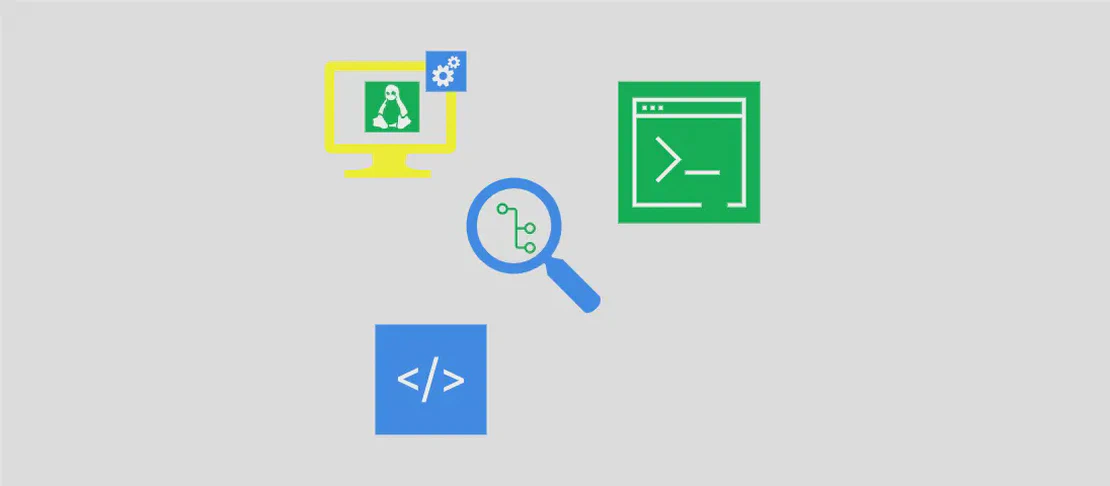
How to use the command 'gfortran' (with examples)
GFortran is a part of the GNU Compiler Collection (GCC) that is specifically tailored for compiling Fortran programs. It takes Fortran source code, preprocesses it, compiles it, assembles, and finally links it into executable programs. GFortran is a versatile tool that supports various Fortran standards and provides a rich set of compiler options aimed at performance enhancement, debugging, and code optimization.
Use Case 1: Compile multiple source files into an executable
Code:
gfortran path/to/source1.f90 path/to/source2.f90 ... -o path/to/output_executable
Motivation:
In complex software projects, code is typically split into multiple source files to promote modularity and reusability. By compiling these files together, a developer can create a comprehensive executable that links all individual pieces into a cohesive program. This use case is essential for building applications that are larger in scope and depend on different modules for functionality.
Explanation:
gfortran
: Calls the GFortran compiler.path/to/source1.f90 path/to/source2.f90
: Specifies the paths to the Fortran source files that need to be compiled. In a real-world scenario, you might have numerous files listed.-o path/to/output_executable
: The-o
option indicates the name and location of the resulting executable file produced by the compilation process.
Example Output:
An executable file named as specified in -o path/to/output_executable
is created, consolidating the functionality and logic contained in the input source files.
Use Case 2: Show common warnings, debug symbols in output, and optimize without affecting debugging
Code:
gfortran path/to/source.f90 -Wall -g -Og -o path/to/output_executable
Motivation:
When developing software, it’s vital to highlight potential issues in the code by using compiler warnings. This ensures higher code quality and fewer runtime errors. Debug symbols make the process of tracking and fixing bugs easier by retaining information about the source code in the compiled program. And optimization (-Og
) allows for a balance between execution speed and debuggability, which is particularly useful during development.
Explanation:
gfortran
: Initiates the GFortran compiler.path/to/source.f90
: Path to the source file to be compiled.-Wall
: Displays all common warning messages which help identify potential issues in the code.-g
: Includes debug information in the output executable, aiding in debugging processes.-Og
: Optimizes the code to improve speed while retaining sufficient debug information, ensuring debugging tools can function correctly.-o path/to/output_executable
: Determines the name and location of the compiled executable.
Example Output:
The output is an executable containing debugging information and optimized code, making it suitable for developmental testing and troubleshooting while highlighting potential issues with warnings.
Use Case 3: Include libraries from a different path
Code:
gfortran path/to/source.f90 -o path/to/output_executable -Ipath/to/mod_and_include -Lpath/to/library -llibrary_name
Motivation:
In scenarios where external libraries or modules are required to supplement the program’s functionalities, it’s necessary to point the compiler to the correct paths that contain these dependencies. This ensures that all referenced functions and methods are adequately linked during the compilation process.
Explanation:
gfortran
: Executes the GFortran compiler.path/to/source.f90
: Path to the main source code file.-o path/to/output_executable
: Specifies the output executable file.-Ipath/to/mod_and_include
: Adds a directory to the list for searching include files or modules, allowing access to additional required headers or modules.-Lpath/to/library
: Adds a directory to the library search path, ensuring any included libraries can be found.-llibrary_name
: Links the specified library by name;-l
followed by the library name tells GFortran to include this library during the linking phase.
Example Output:
An executable is produced that fully incorporates the functionalities from the main source file and any external libraries specified, ready for execution with all dependencies resolved.
Use Case 4: Compile source code into Assembler instructions
Code:
gfortran -S path/to/source.f90
Motivation:
Generating assembler instructions can be useful for developers who need to explore low-level implementation details for optimization and understanding how high-level Fortran code translates into machine code. It’s particularly beneficial in performance-critical applications to assess instruction-level behavior.
Explanation:
gfortran
: Begins the compilation process.-S
: Directs the compiler to stop after the assembling step and output assembler instructions, foregoing the linking step.path/to/source.f90
: The path to the Fortran source file you wish to convert to assembly language.
Example Output:
An assembly file (.s
extension) is generated, which contains the assembler instructions for the respective Fortran source code, giving insights into the generated low-level code.
Use Case 5: Compile source code into an object file without linking
Code:
gfortran -c path/to/source.f90
Motivation:
Creating object files is a common practice in software development where separate modules are compiled independently. Building object files allows for incremental compilation, where only changed modules need recompiling. This saves time during the build process of large applications.
Explanation:
gfortran
: Invokes the compiler.-c
: Compiles the given source file into an object file (.o) but does not link, which is useful for modular projects where linking occurs later.path/to/source.f90
: Directs the compiler to the source file that needs to be compiled.
Example Output:
An object file (.o
) corresponding to the input source code is produced. This file can later be linked with other object files or libraries to create an executable.
Conclusion:
GFortran is a powerful and flexible tool for developers working with Fortran, providing a range of functionalities essential for modern software development. From compiling multi-file projects to integrating external libraries, generating assembly code, and producing debug-ready executables, GFortran simplifies the complex process of code development and optimization in Fortran. Its versatility makes it an invaluable asset in both academic and commercial settings, where performance and precision are paramount.