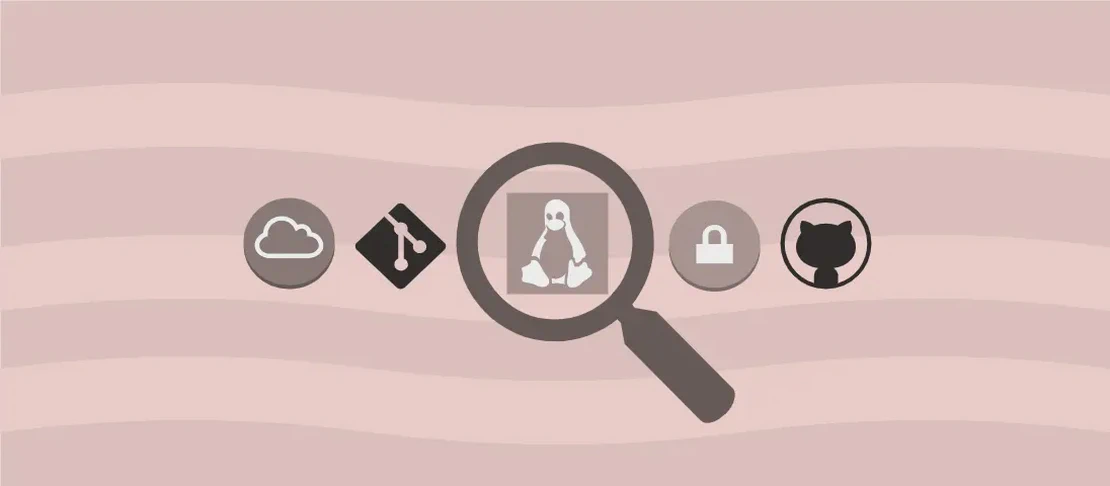
How to use the command 'gh secret' (with examples)
The gh secret
command is part of the GitHub CLI, which allows users to manage secrets within their GitHub repositories and organizations. Secrets are encrypted environment variables that help securely pass sensitive information required for building or deploying applications. With gh secret
, you can effortlessly set, view, and remove secrets in your repositories or across organizations, simplifying workflow automation and securing data integrity.
List Secret Keys for the Current Repository
Code:
gh secret list
Motivation:
Listing secret keys for the current repository is an essential task for developers and project maintainers who need to track what sensitive data is stored in their repository’s secret vault. This can help during audits, security reviews, or when you are unsure of what secrets are available to your GitHub Actions workflows.
Explanation:
gh secret list
: This command fetches and displays all the secret keys stored in the current repository you are working on. By default, it lists all secrets in the present repository context of your command line interface.
Example Output:
NAME UPDATED AT
--------- ---------------
SECRET_1 January 20, 2023
SECRET_2 March 2, 2023
List Secret Keys for a Specific Organization
Code:
gh secret list --org organization
Motivation:
Organizations using GitHub for complex projects often manage numerous secrets across multiple repositories. This command allows organization administrators to gather a concise inventory of present secrets at the organization level, which can enhance security management and streamline compliance processes.
Explanation:
gh secret list
: Initiates the listing process.--org organization
: Specifies the organization from which you want to list secrets. Replace “organization” with your organization’s actual name on GitHub.
Example Output:
NAME UPDATED AT
--------------- ---------------
ORG_SECRET_1 February 15, 2023
ORG_SECRET_2 March 30, 2023
List Secret Keys for a Specific Repository
Code:
gh secret list --repo owner/repository
Motivation:
Listing secrets for a specific repository is particularly useful for maintainers who handle multiple projects and need to inspect secrets related to a particular project only. This ability allows for targeted audits and improved management of repository-specific sensitive data.
Explanation:
gh secret list
: This initiates the list command.--repo owner/repository
: Targets a specific repository. Replace “owner” with the username or organization name and “repository” with the repository name you are querying.
Example Output:
NAME UPDATED AT
------------ ---------------
REPO_SECRET_1 March 5, 2023
REPO_SECRET_2 April 10, 2023
Set a Secret for the Current Repository
Code:
gh secret set name
Motivation:
Setting a secret in the current repository is crucial for inserting sensitive data like API keys, passwords, or other confidential information needed for your application to function or deploy properly. This process ensures that you avoid hardcoding sensitive information within codebases, thus maintaining security best practices.
Explanation:
gh secret set
: Begins the process of setting a new secret key.name
: The placeholder for the secret’s name you wish to add. The user will be prompted to enter the secret value in a secure manner.
Example Output:
✓ Secret 'name' set for the repository.
Set a Secret from a File for the Current Repository
Code:
gh secret set name < path/to/file
Motivation:
Setting a secret from a file is beneficial when dealing with larger pieces of sensitive data or when automating secret management where the secret value is stored in a file. This method minimizes manual input errors and allows for secure batch operations on secrets.
Explanation:
gh secret set
: Initiates the command for setting a new secret.name
: Represents the secret’s designation.< path/to/file
: This redirects the content of a file specified bypath/to/file
into the secret value, ensuring confidentiality.
Example Output:
✓ Secret 'name' set for the repository from file.
Set an Organization Secret for Specific Repositories
Code:
gh secret set name --org organization --repos repository1,repository2
Motivation:
Setting an organization-level secret for specific repositories is strategic for managing shared secrets across multiple projects within an organization. This setup is often required when repositories need common access to shared resources like AWS keys or database credentials without storing them in each repository individually.
Explanation:
gh secret set
: Commences the setting process.name
: Indicates the name to be used for the secret.--org organization
: Names the organization that will own the secret.--repos repository1,repository2
: Specifies the list of repositories that can access the given secret. Replace “repository1” and “repository2” with the actual repository names.
Example Output:
✓ Secret 'name' set for organization 'organization' for repositories 'repository1,repository2'.
Remove a Secret for the Current Repository
Code:
gh secret remove name
Motivation:
Removing a secret from the current repository is an important step when a secret is no longer needed or if it’s been compromised. This action helps maintain a clean and secure environment by ensuring outdated or potentially harmful data is not accessible in your repositories.
Explanation:
gh secret remove
: Engages the removal process for a secret key.name
: Specifies the name of the secret to be removed.
Example Output:
✓ Secret 'name' removed from the repository.
Remove a Secret for a Specific Organization
Code:
gh secret remove name --org organization
Motivation:
In rapidly growing organizations, secret management demands keen oversight. Removing obsolete or compromised organization-level secrets aids in minimizing risks and keeping credentials pertinent and well-maintained.
Explanation:
gh secret remove
: Activates the command to delete a secret.name
: Identifies the specific name of the secret you intend to delete.--org organization
: Defines the organizational context where the secret is being removed.
Example Output:
✓ Secret 'name' removed for organization 'organization'.
Conclusion:
Managing secrets with the gh secret
CLI command is a pivotal task for maintaining secure and efficient workflows in both individual repositories and organization settings on GitHub. Understanding the different contexts in which secrets operate and how to efficiently manage them using the provided command examples, developers and administrators can ensure sensitive data is handled with care, thereby reducing security vulnerabilities and enhancing project management.