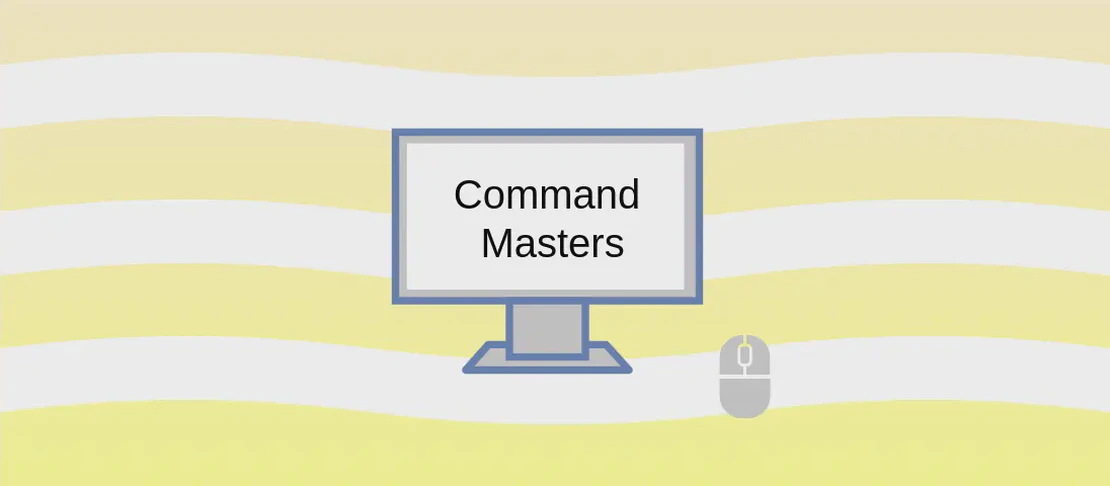
How to use the command 'gcc' (with examples)
gcc is a popular compiler command used to preprocess, compile, and link C and C++ source files. It offers a range of options to optimize the program, handle libraries, and generate different types of output files. This article will provide examples of various use cases of the gcc command.
Use case 1: Compile multiple source files into an executable
Code:
gcc path/to/source1.c path/to/source2.c ... -o path/to/output_executable
Motivation: Compiling multiple source files into a single executable allows developers to organize their code into separate modules or libraries. This improves code maintainability and reusability.
Explanation:
path/to/source1.c path/to/source2.c
: Specifies the path to the source files that need to be compiled.-o path/to/output_executable
: Specifies the location and name of the output executable file.
Example output: If we execute the following command:
gcc src/main.c src/utilities.c -o bin/myprogram
The gcc command will compile the main.c and utilities.c files located in the “src” directory and create an executable named “myprogram” in the “bin” directory.
Use case 2: Show common warnings, debug symbols in output, and optimize without affecting debugging
Code:
gcc path/to/source.c -Wall -g -Og -o path/to/output_executable
Motivation: When debugging or analyzing code, it is important to have access to meaningful warnings and debug symbols. However, optimizing the code can improve performance without significantly affecting the debugging experience.
Explanation:
-Wall
: Enables all common warning messages.-g
: Includes debugging information in the output file.-Og
: Enables optimization suitable for debugging.-o path/to/output_executable
: Specifies the location and name of the output executable file.
Example output: If we execute the following command:
gcc src/main.c -Wall -g -Og -o bin/myprogram
The gcc command will compile the main.c file with all common warnings enabled, including debug symbols. The resulting executable named “myprogram” will be available in the “bin” directory.
Use case 3: Include libraries from a different path
Code:
gcc path/to/source.c -o path/to/output_executable -Ipath/to/header -Lpath/to/library -llibrary_name
Motivation: Libraries provide pre-existing code that can be used by developers to extend their applications. By specifying the library header and path, developers can utilize external libraries in their code.
Explanation:
-Ipath/to/header
: Specifies the path to the header files of the external library.-Lpath/to/library
: Specifies the path to the library files.-llibrary_name
: Links the specified library during compilation.-o path/to/output_executable
: Specifies the location and name of the output executable file.
Example output: If we execute the following command:
gcc src/main.c -o bin/myprogram -I/usr/local/include -L/usr/local/lib -lmylibrary
The gcc command will compile the main.c file with the header file located in “/usr/local/include” and link the associated library file located in “/usr/local/lib”. The resulting executable named “myprogram” will be available in the “bin” directory.
Use case 4: Compile source code into Assembler instructions
Code:
gcc -S path/to/source.c
Motivation: Understanding the generated Assembler instructions can help analyze, optimize, or debug the compiled code. This option allows developers to obtain the Assembler code without generating an executable.
Explanation:
-S
: Instructs the compiler to generate Assembler instructions instead of an object file or executable.path/to/source.c
: Specifies the path to the source file that needs to be compiled.
Example output: If we execute the following command:
gcc -S src/main.c
The gcc command will compile the main.c file and generate an Assembler file named “main.s” in the same directory. The “main.s” file will contain the generated Assembler instructions.
Use case 5: Compile source code into an object file without linking
Code:
gcc -c path/to/source.c
Motivation: Sometimes it is necessary to compile the source code into an object file without immediate linking. This can be useful when creating libraries or when the final executable will be linked at a later stage, allowing for separate compilation and linking phases.
Explanation:
-c
: Instructs the compiler to compile the source file into an object file without linking.path/to/source.c
: Specifies the path to the source file that needs to be compiled.
Example output: If we execute the following command:
gcc -c src/main.c
The gcc command will compile the main.c file and generate an object file named “main.o” in the same directory. The “main.o” file will contain the compiled object code that can be linked later.
Use case 6: Optimize the compiled program for performance
Code:
gcc path/to/source.c -O1|2|3|fast -o path/to/output_executable
Motivation: Optimization techniques can significantly improve the performance of the compiled program. By specifying an optimization level, developers can fine-tune the balance between compilation speed and the resulting performance.
Explanation:
-O1|2|3|fast
: Specifies the optimization level. “O1” represents a moderate optimization level, and “O3” represents the highest optimization level. Alternatively, “fast” can be used to optimize for performance with less regard for code size or compilation time.path/to/source.c
: Specifies the path to the source file that needs to be compiled.-o path/to/output_executable
: Specifies the location and name of the output executable file.
Example output: If we execute the following command:
gcc src/main.c -O2 -o bin/myprogram
The gcc command will compile the main.c file with the optimization set to level 2. The resulting executable named “myprogram” will be available in the “bin” directory.
Conclusion:
The gcc command is a powerful tool for compiling C and C++ code. It provides a range of options to optimize the code, handle libraries, and generate different output files. By understanding and utilizing these options effectively, developers can enhance the performance and functionality of their programs.