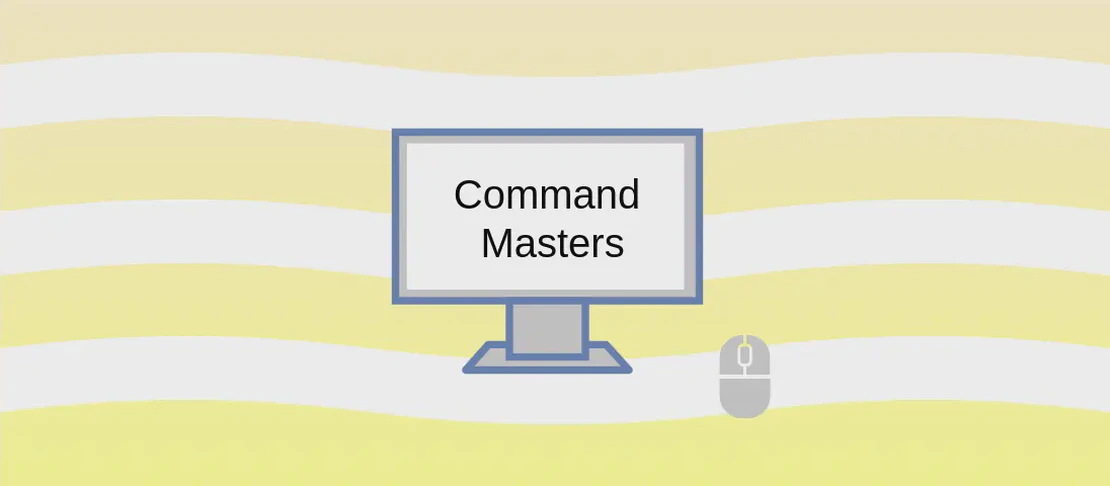
How to Use the Command 'fswatch' (with examples)
fswatch
is a powerful and flexible command-line tool designed to monitor file changes across platforms such as Linux, macOS, and BSD. It listens for changes in files and directories and can trigger specific actions when modifications are detected. Whether you’re a developer monitoring source files, a system administrator keeping an eye on configuration files, or just someone interested in tracking changes to important documents, fswatch
provides a comprehensive solution for all these needs.
Use case 1: Run a Bash command on file creation, update, or deletion
Code:
fswatch path/to/file | xargs -n 1 bash_command
Motivation:
This use case is particularly useful for developers or system administrators who need to automate tasks in response to file changes. For example, if a configuration file is updated, an immediate action may be required to reload a service or compile new code automatically. This automation saves time and reduces the risk of manual errors.
Explanation:
fswatch
initiates the monitoring process on the specifiedpath/to/file
.- The
|
character pipes the output fromfswatch
to another command. xargs
takes input fromfswatch
and executesbash_command
each time a change is detected.-n 1
ensuresbash_command
is executed once per input line.
Example Output:
Suppose bash_command
is set to update a log file:
[INFO] File at path/to/file was modified
[INFO] File at path/to/file was modified
Use case 2: Watch one or more files and/or directories
Code:
fswatch path/to/file path/to/directory path/to/another_directory/**/*.js | xargs -n 1 bash_command
Motivation:
Monitoring multiple files and directories is essential in large projects where changes can occur in various locations simultaneously. Whether it’s JavaScript files in a directory or configuration settings spread across different folders, fswatch
can handle these more complex monitoring needs effortlessly.
Explanation:
fswatch
is set to observe multiple paths:path/to/file
,path/to/directory
, and all.js
files underpath/to/another_directory
, specified using the**/*.js
wildcard for recursive file inclusion.- As changes occur in the monitored locations, they are piped into
xargs
. xargs -n 1 bash_command
executes the specifiedbash_command
for each change detected, once per input line.
Example Output:
Upon watching changes in a set of JavaScript files:
[INFO] JavaScript file at path/to/another_directory/subdir/a.js was modified
[INFO] JavaScript file at path/to/another_directory/subdir/b.js was deleted
Use case 3: Print the absolute paths of the changed files
Code:
fswatch path/to/directory | xargs -n 1 -I {} echo {}
Motivation:
Sometimes, it’s valuable to have a record of files that were altered without needing to take immediate action. This might be useful for auditing purposes, maintaining records of file operations in a system, or simply for debugging when trying to trace the source of unexpected changes.
Explanation:
fswatch
monitorspath/to/directory
for any modifications.- The output is directed through a pipe to
xargs
. xargs -n 1 -I {}
specifies a placeholder{}
that will be replaced with each detected event.echo {}
displays the path of each changed file.
Example Output:
As files change in the given directory, it logs their paths:
/full/path/to/directory/file1.txt
/full/path/to/directory/file2.txt
Use case 4: Filter by event type
Code:
fswatch --event Updated|Deleted|Created path/to/directory | xargs -n 1 bash_command
Motivation:
Filtering events is critical when only certain types of changes matter. For instance, a team might only be concerned about deletions of important data files or the creation of new user accounts in a directory. With this filtering capability, fswatch
can prioritize specific actions based on the occurrence of these targeted events.
Explanation:
fswatch
starts watching for changes withinpath/to/directory
.- The
--event
option filters changes based on specified types:Updated
,Deleted
, andCreated
. - Upon detection of these specific event types, the results are piped to
xargs
. xargs -n 1 bash_command
ensures thatbash_command
is invoked for each significant event.
Example Output:
If the specified event is a file update:
File /path/to/directory/file3.txt was updated
Conclusion:
The fswatch
tool stands out with its ability to monitor files across platforms and execute commands based on varied change types. With these illustrative examples, users can harness fswatch
for numerous applications, from automating workflow tasks and improving productivity to tracking important file modifications seamlessly.