom needs.path/to/file_or_directory
: Represents the target file or directory for lint checks.
Example Output:
path/to/file_or_directory/file3.py:30:2: F403 'from module import *' used; unable to detect undefined names
In this output, only violations outside the ignored rules are reported, focusing the developer’s attention on specific issues.
Use case 4: Lint a File or Directory Recursively but Exclude Files Matching the Given Globs or Substrings
Code:
flake8 --exclude substring1,glob2 path/to/file_or_directory
Motivation:
In robust codebases, some files may not need linting due to their temporary nature (like auto-generated files) or because they belong to third-party libraries. Excluding these files helps focus on the more critical sections of the codebase that require consistent style enforcement, thus optimizing the developer’s efforts during code checks.
Explanation:
flake8
: Executes the linting with Flake8.--exclude substring1,glob2
: This option helps to omit files that match certain patterns, based on either globs or substrings, from the linting process.path/to/file_or_directory
: The directory or file being analyzed.
Example Output:
path/to/file_or_directory/source.py:42:1: W391 blank line at end of file
Only files that aren’t excluded are considered in the linting process, thus reducing noise from the linting output.
Conclusion:
Using Flake8 with its versatile command options helps enhance the quality and style consistency of Python codebases. Whether you need to lint entire directories, focus on specific rules, or exclude irrelevant segments of your project, Flake8 offers a range of configurations to fit different developer needs. By carefully selecting command options, developers can maintain cleaner and more maintainable codebases.