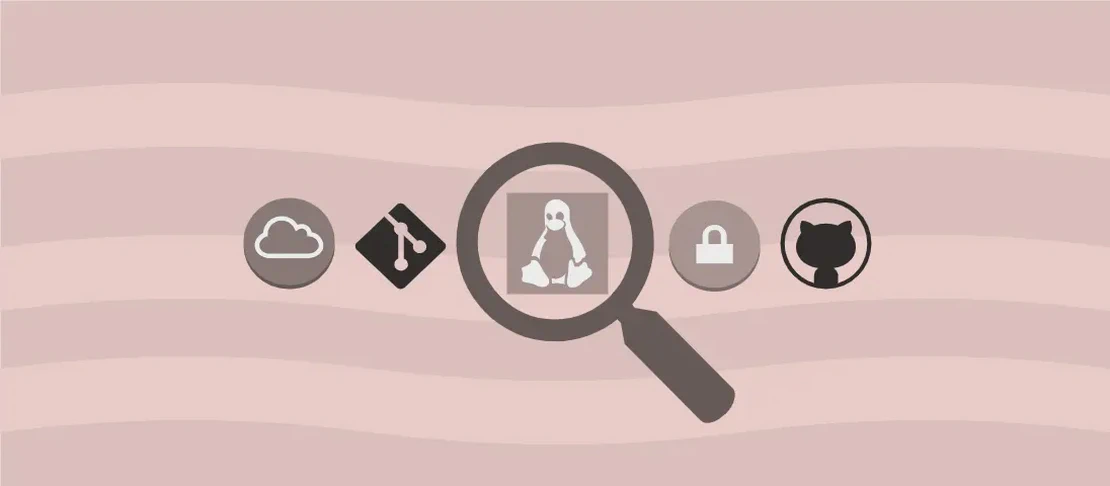
How to Use the Command 'flow' (with Examples)
Flow is a static type checker for JavaScript, created to help developers identify and avoid potential bugs by analyzing code. With Flow, developers can add type annotations to JavaScript code, enabling them to predict and prevent type-related errors. Offering compatibility with popular JavaScript tools and integrations, Flow aims to improve developer productivity and code reliability.
Use case 1: Run a Flow Check
Code:
flow
Motivation for using this example:
Running a Flow check is a critical step in the development lifecycle, especially when working in a large codebase with many contributors. It provides a quick and efficient means to catch type errors and inconsistencies across the codebase, preventing bugs from advancing into production. By regularly performing Flow checks, teams can maintain high code quality and assure that all type assumptions hold true.
Explanation:
- This command invokes the Flow server to actively analyze your JavaScript files against the specified types. When running
flow
, it performs real-time checks for any type mismatches or errors and reports them directly to the developer. This basic command serves as the starting point for initiating Flow’s powerful type-checking capabilities.
Example output:
Error ┈── path/to/file.js:8:14
Cannot assign 123 to `myString` because number [1] is incompatible with string [2].
path/to/file.js
:
8 ┌ let myString: string = 123;
│ ^ [1]
└───────────────────────── [2]
Found 1 error
Use case 2: Check Which Files are Being Checked by Flow
Code:
flow ls
Motivation for using this example:
When working with Flow, especially in a complex or modular project, it is critical to understand which files are included or excluded from type-checking. Knowing which files are being checked can help decide where to add new types, debug type errors, and ensure that all critical parts of the code are covered by Flow. This command helps ensure comprehensive type-checking coverage and can aid in troubleshooting inclusion issues.
Explanation:
- The
ls
subcommand lists the files currently under Flow’s purview. It provides a clear visual representation of which files are subject to type checking, allowing developers to easily identify potential gaps in coverage. Checking this list can reveal whether all necessary files are included or if certain files require their type annotations to be refined.
Example output:
path/to/file1.js
path/to/file2.js
path/to/utils/file3.js
Use case 3: Run a Type Coverage Check on All Files in a Directory
Code:
flow batch-coverage --show-all --strip-root path/to/directory
Motivation for using this example:
Conducting a type coverage check across all files in a specific directory gives developers insight into how extensively Flow types are being utilized. This is particularly valuable for projects in their initial phase of adopting Flow or for monitoring the progress as more files gain enhanced type coverage. This step highlights files that are under-typed or over-reliant on weak types, prompting developers to enhance their type definitions.
Explanation:
batch-coverage
analyzes multiple files and calculates their type coverage.--show-all
asks Flow to display coverage details for all files, regardless of whether the coverage is complete.--strip-root
removes the root directory from the reported paths, making the output more readable.path/to/directory
specifies the directory where the type coverage will be checked, providing an entry point for where the Flow analysis takes place.
Example output:
path/to/directory/file1.js: 75%
path/to/directory/file2.js: 60%
path/to/directory/subdirectory/file3.js: 90%
Overall type coverage: 75%
Use case 4: Display Line-by-Line Type Coverage Stats
Code:
flow coverage --color path/to/file.jsx
Motivation for using this example:
When optimizing code for better type safety, it’s incredibly efficient to view type coverage on a granular, line-by-line basis. This practice allows developers to pinpoint exactly where types are lacking and where the introduction of precise type annotations can have the most significant impact. This granular view invariably leads to a stronger understanding of code complexity and type usage possibilities.
Explanation:
coverage
computes and presents the type coverage for a specific file.--color
adds color-coding to the output, enhancing readability by easily distinguishing between typed and untyped code segments.path/to/file.jsx
denotes the specific file to be analyzed, targeting coverage statistics for precise files of interest.
Example output:
1: // @flow
2:
3: function add(a: number, b: number): number {
4: return a +
5: ^ number
6: b;
7: }
Conclusion:
Through its various commands, Flow provides JavaScript developers with a robust toolset for improving code quality and reducing bugs through type safety. Whether you’re running basic checks, understanding file inclusivity, auditing coverage throughout directories, or obtaining detailed line-by-line insights, Flow’s capabilities can form part of a solid foundation for any JavaScript project looking to enhance reliability and developer confidence.