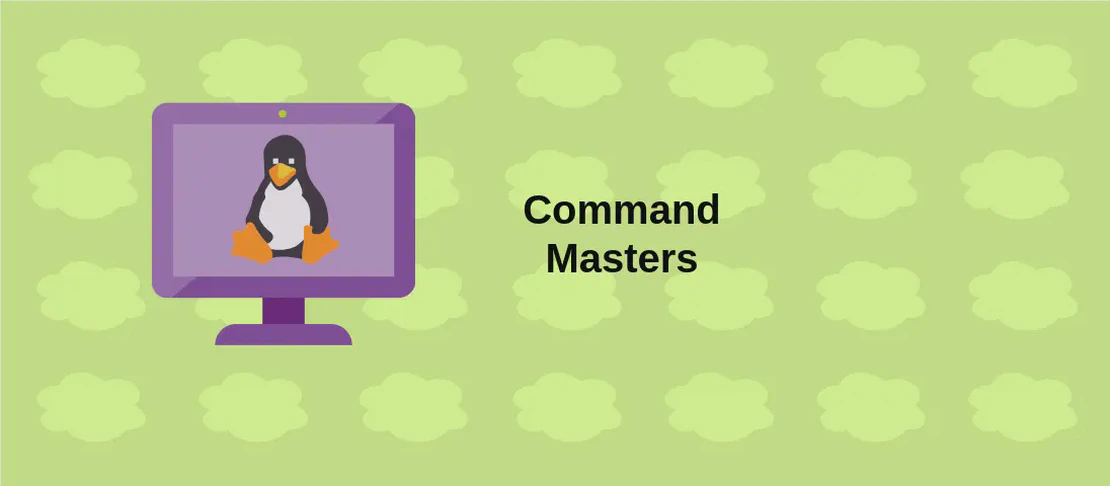
How to use the command 'ghci' (with examples)
The ghci
command initiates the Glasgow Haskell Compiler’s interactive environment, often referred to as the GHCi REPL (Read-Eval-Print Loop). This tool allows users to interactively execute Haskell expressions, test code snippets, and experiment with Haskell features. Serving as a fundamental tool for both beginners and experienced Haskell developers, GHCi provides immediate feedback and a convenient playground for Haskell code development. This article will explore various ways to leverage GHCi with practical examples and explanations.
Use case 1: Start a REPL (interactive shell)
Code:
ghci
Motivation:
Launching GHCi without any specific files or options allows users to enter into the interactive Haskell environment. This is particularly beneficial for beginners who are learning the nuances of Haskell syntax and functional programming concepts, as it provides an immediate platform to type and evaluate Haskell expressions.
Explanation:
The command ghci
initializes the interactive shell. With no additional arguments or file specifications, it launches a blank REPL session, providing a prompt where you can type Haskell code and see instant results.
Example Output:
GHCi, version 8.10.4: https://www.haskell.org/ghc/ :? for help
Prelude>
Use case 2: Start a REPL and load the specified Haskell source file
Code:
ghci source_file.hs
Motivation: Loading a Haskell source file directly into GHCi is advantageous for developers working on larger projects or learning from code examples. This allows users to test and modify specific sections of a codebase incrementally, without having to compile the complete project each time.
Explanation:
In this use case, ghci source_file.hs
specifies that source_file.hs
is to be preloaded into the GHCi session. On initiation, the REPL environment will automatically parse and interpret the contents of the specified file, making its definitions (such as functions and types) available to the user at the prompt.
Example Output:
[1 of 1] Compiling Main ( source_file.hs, interpreted )
Ok, one module loaded.
*Main>
Use case 3: Start a REPL and enable a language option
Code:
ghci -XTypeFamilies
Motivation: GHCi supports numerous language extensions beyond Haskell’s standard features. For a developer exploring advanced language capabilities such as Type Families, enabling a specific language option directly from the command line streamlines the process of testing and learning those features.
Explanation:
The -X
flag is used to enable specific GHC language extensions in the GHCi session. Here, -XTypeFamilies
activates the Type Families extension, allowing you to utilize this feature during your interactive session. It modifies the default language configuration to incorporate these advanced features.
Example Output:
GHCi, version 8.10.4: https://www.haskell.org/ghc/ :? for help
Prelude> :set -XTypeFamilies
Prelude>
Use case 4: Start a REPL and enable some level of compiler warnings
Code:
ghci -Wall
Motivation: Compiler warnings can provide valuable insights into potential issues or inefficiencies in code. Enabling warnings during a GHCi session aids developers in catching common mistakes early in the development process by emphasizing places in their code that may contain bugs or bad practices.
Explanation:
The -W
flag in GHCi is followed by a warning level such as all
, which prompts the compiler to display warnings about potentially problematic code patterns. -Wall
activates comprehensive warnings, helping maintain code quality by encouraging best practices.
Example Output:
GHCi, version 8.10.4: https://www.haskell.org/ghc/ :? for help
Loaded package environment from /home/user/.ghc/x86_64-linux-8.10.4/environments/default
Prelude>
Use case 5: Start a REPL with a colon-separated list of directories for finding source files
Code:
ghci -i/home/user/haskell_projects:/home/user/libraries
Motivation: Projects are often spread across multiple directories, especially in collaborative environments or larger codebases. Specifying directories where source files are located allows GHCi to locate and load these files efficiently, streamlining the development workflow when working across different projects or modules.
Explanation:
The -i
flag, followed by a colon-separated list of directories, instructs GHCi to look for source files within the specified paths in addition to the current directory. This feature is vital for projects with complex structures that rely on multiple modules or external libraries.
Example Output:
GHCi, version 8.10.4: https://www.haskell.org/ghc/ :? for help
Prelude>
Conclusion:
The ghci
command is a versatile tool for Haskell development, facilitating everything from immediate code testing to exploring advanced language features. By tailoring its startup with various options, developers can enhance their productivity and streamline their coding processes in this powerful interactive environment. GHCi truly embodies the interactive spirit of functional programming by encouraging experimentation, exploration, and engagement with code in real-time.