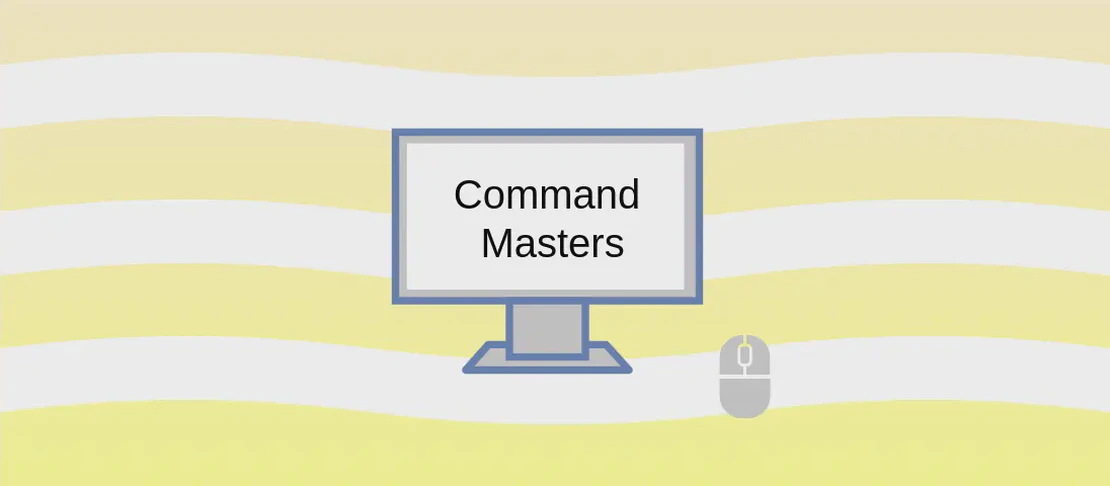
How to use the command 'gh api' (with examples)
The gh api
command is a versatile tool from the GitHub CLI that allows users to make authenticated HTTP requests to the GitHub API. This tool is particularly useful for developers who need to interact with GitHub’s vast array of features programmatically without directly dealing with authentication complexities. With gh api
, users can fetch repository data, manipulate issues, automate workflows, and more, making it a powerful utility for automating tasks in CI/CD pipelines or during development.
Use case 1: Display the releases for the current repository in JSON format
Code:
gh api repos/:owner/:repo/releases
Motivation:
In many development workflows, automatically fetching release information from a repository is crucial. For instance, when building a continuous deployment pipeline, knowing the latest release version can determine which version of the software to deploy. Displaying the releases in JSON format allows easy parsing and manipulation in scripts or applications, enabling seamless integration into automated systems.
Explanation:
gh api
: This calls the command to make a request to the GitHub API.repos/:owner/:repo/releases
: Specifies the API endpoint to fetch releases.:owner
is a placeholder for the repository owner, and:repo
represents the repository name. Replace these placeholders with actual values to target a specific repository.
Example Output:
[
{
"url": "https://api.github.com/repos/owner/repo/releases/1",
"id": 1,
"tag_name": "v1.0.0",
"name": "Initial Release",
...
},
...
]
Use case 2: Create a reaction for a specific issue
Code:
gh api --header Accept:application/vnd.github.squirrel-girl-preview+json --raw-field 'content=+1' repos/:owner/:repo/issues/123/reactions
Motivation:
Reactions to issues provide a way for users to express agreement or acknowledgment without posting additional comments. This is particularly useful in issue tracking, where quick feedback is necessary from numerous collaborators. Automating this process can streamline feedback collection and reduce noise in discussions.
Explanation:
gh api
: Initiates an authenticated API request.--header Accept:application/vnd.github.squirrel-girl-preview+json
: Sets a custom header to specify that the request is using the preview version of the reactions API, which might not be fully stable but provides reaction functionality.--raw-field 'content=+1'
: This specifies the payload, in this case, adding a “+1” reaction to show approval on the issue.repos/:owner/:repo/issues/123/reactions
: Targets the API endpoint for a specific issue’s reactions, where:owner
,:repo
, and123
should be replaced with the actual owner, repo name, and issue number, respectively.
Example Output:
{
"id": 1,
"user": {
"login": "user123",
"id": 456,
...
},
"content": "+1",
...
}
Use case 3: Display the result of a GraphQL query in JSON format
Code:
gh api graphql --field name=':repo' --raw-field 'query'
Motivation:
GraphQL allows developers to request specific data efficiently, which is especially beneficial when working with large datasets. By using GraphQL with GitHub’s API, developers can fetch precise information tailored to their application’s needs, reducing data processing and bandwidth usage.
Explanation:
gh api graphql
: Instructsgh api
to use GitHub’s GraphQL API instead of the REST API.--field name=':repo'
: Declares a variable for the query. Here,:repo
should be replaced with the repository name.--raw-field 'query'
: This specifies the raw GraphQL query to be executed. Users will need to replace'query'
with the desired GraphQL query string.
Example Output:
{
"data": {
"repository": {
"name": "example",
"stargazers_count": 42,
...
}
}
}
Use case 4: Send a request using a custom HTTP method
Code:
gh api --method POST endpoint
Motivation:
Certain API operations require different HTTP methods (such as POST, PUT, DELETE) to perform actions other than retrieving data, like creating new entities or updating existing ones. This use case enables that functionality, allowing comprehensive interactions with GitHub’s resources.
Explanation:
gh api
: Utilizesgh api
for an authenticated API call.--method POST
: Specifies that the request should use the POST HTTP method, which is often used to create new resources.endpoint
: Represents the desired API endpoint, which the user needs to specify based on the desired action.
Example Output:
Due to the generic nature, the output will depend on the endpoint. For a success response in JSON, you might see:
{
"success": true,
"message": "Resource created successfully",
...
}
Use case 5: Include the HTTP response headers in the output
Code:
gh api --include endpoint
Motivation:
Including HTTP response headers can provide valuable context, such as rate limit status or content type. This is particularly important for debugging and monitoring interactions with the GitHub API, ensuring compliance with rate limits and proper handling of responses.
Explanation:
gh api
: Initiates a GitHub API request.--include
: Indicates that the response should include HTTP headers, adding metadata to the standard response body.endpoint
: The specific API endpoint the request targets.
Example Output:
HTTP/1.1 200 OK
Date: Wed, 20 Oct 2023 12:34:56 GMT
Content-Type: application/json
...
{
"data": {
...
}
}
Use case 6: Do not print the response body
Code:
gh api --silent endpoint
Motivation:
Silent requests are useful when the primary interest is in the side effects of the API call, rather than the returned data. This is often used in scenarios where a command triggers an action, like a webhook event, and the user doesn’t need to process the response data.
Explanation:
gh api
: Performs an API request.--silent
: Suppresses the printing of the response body. The command will execute the request without outputting data to the console.endpoint
: The API endpoint to send the request to.
Example Output:
No output will be displayed in the console.
Use case 7: Send a request to a specific GitHub Enterprise Server
Code:
gh api --hostname github.example.com endpoint
Motivation:
For organizations using GitHub Enterprise, hitting the correct server is crucial for accessing the appropriate repository and user data. This capability allows users to interact with enterprise-specific instances rather than GitHub’s public offerings, enabling secure and isolated API interactions in enterprise environments.
Explanation:
gh api
: Executes an API request.--hostname github.example.com
: Directs the command to a specific enterprise GitHub server, wheregithub.example.com
should be replaced with the actual enterprise server hostname.endpoint
: Targets the specific API endpoint on the enterprise server.
Example Output:
Output would depend on the endpoint, typically formatted in JSON.
Use case 8: Display the subcommand help
Code:
gh api --help
Motivation:
Knowing how to use command-line tools efficiently can significantly reduce developer time and errors. Accessing the help documentation from the command line provides instant assistance and details about available options, flags, and typical usage scenarios. This can be invaluable for users new to the tool or for quickly verifying command syntax.
Explanation:
gh api
: References the GitHub API command.--help
: Displays help documentation forgh api
, including usage patterns and available options.
Example Output:
Usage: gh api <command> [options]
Options:
--method <METHOD> HTTP method to use: GET, POST, PUT, DELETE, PATCH
--hostname <HOST> Specify a different hostname for GitHub Enterprise
...
Conclusion:
The gh api
command is a flexible and powerful tool for interacting with GitHub’s API, providing a range of functionalities from basic data retrieval to complex automation tasks. By facilitating authenticated requests and offering extensive customization through its options, it serves as an effective bridge between developers and GitHub’s vast ecosystem, streamlining workflows and enhancing productivity. Understanding and utilizing these use cases allows developers to leverage the full potential of GitHub’s API infrastructure directly from their command line.