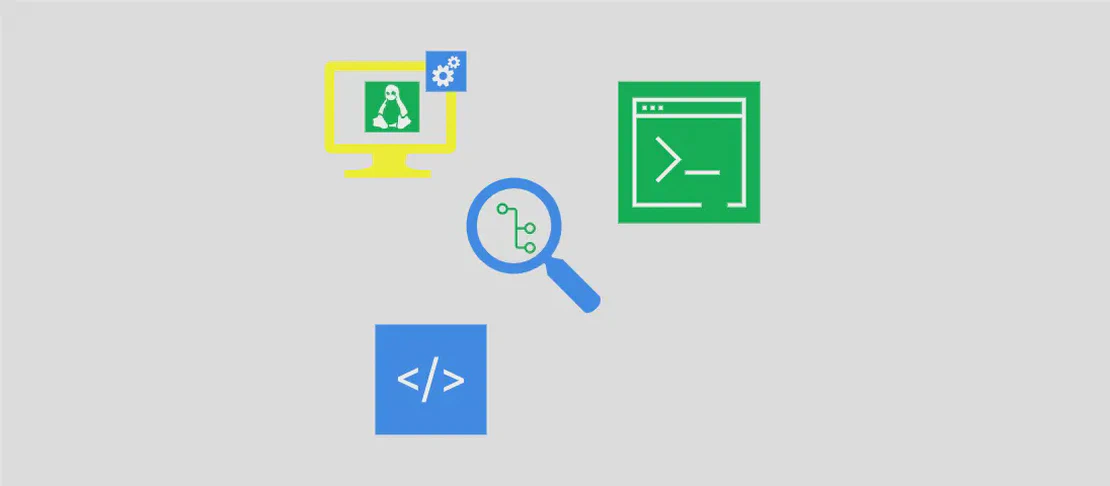
How to effectively use the 'for' command in Bash (with examples)
The for
loop is a versatile loop construction in Bash scripting, allowing users to execute commands multiple times, iterate over a list of items, or automate repetitive tasks. The loop structure iterates over a series of values and performs specified commands on each value, making it a powerful tool in scripting and automation.
Use case 1: Execute the given commands for each of the specified items
Code:
for variable in item1 item2 item3; do echo "Loop is executed"; done
Motivation: This use case is ideal when you need to perform the same operation on a predefined list of items. It can save time and reduce human error when working with multiple elements, such as processing a set of user inputs or data entries.
Explanation:
for variable
: Initiates the beginning of a for loop by setting the iteration variablevariable
.in item1 item2 item3
: Specifies a list of items (item1
,item2
,item3
) over which the loop will iterate.do
: Indicates the start of the block of code to be executed for each item.echo "Loop is executed"
: The command executed during each iteration of the loop.done
: Marks the end of the loop.
Example Output:
Loop is executed
Loop is executed
Loop is executed
Use case 2: Iterate over a given range of numbers
Code:
for variable in {1..10..2}; do echo "Loop is executed"; done
Motivation: Iterating over numerical ranges is particularly useful for tasks such as batch processing files by index, scheduling repetitive processes, or conducting iterations in a numerical calculation. It offers concise syntax for working directly with number sequences.
Explanation:
for variable
: Establishes the loop and definesvariable
to hold each number in the range.in {1..10..2}
: Defines a range from 1 to 10, incrementing by 2. The..
syntax helps automate loops over numeric sequences with specified steps.do
: Begins the loop’s command set.echo "Loop is executed"
: Command executed at each loop iteration.done
: Concludes the loop.
Example Output:
Loop is executed
Loop is executed
Loop is executed
Loop is executed
Loop is executed
Use case 3: Iterate over a given list of files
Code:
for variable in path/to/file1 path/to/file2 path/to/file3; do echo "Loop is executed"; done
Motivation: When dealing with file processing, applying the same operation to multiple files, such as formatting content or renaming, can be repetitive. Using a loop to iterate through files makes script-based automation more manageable and reduces manual errors.
Explanation:
for variable
: Initiates the loop, wherevariable
represents each file name.in path/to/file1 path/to/file2 path/to/file3
: Lists the file paths over which the loop iterates.do
: Begins the execution block for each file.echo "Loop is executed"
: Acts as a placeholder for the file processing command you’ll implement.done
: Ends the loop.
Example Output:
Loop is executed
Loop is executed
Loop is executed
Use case 4: Iterate over a given list of directories
Code:
for variable in path/to/directory1/ path/to/directory2/ path/to/directory3/; do echo "Loop is executed"; done
Motivation: The ability to iterate through directories is useful for administrative tasks such as backup creation, permission modification, or directory-specific operations (e.g., cleaning up temporary files across multiple directories).
Explanation:
for variable
: Sets up the loop withvariable
to iterate through directory names.in path/to/directory1/ path/to/directory2/ path/to/directory3/
: Specifies directory paths for looping.do
: Denotes the start of the command sequence.echo "Loop is executed"
: To be replaced with directory operation commands.done
: Marks loop completion.
Example Output:
Loop is executed
Loop is executed
Loop is executed
Use case 5: Perform a given command in every directory
Code:
for variable in */; do (cd "$variable" || continue; echo "Loop is executed"); done
Motivation: Navigating through each subdirectory to perform operations like version control tasks or content indexing is frequent in systems administration. This script automates processing each directory without manual navigation or error susceptibility.
Explanation:
for variable
: Establishes the loop withvariable
representing each directory in the current path.in */
: Wildcard*/
selects all directories in the current directory.do
: Begins the code block.(cd "$variable" || continue; echo "Loop is executed")
: Attempts to change into each directory (cd "$variable"
), continuing only if successful, then executes a placeholder command, which can be altered as needed.done
: Ends the loop.
Example Output:
Loop is executed
Loop is executed
Loop is executed
Conclusion:
The for
command in Bash is a powerful tool for scripting repetitive tasks over items, numbers, files, or directories. Its adaptability makes it vital for streamlining operations and automating processes, ultimately enhancing efficiency and minimizing errors in diverse computing environments.