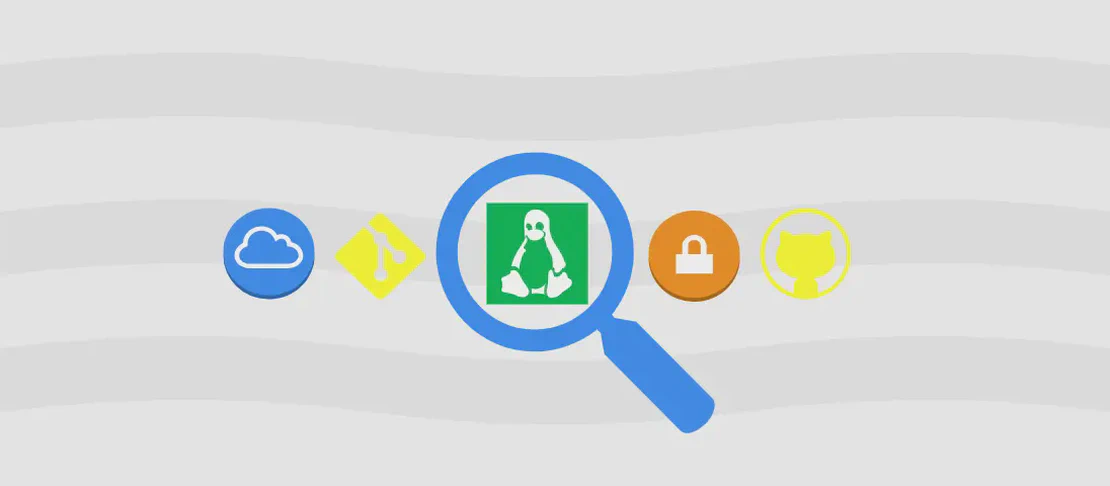
Exploring the 'for' Command in Windows Command Prompt (with examples)
- Windows
- December 17, 2024
The for
command in the Windows Command Prompt is a versatile tool used to execute commands multiple times based on various conditions. It allows for looping over sets, ranges, files, and directories, making it a powerful utility for automating repetitive tasks. This command can be used in batch files or directly in the command prompt for automating and streamlining workflows. In this article, we’ll explore several use cases of the for
command, demonstrating its flexibility and practical applications with examples.
Execute given commands for the specified set
Code:
for %variable in (item_a item_b item_c) do (echo Loop is executed)
Motivation: The need to perform the same action on a specified set of items is common in various scenarios. This use case is perfect when you want to perform simple but repetitive tasks across a defined list, such as generating reports, validating files, or even checking server statuses across a group of server names.
Explanation:
%variable
: A placeholder used to temporarily store each item from the specified set during each iteration of the loop.(item_a item_b item_c)
: The set of elements for which the command inside the loop block will be executed.do (echo Loop is executed)
: Specifies the action to be performed for each item. In this case, it simply echoes a message to the screen.
Example Output:
Loop is executed
Loop is executed
Loop is executed
For each item (item_a, item_b, item_c), the command within the loop block (echo Loop is executed
) is executed, and the message is printed to the screen.
Iterate over a given range of numbers
Code:
for /l %variable in (1, 1, 3) do (echo Loop is executed)
Motivation: Iterating over a range of numbers is useful for tasks that involve numeric progression or threshold checks, such as polling a service every few seconds or iterating through figures for mathematical operations or logging activities iteratively.
Explanation:
/l
: Indicates that thefor
command will iterate over a range of numbers.%variable
: A placeholder to hold the current number in the sequence during each iteration.(1, 1, 3)
: Defines the range and step, starting from 1, increasing by 1 each time, and ending at 3.do (echo Loop is executed)
: The command executed for each number in the range.
Example Output:
Loop is executed
Loop is executed
Loop is executed
The command prints “Loop is executed” three times, once for each number in the range 1 to 3.
Iterate over a given list of files
Code:
for %variable in (path\to\file1.ext path\to\file2.ext) do (echo Loop is executed)
Motivation: Automations involving file manipulation, such as backups, conversions, or deletions, often require operating over a list of files. This command enables the automatique execution of file operations without manual intervention.
Explanation:
%variable
: A placeholder to hold each file path during each iteration.(path\to\file1.ext path\to\file2.ext ...)
: The list of file paths upon which the command will be executed.do (echo Loop is executed)
: Specifies the command to be executed, in this example, simply printing a message.
Example Output:
Loop is executed
Loop is executed
The command results in the message being printed once for each file path in the list.
Iterate over a given list of directories
Code:
for /d %variable in (path\to\directory1 path\to\directory2) do (echo Loop is executed)
Motivation: Directory operations, such as cleanup tasks, file distribution across directories, or even verification of directory contents, can be effectively managed using this command, which automates iterative processes across directories.
Explanation:
/d
: Directs thefor
command to iterate over directories rather than files or other items.%variable
: A placeholder to hold each directory path during each iteration.(path\to\directory1 path\to\directory2 ...)
: The list of directory paths to iterate over.do (echo Loop is executed)
: Specifies the command to execute for each directory.
Example Output:
Loop is executed
Loop is executed
This result shows the message was printed twice, once for each listed directory.
Perform a given command in every directory
Code:
for /d %variable in (*) do (if exist %variable echo Loop is executed)
Motivation: When working with multiple directories, there might be operations or checks that need to be applied universally. This loop structure allows users to automate the execution of commands in all directories within a folder, facilitating bulk processing or checks.
Explanation:
/d
: Indicates the operation will apply broadly across directories.%variable
: Holds the name of each directory during each loop iteration.(*)
: Represents a wildcard character, denoting all directories in the current location.(if exist %variable echo Loop is executed)
: A conditional check within the loop to only execute the command if a directory exists.
Example Output:
Loop is executed
Loop is executed
...
This message is printed once for each existing directory in the current directory where the command is executed.
Conclusion:
The for
command in Windows Command Prompt is a powerful utility that provides flexibility in performing repetitive or batch operations across different kinds of data sets or system structures. Its ability to iterate over individual items, numeric ranges, files, and directories makes it indispensable for script automation, system administration, and efficient task handling in both personal and professional settings. By understanding and leveraging these use cases, users can optimize their command prompt interactions significantly.