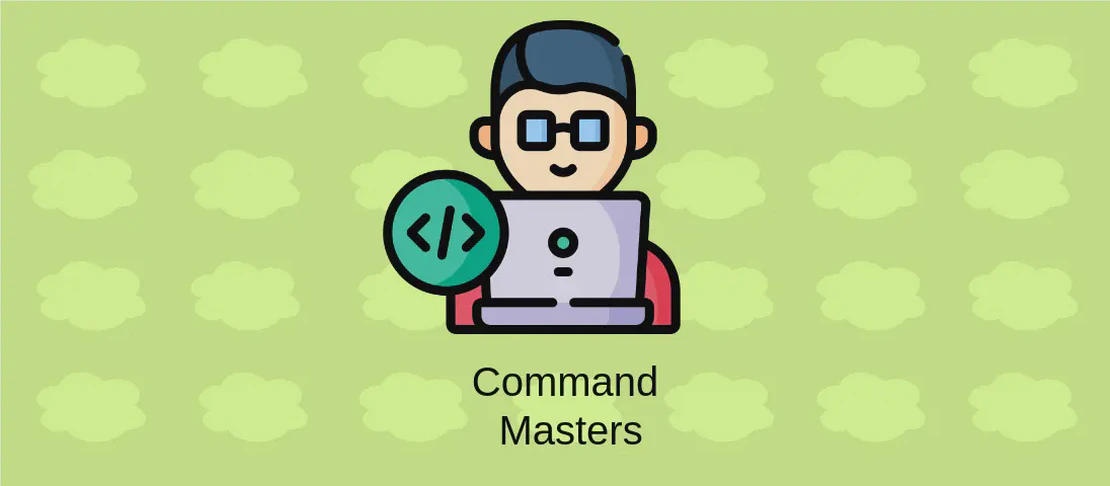
How to Use the Glasgow Haskell Compiler (ghc) (with Examples)
The Glasgow Haskell Compiler (GHC) is an open-source native code compiler for the Haskell programming language. It stands out for its robust optimizations and supports a wide array of platforms. GHC is primarily used for compiling and linking Haskell source files into executable programs, making it an essential tool for developers working within the Haskell ecosystem.
Use case 1: Finding and Compiling All Modules in the Current Directory
Code:
ghc Main
Motivation:
In a Haskell project structure, it’s common to have multiple modules that need to be compiled together. Using ghc
to compile the main module in your project is a straightforward way to ensure that all related and necessary files are compiled. This method saves time and effort, automating the process of handling dependencies between multiple Haskell files.
Explanation:
ghc
: This is the command for the Glasgow Haskell Compiler, which is responsible for compiling Haskell programs.Main
: The main module of the Haskell project. When you specifyMain
, GHC looks for a file namedMain.hs
in the directory, along with other modules it may depend on, and attempts to compile them into an executable.
Example Output:
Upon successful compilation, you’ll get an output indicating that the modules have been compiled and an executable has been created. If there are errors, GHC will present diagnostic messages.
Use case 2: Compiling a Single File
Code:
ghc path/to/file.hs
Motivation:
Compiling a single Haskell file directly is useful during development and testing to ensure individual parts of your codebase work as expected. This can help isolate problems in your code, making it easier to debug specific functions or modules separately from the rest of your program.
Explanation:
path/to/file.hs
: Represents the relative or absolute path to the specific Haskell source file you wish to compile. The extension.hs
indicates a Haskell source file.
Example Output:
If the compilation is successful, GHC will create an object file (and possibly an executable) for the Haskell file specified. The output will include any warnings or errors that occurred.
Use case 3: Compiling with Extra Optimization
Code:
ghc -O path/to/file.hs
Motivation:
When performance is critical, optimizing your Haskell code can make a significant difference. The -O
flag enables GHC’s optimizer, which works to produce faster executable code. This is valuable for performance-sensitive applications or when deploying production-ready code.
Explanation:
-O
: This flag tells GHC to perform optimizations to improve the running time and performance of the compiled code.path/to/file.hs
: The Haskell file to be compiled with optimization settings applied.
Example Output:
Optimized compilation may take longer but generally results in a more performance-efficient executable. GHC provides output on the compilation process including any optimizations applied.
Use case 4: Stopping Compilation After Generating Object Files
Code:
ghc -c path/to/file.hs
Motivation:
Sometimes, you may not need to produce an entire executable but rather an object file, which can later be linked with other object files. Using the -c
flag allows developers to generate object files for use in a modular compilation or linking process.
Explanation:
-c
: This flag indicates that GHC should stop after creating an object file (.o
), without producing a final executable.path/to/file.hs
: The path to the Haskell file to convert into an object file.
Example Output:
The output includes a .o
file corresponding to the source file specified. This can be later used to link with other object files into a complete program.
Use case 5: Starting a REPL (Interactive Shell)
Code:
ghci
Motivation:
The interactive shell (REPL) provided by GHC, called GHCi, is a powerful tool for Haskell developers. It allows for rapid testing and development by evaluating expressions and functions on the fly without creating a standalone application. It’s ideal for those just learning Haskell or for experienced developers prototyping new code.
Explanation:
ghci
: Launches GHC’s interactive environment where developers can enter and execute Haskell expressions interactively.
Example Output:
The shell offers an interactive prompt where you can enter Haskell expressions, receive immediate feedback, and explore different approaches to your coding problems.
Use case 6: Evaluating a Single Expression
Code:
ghc -e expression
Motivation:
Evaluating a single expression using the -e
flag is especially useful for quick calculations or for testing short snippets of Haskell code without setting up a full-fledged project or module. It allows instant execution of Haskell expressions directly from the command line.
Explanation:
-e
: This flag is used to tell GHC you want to evaluate a Haskell expression directly.expression
: The Haskell code to be evaluated (e.g.,print (1 + 2)
) as a string.
Example Output:
Evaluates and prints the result of the expression provided. For instance, if you entered ghc -e "print (1 + 2)"
, the output would be 3
.
Conclusion:
The Glasgow Haskell Compiler (GHC) is a flexible and powerful tool for compiling Haskell programs, offering various features to enhance and streamline the development process. Whether optimizing performance, managing object files, or rapidly testing code in a REPL environment, GHC provides a comprehensive set of capabilities for developers. This versatility makes it indispensable for both learning and professional development in Haskell.