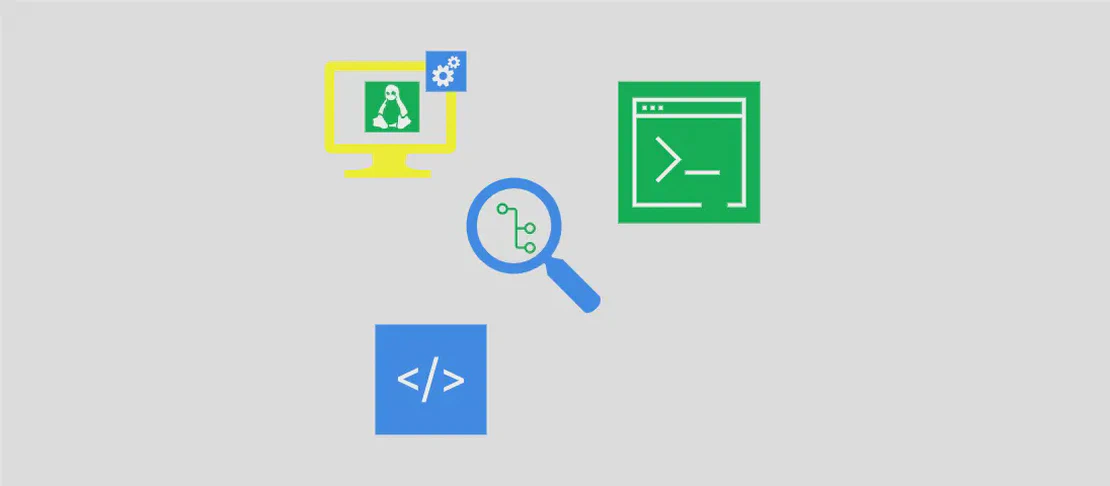
How to use the command 'fselect' (with examples)
‘fselect’ is a command-line tool that allows users to query files using SQL-like statements. This tool is particularly useful for users who need to perform complex queries on file systems without writing extensive scripts or switching between different command-line utilities. By leveraging SQL-like syntax, ‘fselect’ provides a familiar environment for those accustomed to SQL databases, making the process of selecting, filtering, and outputting file information more intuitive and efficient.
Use case 1: Select full path and size from temporary or configuration files in a given directory
Code:
fselect size, path from path/to/directory where name = '*.cfg' or name = '*.tmp'
Motivation:
Temporary and configuration files often accumulate in directories, sometimes consuming significant disk space or causing clutter. Identifying these files is crucial for system maintenance and optimization. This command allows users to quickly locate and assess the impact of such files, facilitating cleanup and management operations.
Explanation:
size, path
: These are the columns being selected, representing the size and full path of each file.from path/to/directory
: Specifies the directory in which to perform the search.where name = '*.cfg' or name = '*.tmp'
: Filters the search results to include only files with .cfg or .tmp extensions, which typically represent configuration and temporary files, respectively.
Example output:
512 /path/to/directory/config.cfg
1024 /path/to/directory/temp.tmp
256 /path/to/directory/backup.cfg
Use case 2: Find square images
Code:
fselect path from path/to/directory where width = height
Motivation:
Square images are often used in design and web applications due to their uniform dimensions, making them ideal for profiles, icons, or thumbnails. Locating these images can aid designers or developers in organizing their assets or preparing batches for specific uses.
Explanation:
path
: Specifies the column to be selected, in this instance, the file path.from path/to/directory
: Designates the directory to query.where width = height
: A condition that filters files to find only those images where the width and height are the same, indicating a square shape.
Example output:
/path/to/directory/square_image1.png
/path/to/directory/thumb.png
Use case 3: Find old-school rap 320kbps MP3 files
Code:
fselect path from path/to/directory where genre = Rap and bitrate = 320 and mp3_year lt 2000
Motivation:
Music aficionados or archivists might desire to locate high-quality, high-bitrate MP3 files of a particular genre from a specific era, such as old-school rap before 2000. This command helps efficiently retrieve these files for listening, archiving, or analysis.
Explanation:
path
: Specifies the file path to be selected.from path/to/directory
: Identifies the location to search for files.where genre = Rap
: Filters files classified under the ‘Rap’ genre.and bitrate = 320
: Ensures that the MP3 files have a bitrate of 320 kbps, indicating high audio quality.and mp3_year lt 2000
: Limits results to files released before the year 2000.
Example output:
/path/to/directory/rap_classic.mp3
/path/to/directory/old_rap_hit.mp3
Use case 4: Select only the first 5 results and output as JSON
Code:
fselect size, path from path/to/directory limit 5 into json
Motivation:
When working in environments that necessitate limited data retrieval or testing, selecting a subset of results can be critical. Furthermore, outputting in JSON format is advantageous for integration with web services or software development processes, allowing for standardized data handling.
Explanation:
size, path
: The data fields to be selected, representing file size and path.from path/to/directory
: Specifies where within the file system to execute the query.limit 5
: Restricts the results to just five entries, useful for sampling or debugging.into json
: Formats the output in JSON, making it machine-readable and easily consumable by other programs or services.
Example output:
[
{"size": 1024, "path": "/path/to/directory/file1.txt"},
{"size": 2048, "path": "/path/to/directory/file2.txt"},
{"size": 512, "path": "/path/to/directory/file3.txt"},
{"size": 256, "path": "/path/to/directory/file4.txt"},
{"size": 4096, "path": "/path/to/directory/file5.txt"}
]
Use case 5: Use SQL aggregate functions to calculate minimum, maximum and average size of files in a directory
Code:
fselect "MIN(size), MAX(size), AVG(size), SUM(size), COUNT(*) from path/to/directory"
Motivation:
Data analysis often requires summary statistics to understand a dataset better. By calculating the minimum, maximum, average, sum, and count of file sizes, users can gain insights into disk usage patterns and identify potential issues, such as unusually large files or the total number of files in a directory.
Explanation:
MIN(size), MAX(size), AVG(size), SUM(size), COUNT(*)
: Aggregate functions that compute the respective minimum size, maximum size, average size, total size, and file count in the directory.from path/to/directory
: Indicates the target directory to analyze.
Example output:
MIN(size): 128
MAX(size): 16384
AVG(size): 2048
SUM(size): 51200
COUNT(*): 25
Conclusion:
The ‘fselect’ command is a powerful tool that effectively bridges the gap between file system management and database query capabilities. Through its SQL-like syntax, users can perform detailed queries and aggregations on their file systems, streamlining tasks such as data cleanup, organizational structuring, and resource analysis. Whether one is searching for specific media files, analyzing disk space, or filtering file types, ‘fselect’ provides an efficient and flexible solution to meet various needs.