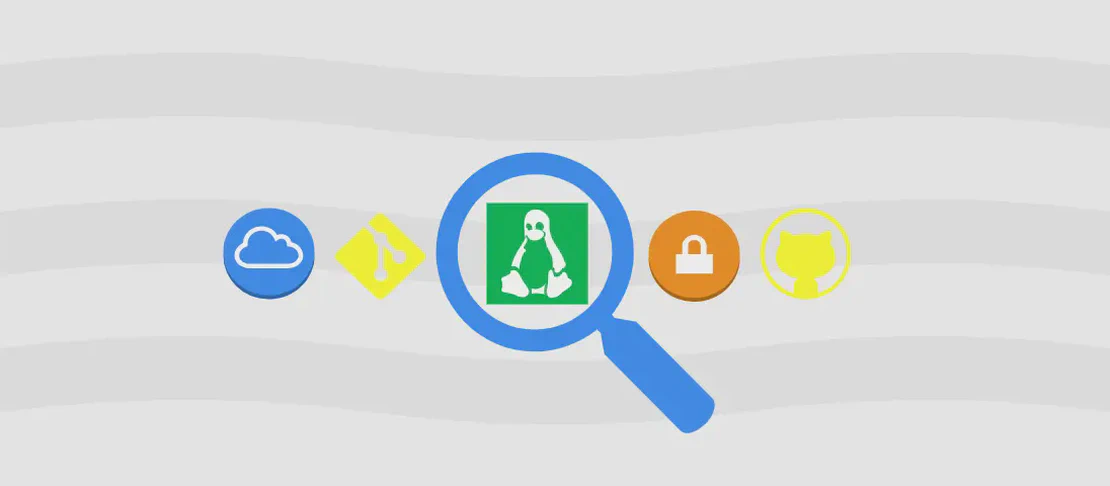
How to use the command 'flask' (with examples)
Flask is a lightweight web framework written in Python, providing developers with essential tools and features to build robust web applications. Its command-line interface (CLI) includes a general utility script allowing for various tasks, such as running development servers, initializing a Python shell, and managing database operations. The primary setup for Flask applications hinges on defining the app in the FLASK_APP
environment variable, enabling the command to perform operations within the application context. This versatility makes Flask a popular choice for both beginner and experienced web developers.
Use case 1: Run a development server
Code:
flask run
Motivation for using this example:
Running a development server is one of the most common tasks when working with Flask applications. This use case is particularly beneficial during the development phase because it allows developers to see real-time updates and interactions with the application they are building. By using the flask run
command, developers can efficiently test changes, debug issues, and ensure that the application behaves as expected in a controlled environment. The development server also provides a simple setup without requiring complex server configurations, thus facilitating a smooth development workflow.
Explanation for every argument given in the command:
flask
: This initiates the Flask command-line interface, which is the entry point for all Flask-related commands. The CLI provides various subcommands that can interact with the Flask application.run
: This specific subcommand starts a built-in development server that hosts the Flask application. The server is designed for development purposes, offering features like automatic reloading and a debugger.
Example output:
Running the flask run
command will output something like the following:
* Serving Flask app 'my_app.py'
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
This output confirms that the development server is up and running, pointing to the local machine’s IP address (127.0.0.1
) and the port number (5000
). It also provides information on how to terminate the server.
Use case 2: Show the routes for the app
Code:
flask routes
Motivation for using this example:
Understanding the available routes in a Flask application is crucial for developers as it outlines the various endpoints users can interact with. This command is especially helpful for gaining insights into the application’s structure, verifying expected routes, and debugging routing issues. Additionally, when collaborating in teams, having access to the complete list of routes aids in ensuring that different application modules can communicate effectively without conflicts. The flask routes
command quickly displays all registered URLs alongside the corresponding methods and endpoint functions, enhancing the visibility of the application map.
Explanation for every argument given in the command:
flask
: As before, this starts the Flask command-line interface, providing access to a suite of commands for managing the application.routes
: This subcommand generates a comprehensive list of all the routes defined in the Flask application, including their methods and endpoint functions. This gives a structural overview of the URLs the application can handle.
Example output:
The output of the flask routes
command will resemble the following:
Endpoint Methods Rule
-------- ------- ----------------------
index GET /
about GET /about
contact GET,POST /contact
This tabular format indicates the endpoints (such as index
, about
, and contact
), the HTTP methods allowed (e.g., GET
, POST
), and the URL rules corresponding to each endpoint.
Use case 3: Run a Python interactive shell in the app’s context
Code:
flask shell
Motivation for using this example:
Opening a Python shell within the Flask app’s context is a powerful tool for developers. This feature is particularly useful for testing functions, interacting with the application’s database, and exploring objects and data without needing to run the server. It enables developers to quickly prototype, debug, and experiment directly with the application code. By having access to the app’s context in a shell, developers can directly import modules, manipulate data, and observe the immediate effects of their commands in a controlled setting, fostering a more dynamic and interactive debugging experience.
Explanation for every argument given in the command:
flask
: Initiates the command-line utility provided by Flask, designed to perform various tasks within the application’s environment.shell
: This subcommand launches an interactive Python shell (commonly IPython if available) with the application’s context preloaded. This means developers can work directly with the app’s modules and entities without additional imports.
Example output:
Upon executing the flask shell
command, you might see output similar to this:
Python 3.x.x (default, Jul 8 2022, 18:18:08)
Type 'help', 'copyright', 'credits' or 'license' for more information.
(InteractiveConsole)
>>>
At this prompt, developers can execute Python code with the application’s context ready to use, providing a sandbox for testing and exploration.
Conclusion:
The Flask command-line interface is a versatile tool packed with essential commands that streamline the development and management of web applications. By utilizing commands like flask run
, flask routes
, and flask shell
, developers can efficiently run a development server, explore available application routes, and interact with the application in a shell environment. These use cases showcase the flexibility and convenience Flask offers, empowering developers to create, debug, and manage applications with ease.