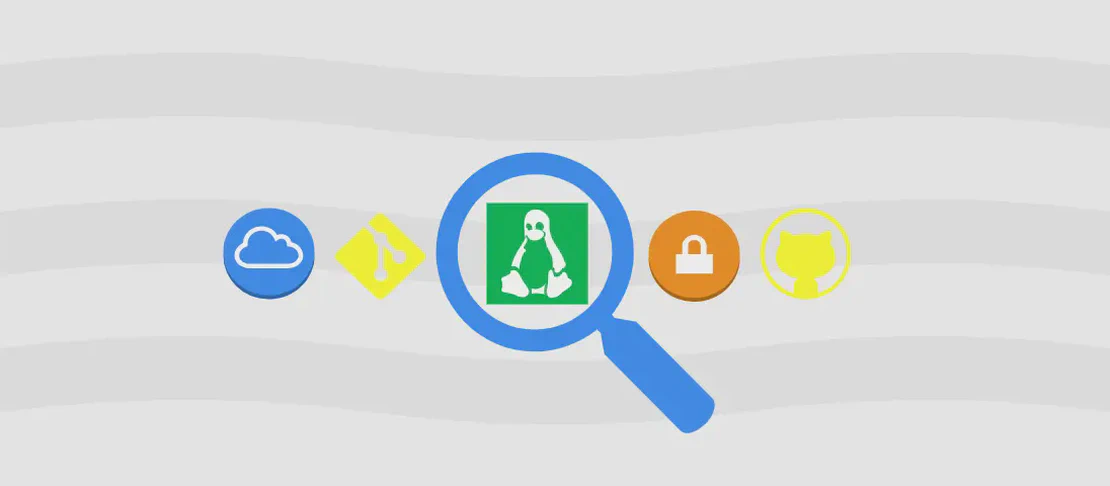
How to use the command `gcov` (with examples)
- Linux
- December 25, 2023
The gcov
command is a code coverage analysis and profiling tool that helps track the untested parts of a program. It generates a coverage report and annotates the source code with execution frequencies of code segments. This tool is useful for identifying areas of code that can benefit from additional testing and optimization.
Use case 1: Generate a coverage report (file.cpp.gcov)
Code:
gcov path/to/file.cpp
Motivation:
Generating a coverage report is the primary use case of the gcov
command. By running this command, you can obtain a detailed report that shows which parts of your code were executed during testing and which parts were not. This information can be used to improve test coverage and identify areas that require additional attention.
Explanation:
gcov
- This is the command name.path/to/file.cpp
- This argument specifies the path to the source file for which you want to generate the coverage report.
Example output:
Running 'gcov' on 'path/to/file.cpp'
File 'path/to/file.cpp'
Lines executed:100.00% of 50
Creating 'path/to/file.cpp.gcov'
In this example, the gcov
command was run on the file.cpp
source file located at the given path. The output indicates that all lines were executed, and a coverage report named file.cpp.gcov
was created.
Use case 2: Write individual execution counts for every basic block
Code:
gcov --all-blocks path/to/file.cpp
Motivation:
Sometimes, it can be helpful to have detailed information about execution counts at the basic block level. This use case allows you to see the number of times each basic block was executed during testing. By analyzing this information, you can gain insights into the execution flow and identify potential performance bottlenecks.
Explanation:
--all-blocks
- This argument tellsgcov
to write individual execution counts for every basic block in the coverage report.path/to/file.cpp
- This argument specifies the path to the source file for which you want to generate the coverage report.
Example output:
Running 'gcov' on 'path/to/file.cpp'
File 'path/to/file.cpp'
Function 'main'
Lines executed:100.00% of 50
Block execution frequencies:
1% #1
2% #2
5% #3
...
Creating 'path/to/file.cpp.gcov'
In this example, the gcov
command was run with the --all-blocks
option on the file.cpp
source file. The output displays the execution frequencies for each basic block in the main
function.
Use case 3: Write branch frequencies and print summary information as a percentage
Code:
gcov --branch-probabilities path/to/file.cpp
Motivation:
When analyzing code coverage, it can be valuable to examine the branch frequencies to gain insight into the dynamics of conditional statements. This use case allows you to generate a coverage report with branch frequencies and obtain summary information presented as percentages. This information helps you identify branches that are seldom or never taken during testing.
Explanation:
--branch-probabilities
- This option enablesgcov
to write branch frequencies to the output coverage report.path/to/file.cpp
- This argument specifies the path to the source file for which you want to generate the coverage report.
Example output:
Running 'gcov' on 'path/to/file.cpp'
File 'path/to/file.cpp'
Lines executed:100.00% of 50
Branches executed:95.00% of 20
Taken at least once:75.00%
Creating 'path/to/file.cpp.gcov'
In this example, the gcov
command was used with the --branch-probabilities
option on the file.cpp
source file. The output shows that 95% of the branches were executed, and at least one branch was taken in 75% of the cases.
Use case 4: Write branch frequencies as the number of branches taken
Code:
gcov --branch-counts path/to/file.cpp
Motivation:
To gain a more detailed understanding of branch execution, it can be useful to see the actual count of branches taken rather than just the percentages. This use case allows you to generate a coverage report with branch counts, enabling you to analyze the distribution of branch executions and identify potential areas for optimization or additional testing.
Explanation:
--branch-counts
- This option instructsgcov
to write branch frequencies as the number of branches taken to the output coverage report.path/to/file.cpp
- This argument specifies the path to the source file for which you want to generate the coverage report.
Example output:
Running 'gcov' on 'path/to/file.cpp'
File 'path/to/file.cpp'
Lines executed:100.00% of 50
Branches executed:95 of 100
Creating 'path/to/file.cpp.gcov'
In this example, the gcov
command was executed with the --branch-counts
option on the file.cpp
source file. The output indicates that out of the 100 branches, 95 were executed during testing.
Use case 5: Do not create a gcov output file
Code:
gcov --no-output path/to/file.cpp
Motivation:
In some cases, you may want to run gcov
for its profiling capabilities without generating an output coverage report. This use case allows you to analyze the execution frequencies and branch information without cluttering your workspace with gcov files.
Explanation:
--no-output
- This option preventsgcov
from creating an output coverage report.path/to/file.cpp
- This argument specifies the path to the source file for which you want to generate the coverage report.
Example output:
Running 'gcov' on 'path/to/file.cpp'
File 'path/to/file.cpp'
Lines executed:100.00% of 50
Branches executed:95.00% of 20
Taken at least once:75.00%
In this example, the gcov
command was used with the --no-output
option on the file.cpp
source file. The output provides the execution frequencies and branch information, but no coverage report file is created.
Use case 6: Write file level as well as function level summaries
Code:
gcov --function-summaries path/to/file.cpp
Motivation:
To understand the coverage pattern at a higher level, it can be helpful to obtain both file-level and function-level summaries. This use case allows you to generate a coverage report that includes information about coverage at both levels, making it easier to identify specific functions or files that require attention.
Explanation:
--function-summaries
- This option tellsgcov
to write file-level as well as function-level summaries in the coverage report.path/to/file.cpp
- This argument specifies the path to the source file for which you want to generate the coverage report.
Example output:
Running 'gcov' on 'path/to/file.cpp'
File 'path/to/file.cpp'
Lines executed:100.00% of 50
Creating 'path/to/file.cpp.gcov'
Function 'main'
Lines executed:100.00% of 20
Function 'helper'
Lines executed:75.00% of 30
In this example, the gcov
command was executed with the --function-summaries
option on the file.cpp
source file. The output includes both file-level and function-level summaries, providing coverage information for each individual function.
Conclusion:
The gcov
command is a powerful tool for code coverage analysis and profiling. By utilizing its different options and arguments, you can generate detailed reports, analyze execution frequencies, and gain insight into the untested parts of your code. This information helps you improve the quality and efficiency of your programs by identifying areas that require additional testing or optimization.