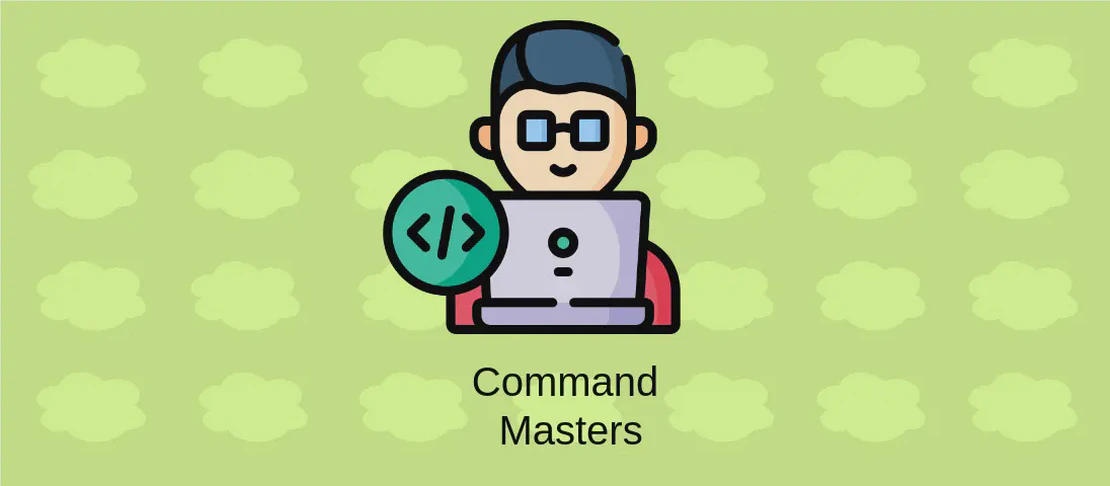
How to Use the Command 'gdc' (with examples)
‘gdc’ stands for the GNU D Compiler, which utilizes GCC (GNU Compiler Collection) as its backend. It is specifically designed for compiling programs written in the D programming language, offering compatibility with GCC’s extensive optimizations and features. ‘gdc’ allows developers to efficiently compile D code into executable binaries, generate documentation, and perform various other tasks to streamline D development. More information can be found on the official D wiki here .
Use case 1: Create an Executable
Code:
gdc path/to/source.d -o path/to/output_executable
Motivation:
When developing software, one of the primary tasks is converting source code into an executable format that can be run directly on a machine. This is crucial for testing, deployment, and distribution. Using ‘gdc’ to compile D programs allows developers to transform their D source files into executable binaries efficiently.
Explanation:
gdc
: This is the command used to invoke the GNU D Compiler, which works with D language files.path/to/source.d
: This specifies the path to the D source file that you want to compile. The.d
extension is typically used for D language source files.-o
: This stands for “output.” It is used to specify the name of the resulting output executable file.path/to/output_executable
: This defines the path and name where the compiled executable will be stored.
Example output:
If the source file compiles successfully, no output will be displayed. If there are errors, error messages detailing the issues will be presented. Successful execution yields a binary executable named output_executable
.
Use case 2: Print Information about Module Dependencies
Code:
gdc -fdeps
Motivation:
Understanding module dependencies is essential for D developers in managing complex projects. When multiple modules and packages are involved, insights into how they depend on each other can facilitate debugging, optimization, and refactoring. This knowledge is critical in avoiding circular dependencies and ensuring efficient compilation.
Explanation:
gdc
: The command starts the D compiler process.-fdeps
: This flag tells ‘gdc’ to output information about the module dependencies. It provides insights into which modules are used by others, helping in managing and organizing code.
Example output:
The command will output a list of module dependencies showing which files or modules depend on others. This information is usually printed to the terminal.
Use case 3: Generate Ddoc Documentation
Code:
gdc -fdoc
Motivation:
Documentation is a crucial aspect of software development. It helps developers understand code functionality, usage, and integration points. Generating documentation directly from source code annotations ensures that documentation stays up-to-date with the codebase. ‘gdc’ facilitates this by creating documentation in Ddoc format, a native documentation system for D.
Explanation:
gdc
: Initiates the D compiler.-fdoc
: This flag instructs gdc to generate documentation from the D source files. The resulting documentation incorporates comments and structure definitions, organized in a readable format.
Example output:
This command does not display output to the terminal unless there are errors. Instead, documentation files are generated, typically in HTML or a specific Ddoc format, detailing the APIs and components of the source code.
Use case 4: Generate D Interface Files
Code:
gdc -fintfc
Motivation:
Interface files in D are essential for separating interface from implementation. They allow developers to share the API of a module or library without exposing its internal workings. This is particularly useful for library authors who want to provide headers for their code.
Explanation:
gdc
: The compiler command being executed here.-fintfc
: This flag is used to create D interface files. These files generally contain only the public declarations needed to interact with the module, hiding the private implementation details.
Example output:
Upon execution, gdc creates .di
interface files corresponding to the input modules. These files contain public interfaces, which help in preserving encapsulation and abstracting implementation details.
Use case 5: Do Not Link the Standard GCC Libraries in Compilation
Code:
gdc -nostdlib
Motivation:
There are scenarios where developers need to customize the linking process, perhaps to reduce binary size, omit certain features, or resolve conflicts with custom libraries. The -nostdlib
option offers significant control over the linking phase by omitting the GCC standard libraries.
Explanation:
gdc
: This begins the compilation process using the D compiler.-nostdlib
: This instructs ‘gdc’ to skip linking against the standard GCC libraries. It’s beneficial when the application uses different or custom versions of these libraries.
Example output:
This command will produce an output executable without the linked standard libraries. However, if the source code references any symbols provided by these libraries, linking errors might occur due to missing symbols.
Conclusion:
‘gdc’ provides a versatile set of functionalities for developers working with the D programming language. Each of these use cases highlights a different aspect of how ‘gdc’ can be used to generate executables, manage dependencies, produce documentation, interact with interfaces, and manipulate linking in diverse development scenarios. Understanding these commands enhances a developer’s ability to optimize and control their D software projects.