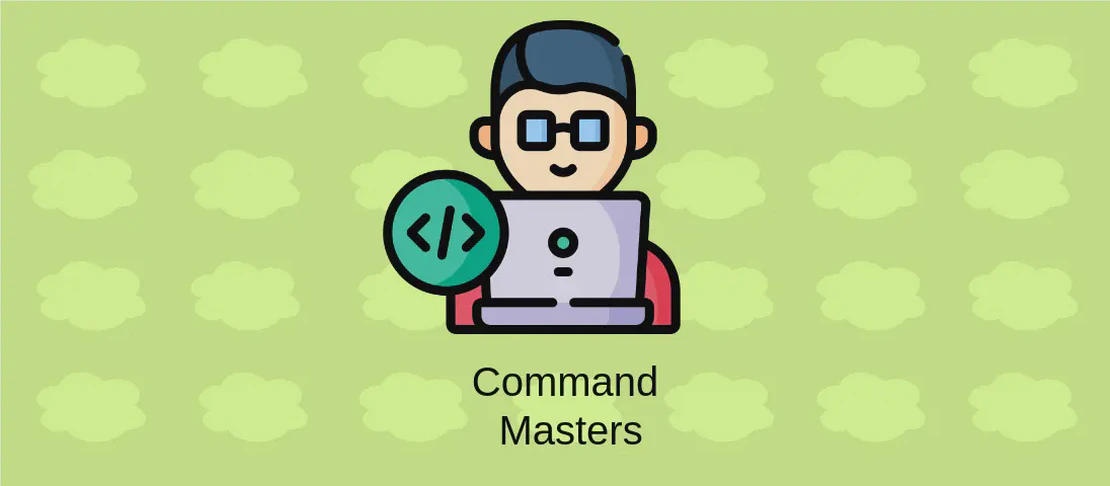
An In-Depth Guide to Using Flex (with examples)
Flex, or Fast Lexical Analyzer Generator, is a powerful tool for generating lexical analyzers. It is a re-imagined version of the lex program, incorporating additional features while staying true to the POSIX specification. By taking a set of rules defined in a specification file, it outputs C code that implements these rules, effectively automating the process of building lexical analyzers. Note that on OpenBSD, long options aren’t supported.
Use case 1: Generate an analyzer from a flex file, storing it to the file lex.yy.c
Code:
lex analyzer.l
Motivation:
This is often the first step in using flex. Developers typically have a .l
file, which contains the rules for recognizing lexical patterns in source text. After creating this file, the next logical step is to convert those rules into a C source file that flex can use as input to produce an executable matching those rules.
Explanation:
lex
: Invokes the flex tool.analyzer.l
: Represents the input file containing the lexical specifications. This file is a user-defined file and typically ends in.l
, indicating it is a flex file.
Example Output:
The command will produce a default output file named lex.yy.c
containing the C code implementing the specified lexical rules.
Use case 2: Write analyzer to stdout
Code:
lex --stdout analyzer.l
Motivation:
Sometimes, developers want to immediately view the generated C code without saving it directly into a file. Redirecting the output to stdout
allows the developer to review the code in their terminal, providing a quick insight into the generated code.
Explanation:
lex
: Initiates the flex program.--stdout
: Instructs flex to write the generated C code to the standard output (stdout) rather than a default file likelex.yy.c
.analyzer.l
: The source file containing the lex rules to be translated into C.
Example Output:
The terminal window displays the generated C code directly, so you can inspect the logic flex derived based on the rules inside the analyzer.l
file.
Use case 3: Specify the output file
Code:
lex analyzer.l -o analyzer.c
Motivation:
Developers may prefer to control the naming and location of the output file. Instead of allowing flex to default to lex.yy.c
, specifying an output filename can improve file management and project organization.
Explanation:
lex
: Calls the flex program to action.analyzer.l
: The input file defining lexical rules.-o analyzer.c
: The-o
option allows specifying a custom file name for the output, in this case,analyzer.c
.
Example Output:
The generated C code is stored in a file named analyzer.c
, making it easier to integrate into projects that may have multiple such files or require specific naming conventions.
Use case 4: Generate a [B]atch scanner instead of an interactive scanner
Code:
lex -B analyzer.l
Motivation:
By default, flex creates a scanner that assumes interaction with stdin
(an interactive mode). However, in scenarios where batch processing of data is more appropriate—such as processing files non-interactively—the batch scanner becomes essential as it does not rely on stdin
.
Explanation:
lex
: Executes the flex program.-B
: Stands for “batch” scanner, which does not utilize standard input but instead, processes data without interactive prompts.analyzer.l
: The lex file specifying the rules to be compiled into the C code.
Example Output:
The output generated is a C source file configured to handle batch processing, differing in implementation to operate independently of stdin
.
Use case 5: Compile a C file generated by Lex
Code:
cc path/to/lex.yy.c --output executable
Motivation:
Once you have a C file generated by flex, the next natural step involves turning this C source into an executable. Compiling finalizes the creation process, enabling the analyzer to be run against other data sources effectively.
Explanation:
cc
: The C compiler command used to compile C files into executables.path/to/lex.yy.c
: Specifies the path to your C source file generated by flex.--output executable
: Directs the compiler to create an executable file, naming itexecutable
.
Example Output:
A new executable file is produced, referred to as executable
. This file can now be run to lexically analyze data according to the rules specified in your original flex file.
Conclusion:
Flex is a versatile tool for creating lexical analyzers, adaptable for various programming workflows and environments. These examples illustrate its core functionalities and some extended features, showing how it fits efficiently into the software development lifecycle. By understanding the usage and options provided by flex, developers can streamline their application development process, particularly with regard to lexical analysis tasks.