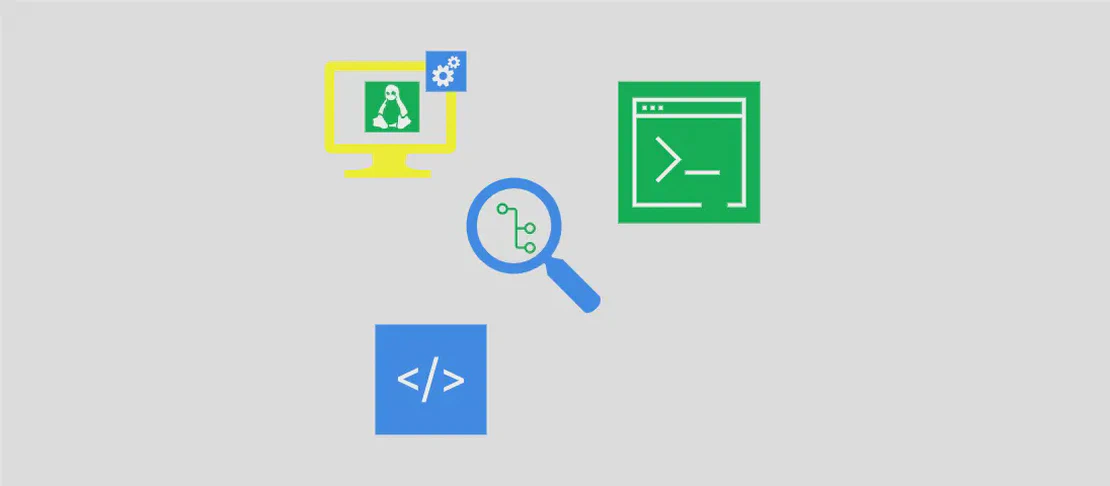
How to Use the Command 'flutter' (with examples)
Flutter is an innovative software development kit (SDK) created by Google, designed to build natively compiled applications for mobile, web, and desktop from a single codebase. It stands out due to its capability to deliver visually appealing interfaces using a fast, flexible, and interactive framework. As a cross-platform tool, it greatly simplifies the process of app development, as developers no longer need to maintain multiple codebases for different platforms like Android, iOS, and web.
Initialize a New Flutter Project in a Directory of the Same Name
Code:
flutter create project_name
Motivation:
When starting a new project in Flutter, this command is your entry point. It sets up the initial folder structure and files necessary for a standard Flutter application. Whether you are a beginner or an advanced developer, using this command saves time and avoids the possibility of errors that come with manually setting up a project.
Explanation:
flutter
: This denotes the invocation of the Flutter command-line tool.create
: This subcommand is used to initialize a new Flutter project.project_name
: This is the desired name for the project and the directory where all files will be generated.
Example Output:
Creating project project_name...
project_name/ios: Skipped
project_name/android: Created
...
Project project_name created. In the main.dart file, replace the contents...
Check if All External Tools Are Correctly Installed
Code:
flutter doctor
Motivation:
The flutter doctor
command is an essential tool for anyone setting up a Flutter development environment. It verifies the presence and versions of the various software dependencies required by Flutter and alerts the developer to any missing components, thereby ensuring a smooth development process.
Explanation:
flutter
: Again calling the Flutter tool.doctor
: This diagnostic tool checks for all required dependencies and installations used by Flutter, reporting any issues or incompatibilities.
Example Output:
[✓] Flutter (Channel stable, 3.0, on Mac OS X 10.15.7 19H2, locale en-US)
[✓] Android toolchain - develop for Android devices (Android SDK version 30.0.2)
[✗] Xcode - develop for iOS and macOS
✗ Xcode installation is incomplete; a full installation is necessary...
List or Change Flutter Channel
Code:
flutter channel stable|beta|dev|master
Motivation:
Flutter adopts a branching strategy known as channels which represent different release streams. Switching between these channels allows developers to prioritize stability (stable), features (beta), development (dev), or latest updates (master). This command is crucial for developers who need specific versions or stability for their projects.
Explanation:
flutter
: Launches the Flutter tool.channel
: This subcommand handles the selection or listing of channels.stable|beta|dev|master
: These represent specific channels. ‘Stable’ suits production-ready apps, ‘beta’ offers newer features with more potential issues, ‘dev’ is for daily time-boxed snapshots, and ‘master’ reflects the latest code which might be unstable.
Example Output:
Flutter channels:
* master
dev
beta
stable
Run Flutter on All Started Emulators and Connected Devices
Code:
flutter run -d all
Motivation:
Developers often need to test applications across multiple devices simultaneously to ensure consistent behavior. The flutter run -d all
command facilitates this by deploying the application to all connected devices and running emulators, offering a comprehensive testing environment right through the command line.
Explanation:
flutter
: Initiates the Flutter tool.run
: This subcommand compiles the app and sends it to a chosen device or emulator.-d
: Specifies the device to run on.all
: A special tag that indicates the application should be run on all available and connected devices.
Example Output:
Launching lib/main.dart on iPhone 12 in debug mode...
Running Xcode build...
Xcode build done. 8.2s
Launching spinner...
Run Tests in a Terminal from the Root of the Project
Code:
flutter test test/example_test.dart
Motivation:
Automated testing is crucial to maintain code quality and ensure robustness in applications. This command facilitates command-line execution of test scripts, making it possible to quickly check the functionality and performance of specific parts of an application without needing an IDE or external testing tools.
Explanation:
flutter
: Starts the Flutter CLI tool.test
: This subcommand is dedicated to running test scripts in the Flutter environment.test/example_test.dart
: Refers to the specific test script file that is to be executed. The path can be adjusted as necessary.
Example Output:
Running "flutter pub get" in project_name... 0.5s
00:05 +1: All tests passed!
Build a Release APK Targeting Most Modern Smartphones
Code:
flutter build apk --target-platform android-arm,android-arm64
Motivation:
To distribute an application on Android, a developer must build the application in APK (Android Package) format. The specified target platforms ensure your app is compatible with the majority of modern Android devices, thus broadening your potential user base.
Explanation:
flutter
: Calls the Flutter command-line interface.build
: Directs the tool to begin the build process of the project.apk
: Specifies that an Android APK build is required.--target-platform
: This flag specifies the intended CPU architecture.android-arm,android-arm64
: These are the specific CPU architectures for 32-bit and 64-bit versions respectively, covering most modern Android devices.
Example Output:
Running Gradle task 'assembleRelease'... 75.0s
√ Built build/app/outputs/flutter-apk/app-release.apk (23.0MB).
Display Help about a Specific Command
Code:
flutter help command
Motivation:
In a rich and complex command-line interface like Flutter’s, understanding the detailed usage and parameters of specific commands can be challenging. The flutter help command
is a quick solution for developers seeking to clarify doubts or learn more about any particular Flutter subcommand or option.
Explanation:
flutter
: The overarching Flutter command framework.help
: This subcommand provides usage information for other commands.command
: Placeholder for the specific command you seek information about. Replace ‘command’ with the actual command you’re interested in.
Example Output:
Usage: flutter [<options>] <command> [<args>]
--verbose Noisy logging, including all shell commands...
Conclusion:
The Flutter command-line tool provides a broad range of functionalities that significantly ease the app development process across various platforms. These examples showcase the core utilities offered by Flutter, including project initialization, managing project dependencies and settings, testing, and building distributable applications. Each command comes equipped with options and flags that can be tailored to specific needs, catering to both novice and experienced developers. Understanding and utilizing these commands is essential for efficient and effective Flutter application development.