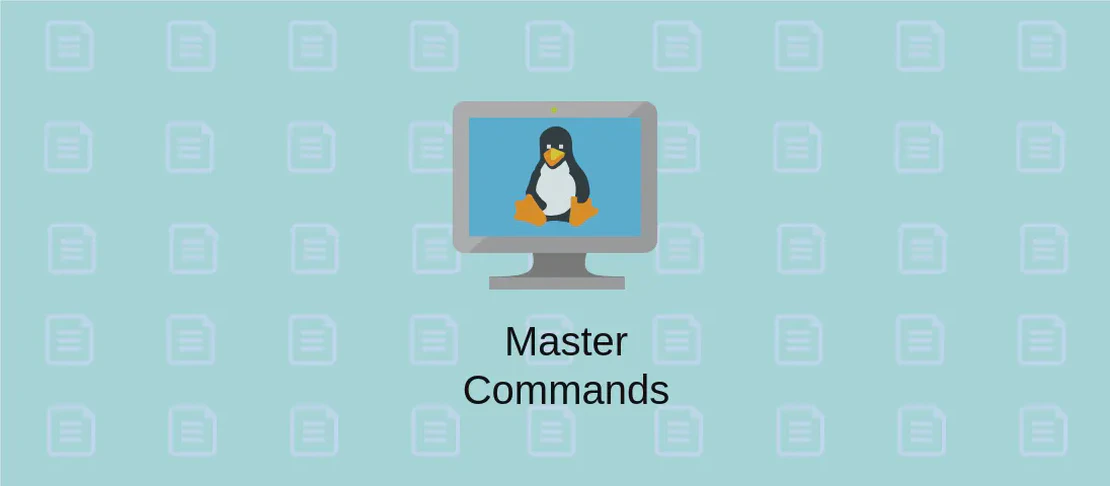
How to use the command 'gdb' (with examples)
The GNU Debugger (gdb) is a powerful tool utilized by developers for debugging applications. It aids in following the execution of programs, viewing what happens when an application’s code runs, examining variables, and determining why a program may crash. GDB is invaluable in the troubleshooting process, providing a more in-depth analysis of software and assisting in pinpointing the root of problems.
Use case 1: Debug an executable
Code:
gdb executable
Motivation: Developers often encounter scenarios where the code behaves unexpectedly, ultimately causing the application to produce incorrect results or fail altogether. Utilizing gdb
to debug an executable allows developers to step into the code during runtime, set breakpoints, and examine the application’s state. This method provides the opportunity to directly observe and investigate issues within the executable.
Explanation:
gdb
: Invokes the GNU Debugger.executable
: The target application or program that needs debugging. This argument specifies the compiled code that requires analysis for errors or misbehavior.
Example output:
When executed, gdb
opens an interactive session which may look like this:
GNU gdb (GDB) 10.2
...
(gdb)
Use case 2: Attach a process to gdb
Code:
gdb -p procID
Motivation: There are circumstances where a long-running process needs troubleshooting without stopping the application. For example, if an application hanging or crashing sporadically, attaching gdb
to a running process can help. This allows the developer to diagnose problems in real-time without restarting or stopping the program.
Explanation:
-p
: This option specifies thatgdb
should attach to a running process.procID
: The process ID of the application you wish to debug. This ID is a unique identifier assigned by the operating system to every running process.
Example output:
When executed, gdb
provides feedback that it is attached:
Attaching to process 1234
...
(gdb)
Use case 3: Debug with a core file
Code:
gdb -c core executable
Motivation: When an application crashes, operating systems can produce a core dump—a file that records the memory and state of the program at the moment of failure. Debugging with such a file helps in diagnosing and fixing the issues that led to the crash without needing to reproduce the problem immediately.
Explanation:
-c
: This option tellsgdb
to use a core dump file.core
: This is the core file generated from the crashed process.executable
: The program related to the core dump. It provides the corresponding symbolic information to the debugger.
Example output:
Upon running the command, gdb
loads the core file for analysis:
Core was generated by `./executable'.
Program terminated with signal 11, Segmentation fault.
...
(gdb)
Use case 4: Execute given GDB commands upon start
Code:
gdb -ex "commands" executable
Motivation: Automating the debugging process with pre-set commands can streamline frequent debugging tasks or initialize specific environments. This is especially useful when performing repetitive or script-based debugging sessions, ensuring consistency and saving developer’s time by automatically executing a sequence of gdb
commands.
Explanation:
-ex
: Directly provides a command to execute upon startup."commands"
: A quoted string specifying the sequence ofgdb
commands. It might include setting breakpoints, running the application, or any othergdb
command.executable
: The program to debug, allowing such automation to apply to the specified application.
Example output:
Running the example might begin gdb
with pre-configured settings:
Breakpoint 1 at 0x112b: file main.c, line 10.
(gdb) run
Starting program: /path/to/executable
...
Use case 5: Start gdb
and pass arguments to the executable
Code:
gdb --args executable argument1 argument2
Motivation: Often, programs require certain arguments to simulate real-world scenarios or specific test cases. This capability permits users to investigate how applications handle different inputs directly, helping to identify problems related to argument parsing or input-based logic faults.
Explanation:
--args
: This option tellsgdb
that the following arguments are for the executable being debugged.executable
: The program being debugged, which may require additional command-line arguments.argument1 argument2
: Represents the arguments that are passed to the executable. These mimic real-world input scenarios or specific parameters for the application.
Example output:
Upon execution, gdb
initiates the program with the provided arguments, displaying:
(gdb) run
Starting program: /path/to/executable argument1 argument2
...
Conclusion:
The gdb
command provides an expansive suite of debugging capabilities crucial for software development and maintenance. By leveraging specific commands and configurations, developers can investigate, simulate, and diagnose various runtime issues, leading to more robust and error-resistant applications.