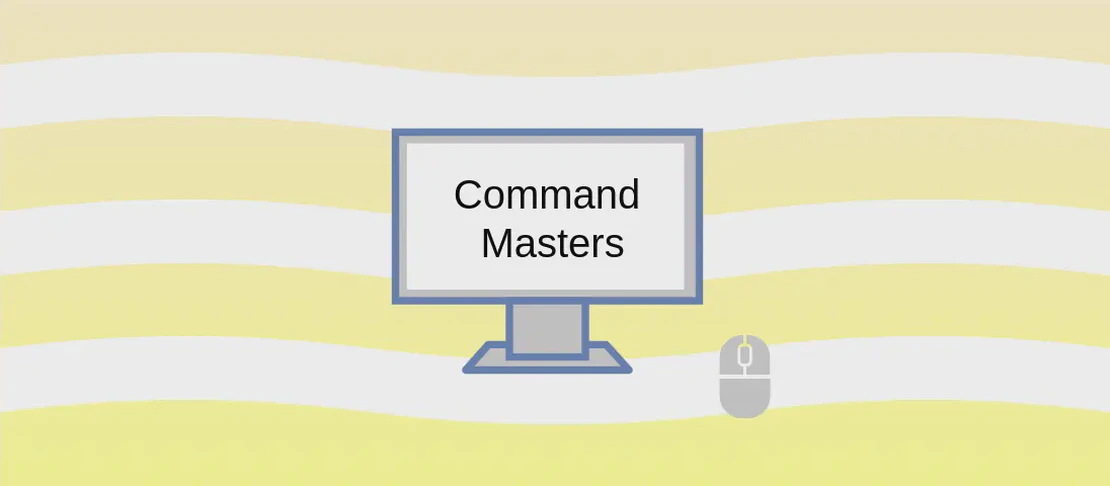
Exploring the Command-Line Fuzzy Finder 'fzf' (with examples)
The fzf
command-line utility is a powerful fuzzy finder that empowers users to efficiently search, filter, and select data from potentially vast lists directly from the terminal. Whether working with files, large datasets, or process lists, fzf
provides an intuitive interface for navigating complex data landscapes. This versatility makes it an invaluable tool for developers, system administrators, and data scientists who seek to streamline their workflow through more efficient command-line operations.
Use Case 1: Start fzf
on All Files in the Specified Directory
Code:
find path/to/directory -type f | fzf
Motivation:
Imagine you are working on a project with thousands of files scattered across different directories and subdirectories. Quickly locating a specific file can become a tedious task. fzf
simplifies this by providing an interactive way to search through files, dramatically reducing the time needed to find what you’re looking for.
Explanation:
find path/to/directory -type f
: This command lists all files (-type f
) in the specifiedpath/to/directory
.find
navigates through directories and subdirectories to generate a comprehensive file list.| fzf
: The pipe (|
) takes the output of thefind
command and passes it as input tofzf
. The user is then presented with an interface to interactively search through and select a file from the list.
Example Output:
Using fzf
, you’d see a list of filenames, and typing part of a filename would filter down to those matching your input. Once you land on the desired file, selecting it completes the process.
Use Case 2: Start fzf
for Running Processes
Code:
ps aux | fzf
Motivation:
System administrators often need to manage and monitor running processes to ensure system health. With numerous processes running simultaneously, manually locating a specific process can be challenging. fzf
eases this by allowing the user to search and filter processes interactively.
Explanation:
ps aux
: This command lists all the currently running processes on the system, providing information such as the user, CPU usage, memory usage, and command run.| fzf
: Thefzf
tool receives the running process list, letting users quickly search and select a particular process entry, useful for subsequent management tasks like killing a process or checking its performance.
Example Output:
You’d see a list of processes displayed in a table format, and could quickly filter down to, say, processes running under your username by typing relevant keywords.
Use Case 3: Select Multiple Files with Shift + Tab
and Write to a File
Code:
find path/to/directory -type f | fzf --multi > path/to/file
Motivation:
Let’s say you need to batch-select files for copying or archiving them. Doing this manually could be time-consuming. With fzf
, you can easily select multiple files using a simple keystroke.
Explanation:
find path/to/directory -type f
: As previously described, this command lists all files.fzf --multi
: The--multi
option allows users to select multiple items from the list. HoldingShift
and pressingTab
selects or deselects entries.> path/to/file
: The selected files’ paths are written topath/to/file
, allowing you to use this list later in scripts or other operations.
Example Output:
After interacting with fzf
, you’ll have a generated file (path/to/file
) containing the paths of the selected files, ready to be utilized in further processing.
Use Case 4: Start fzf
with a Specified Query
Code:
fzf --query "query"
Motivation:
When you already have a definite keyword or pattern in mind, launching fzf
with that as the starting point saves time by instantly narrowing down the choices to only relevant entries.
Explanation:
fzf --query "query"
: The--query
option preloads the search interface with the specified “query”, thereby pre-filtering initial search results.
Example Output:
fzf
displays only those items that match “query” instantly, so when you start the tool, you’re immediately focused on a subset of interest.
Use Case 5: Start fzf
on Entries That Start with ‘core’ and End with Either ‘go’, ‘rb’, or ‘py’
Code:
fzf --query "^core go$ | rb$ | py$"
Motivation:
In scenarios where you’re dealing with files like configurations or source code spread across different languages or implementations, filtering by complex patterns (start and end conditions) can help you efficiently target relevant files.
Explanation:
fzf --query "^core go$ | rb$ | py$"
: This complex query command:^core
: Matches entries that begin with “core”.go$ | rb$ | py$
: Further narrows down to entries ending with “go”, “rb”, or “py”.
Example Output:
Only files such as core.go
, core.rb
, or core.py
are listed, letting you instantly identify files pertinent to those specific programs.
Use Case 6: Start fzf
on Entries That Do Not Match ‘pyc’ and Match Exactly ’travis’
Code:
fzf --query "!pyc 'travis"
Motivation:
During code deployment or review sessions, you might want to exclude irrelevant files (e.g., compiled bytecode like .pyc
) while focusing on specific configuration files (like ’travis.yml’) to ensure accurate review or manipulation.
Explanation:
fzf --query "!pyc 'travis"
: Here’s a breakdown:!pyc
: Excludes any entries that include the substring “pyc”.'travis'
: Matches entries that contain the exact substring “travis”.
Example Output:
After initiating fzf
with this query, you’d see results like travis.yml
and others containing “travis”, excluding all entries with “pyc”.
Conclusion:
With its dynamic searching capabilities, fzf
greatly enhances the functionality of the command line, providing users with a robust toolkit for data selection and filtering across various scenarios. As demonstrated by these examples, fzf
dramatically reduces the complexity and time involved in traditional text-based searching, offering tailored solutions for different data management tasks.