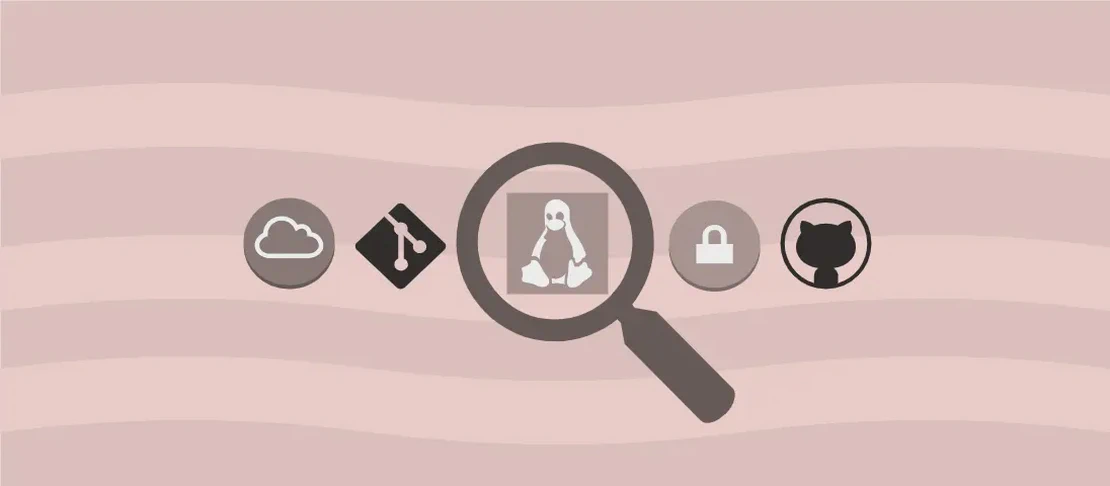
Understanding the Command 'flutter pub' (with examples)
Flutter’s package manager, known as flutter pub
, plays a crucial role in managing project dependencies. With flutter pub
, you can easily integrate packages into your Flutter project, ensuring you have access to a vast range of pre-built solutions available on pub.dev
. This tool simplifies the process of adding, removing, and updating packages, ultimately enhancing your development experience.
Use Case 1: Download/Update All Packages Specified in pubspec.yaml
Code:
flutter pub get
Motivation:
When working on a Flutter project, the pubspec.yaml
file is your best friend. It holds the list of all the dependencies your application requires—alongside their version constraints—to perform various tasks. These dependencies could include libraries for networking, animations, state management, and more. Running flutter pub get
is crucial because it resolves the necessary packages and downloads them to your project, ensuring everything is ready to go. This command sets the stage for a seamless development process by ensuring you have access to all the functionalities your widgets or APIs need.
Explanation:
flutter
: This keyword specifies that we are using Flutter’s command-line tools to run the command.pub
: This refers to Flutter’s package manager itself, which is responsible for handling the dependencies described inpubspec.yaml
.get
: This part of the command specifically tells the package manager to fetch and install all the dependencies listed in thepubspec.yaml
file. It effectively synchronizes your local project with the required packages.
Example Output:
When the command completes successfully, you’ll see an output reflecting that the dependencies were fetched and installed without issues. You might see something like this:
Running "flutter pub get" in your_project_name...
Resolving dependencies...
+ http 0.13.3 (0.14.0 available)
+ provider 6.0.0
...
Got dependencies!
Use Case 2: Add a Package Dependency to an App
Code:
flutter pub add package1 package2
Motivation:
Imagine you’re building a Flutter app and you need to handle HTTP requests efficiently. To achieve this, you decide to include packages like http
and dio
, which are popular for network requests in Flutter. Using flutter pub add
simplifies adding these packages to your project without the hassle of manually editing the pubspec.yaml
file. This approach streamlines the process, ensuring you can leverage these packages quickly to enhance your application’s functionality.
Explanation:
flutter
: Indicates the use of Flutter’s tools.pub
: Specifies the package manager under Flutter.add
: This command is an instruction to import new dependencies to the project.package1 package2
: These are placeholder names for the actual packages you wish to include. When you replace them with real package names, Flutter automatically updatespubspec.yaml
to include these dependencies and fetches them.
Example Output:
Upon successful execution, Flutter will confirm the addition of each package, and afterward, you should see something like:
Resolving dependencies...
+ package1 1.2.3
+ package2 4.5.6
Changed 2 dependencies!
Use Case 3: Remove a Package Dependency from an App
Code:
flutter pub remove package1 package2
Motivation:
There are times during development when specific packages become obsolete or get replaced by better options. In such cases, you may want to remove unnecessary dependencies to optimize your app’s performance and reduce its size. Using flutter pub remove
is an efficient way to declutter your project without directly manipulating the pubspec.yaml
file. This enhances code maintainability and prevents potential conflicts arising from obsolete libraries.
Explanation:
flutter
: Calls the Flutter framework to execute the command.pub
: Engages with Flutter’s package management tool.remove
: The keyword instructs the package manager to eliminate specified dependencies from the project.package1 package2
: These serve as placeholders for the names of the packages you wish to remove. Replacing them with actual package names will updatepubspec.yaml
and eliminate these packages from the project.
Example Output:
Upon execution, the terminal will provide feedback about the packages recently removed:
Resolving dependencies...
- package1 (was 1.2.3)
- package2 (was 4.5.6)
Changed 2 dependencies!
Use Case 4: Upgrade to the Highest Version of a Package Allowed by pubspec.yaml
Code:
flutter pub upgrade package
Motivation:
Keeping your dependencies up-to-date ensures you benefit from the latest features, improvements, and security fixes. With flutter pub upgrade
, you can elevate a package to its newest version that adheres to the constraints specified in your pubspec.yaml
. Regular updates are critical for a project’s longevity, providing enhanced performance, new functionalities, and protection against vulnerabilities.
Explanation:
flutter
: Executes the command using the Flutter toolkit.pub
: Involves Flutter’s dependency manager in the operation.upgrade
: This option instructs the manager to seek out newer package versions, within allowed constraints, to replace the current package.package
: Represents the specific package you wish to upgrade. Simply replace the placeholder with the actual package name to update it.
Example Output:
Upon execution, you might observe the following upgrade confirmation:
Resolving dependencies...
+ package 2.4.7 (was 2.4.5)
Changed 1 dependency!
Conclusion:
Utilizing flutter pub
commands effectively enables developers to manage their Flutter project’s dependencies with ease. Whether ensuring that all necessary packages are downloaded, adding new libraries, removing obsolete ones, or upgrading to newer versions, these commands streamline the workflow, allowing developers to focus more on building innovative and efficient applications. Integrating these practices within your development routine can significantly accelerate your development process and improve overall application quality.