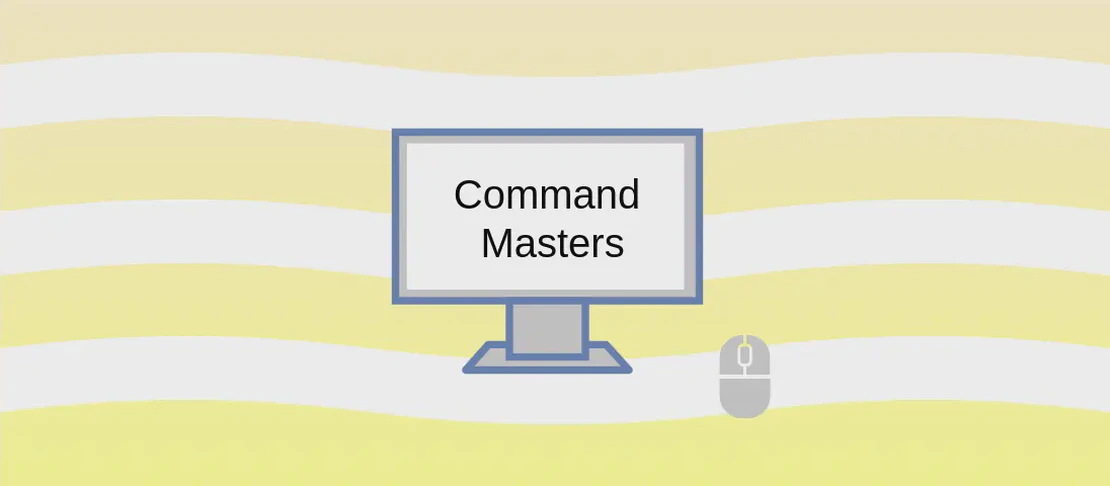
How to use the command 'g++' (with examples)
The g++
command is a part of the GNU Compiler Collection (GCC) and is predominantly used to compile C++ source files into executable binaries. As a powerful and flexible compiler, g++
supports various language standards and optimization levels, ensuring that developers have the necessary tools for any project, ranging from small personal programs to large-scale enterprise applications. More information can be found at https://gcc.gnu.org
.
Use case 1: Compile a source code file into an executable binary
Code:
g++ path/to/source1.cpp path/to/source2.cpp ... -o path/to/output_executable
Motivation:
When you write a C++ program, it often consists of multiple source files that need to be compiled and linked together to create a single executable. Compiling individual source files into one executable is a fundamental procedure that turns your readable C++ code into machine code, which your computer can execute.
Explanation:
path/to/source1.cpp path/to/source2.cpp ...
: These represent the paths to your C++ source files. You can include as many source files as necessary. The files you list here are translated from C++ into machine code by the compiler.-o path/to/output_executable
: This option specifies the name and path of the resulting executable binary. If you don’t use the-o
option,g++
will generate an executable with a default name, usuallya.out
.
Example Output:
$ ./output_executable
Hello, World!
Use case 2: Activate output of all errors and warnings
Code:
g++ path/to/source.cpp -Wall -o output_executable
Motivation:
Errors and warnings are crucial for debugging and ensuring code quality. Using -Wall
, developers can detect potential problems in the code that could lead to bugs or undefined behavior. It is a good practice to enable all warnings to maintain high-quality and reliable software.
Explanation:
path/to/source.cpp
: The path to the C++ source file you want to compile.-Wall
: This flag enables all the compiler’s warning messages. Using this flag acts as a safety check against common pitfalls in C++ programming.-o output_executable
: Specifies the name for the output file of the compiled program.
Example Output:
warning: 'int x' defined but not used [-Wunused-variable]
Use case 3: Show common warnings, debug symbols in output, and optimize without affecting debugging
Code:
g++ path/to/source.cpp -Wall -g -Og -o path/to/output_executable
Motivation:
During the development phase, it is often necessary to debug the program to find and fix issues, while also keeping an eye on performance. Using the -g
flag with -Og
allows you to generate debug symbols and optimize your code simultaneously without degrading the debugging process, thereby creating a good balance between debuggability and performance.
Explanation:
path/to/source.cpp
: Path to the source file.-Wall
: Enables all warnings.-g
: Generates debugging information which can be used by a debugger to step through your code.-Og
: Optimizes the code with a focus on making the debug experience better and preserving essential debugging information.-o path/to/output_executable
: The output executable file.
Example Output:
Reading symbols from path/to/output_executable...
Use case 4: Choose a language standard to compile for (C++98/C++11/C++14/C++17)
Code:
g++ path/to/source.cpp -std=c++11 -o path/to/output_executable
Motivation:
C++ has evolved over the years, introducing various language standards. Each standard includes new features, performance improvements, and enhancements. Specifying the C++ standard version ensures that your code is compliant with the features and syntax changes of that particular version, which is essential for compatibility and making use of modern C++ features.
Explanation:
path/to/source.cpp
: The source file to be compiled.-std=c++11
: This option sets the language standard to C++11. You can switch betweenc++98
,c++03
,c++14
,c++17
, and others depending on the features you wish to use.-o path/to/output_executable
: The resulting executable.
Example Output:
$ ./output_executable
C++11 specific feature output
Use case 5: Include libraries located at a different path than the source file
Code:
g++ path/to/source.cpp -o path/to/output_executable -Ipath/to/header -Lpath/to/library -llibrary_name
Motivation:
In many projects, you’ll use external libraries that provide additional functionality. These libraries may have custom locations. By specifying the include path and linking libraries, you can incorporate these libraries into your project, expanding its capabilities beyond the standard library.
Explanation:
path/to/source.cpp
: Source code file.-o path/to/output_executable
: Specifies output filename.-Ipath/to/header
: Adds an additional directory to the list of directories to be searched for headers.-Lpath/to/library
: Adds a directory to the list of directories to be searched for libraries during linking.-llibrary_name
: Links with the library namedlibrary_name
. This does not include thelib
prefix or file extension.
Example Output:
Successfully linked to external library.
Use case 6: Compile and link multiple source code files into an executable binary
Code:
g++ -c path/to/source1.cpp path/to/source2.cpp ... && g++ -o path/to/output_executable path/to/source1.o path/to/source2.o ...
Motivation:
In large projects, it’s common to separate the compilation and linking process. This can save time, as you can recompile only the modified source files and then link the object files. This method optimizes the build process, particularly important when dealing with numerous files.
Explanation:
-c path/to/source1.cpp path/to/source2.cpp ...
: Compiles the source files into object files (.o
files) without linking.&&
: A shell command separator that triggers the execution of the next command only if the preceding command was successful.g++ -o path/to/output_executable path/to/source1.o path/to/source2.o ...
: Links the object files into an executable.
Example Output:
$ ./output_executable
Program output from compiled multiple source files
Use case 7: Optimize the compiled program for performance
Code:
g++ path/to/source.cpp -O2 -o path/to/output_executable
Motivation:
Optimization is crucial for performance-critical applications where execution speed and efficiency are priorities. By specifying optimization flags such as -O2
, the compiler attempts to improve the program performance. This is particularly important in applications like game engines, simulations, or any software requiring high efficiency.
Explanation:
path/to/source.cpp
: Source code file to optimize.-O2
: This optimization level enables nearly all supported optimizations that do not involve a space-speed tradeoff. It provides a good balance between speed and size.-o path/to/output_executable
: The path for the optimized executable.
Example Output:
Optimized binary created
Use case 8: Display version
Code:
g++ --version
Motivation:
Knowing the version of the g++
compiler can be essential for debugging, compatibility checks, ensuring compliance with the project’s requirements, or when reporting bugs. It helps you understand the features available and any limitations in your current compiler version.
Explanation:
--version
: Displays the version number of the installedg++
compiler along with relevant information.
Example Output:
g++ (Ubuntu 10.3.0-1ubuntu1) 10.3.0
Copyright (C) 2020 Free Software Foundation, Inc.
Conclusion
The g++
command offers a wide range of functionalities catered to different stages of C++ program development. From compiling simple executable files to optimizing code for speed, or incorporating external libraries, g++
proves itself as an invaluable tool in the developer’s toolkit. Understanding these command examples and their motivations ensures a streamlined development process, apt resource management, and deployment of high-quality C++ applications.