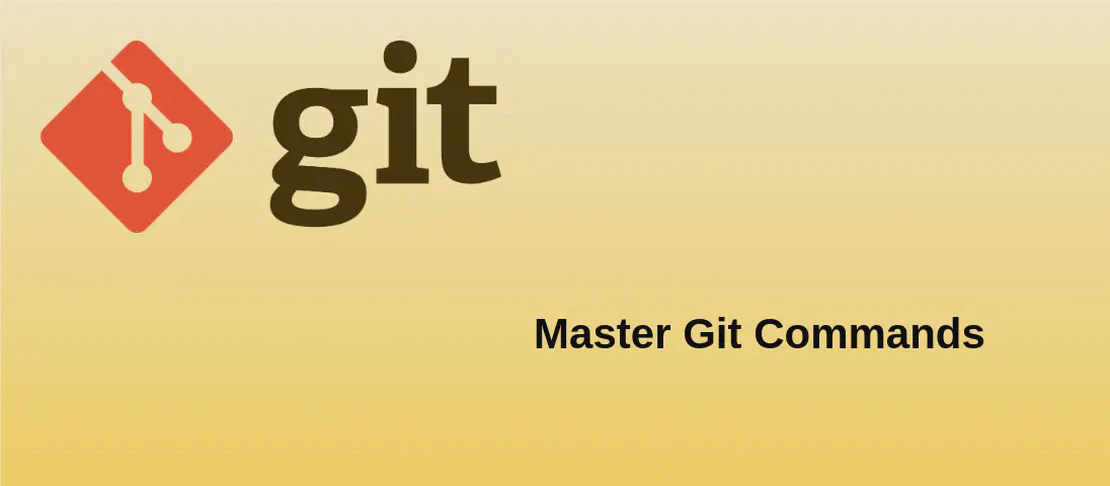
How to Use the Command 'git add' (with Examples)
The git add
command is fundamental in Git operations, allowing users to add changes in the working directory to the staging area. Before committing changes to the repository, using git add
helps you prepare and manage files that you want to include in your next commit. This command operates on a granular level, enabling users to specify individual files, tracked or untracked files, or even parts of files, providing tremendous flexibility and control over what’s included in a commit.
Use case 1: Add a file to the index
Code:
git add path/to/file
Motivation:
This command is used when you want to stage a specific file for a commit. For instance, you may be working on multiple files but only one is ready to be committed. By staging this file individually, you can ensure it is the only one included in the next commit, maintaining clean and organized commit history.
Explanation:
git add
: The base command to stage files for commit.path/to/file
: The specific path of the file you wish to add to the index.
Example Output:
(no output unless an error occurs; 'git status' will show the file as staged)
Use case 2: Add all files (tracked and untracked)
Code:
git add -A
Motivation:
This command is beneficial when you’ve made changes across multiple files, and you want to stage all of them at once, regardless of whether they were previously tracked by Git or are newly created.
Explanation:
git add
: Initiates the staging process.-A|--all
: This flag stages all changes in the directory, capturing both new and modified files.
Example Output:
(no output unless an error occurs; 'git status' will reflect all files as staged)
Use case 3: Add all files in the current folder
Code:
git add .
Motivation:
Using this command is convenient when you want to stage all modifications within the current working directory, especially useful in larger projects where you are focusing changes within a specific folder.
Explanation:
git add
: Commands Git to start staging..
: Refers to the current directory and all its contents, effectively staging all modified and untracked files within it.
Example Output:
(no output unless an error occurs; 'git status' will display all folder contents as staged)
Use case 4: Only add already tracked files
Code:
git add -u
Motivation:
This use case is significant when modifications have been made only to files that are already tracked by Git, and you want to avoid adding any untracked or new files to the staging area.
Explanation:
git add
: The command to stage files.-u|--update
: Stages changes only to files that are already part of the repository.
Example Output:
(no output unless an error occurs; 'git status' will show only tracked files as staged)
Use case 5: Also add ignored files
Code:
git add -f path/to/ignored-file
Motivation:
At times, specific files are ignored by default due to rules set in .gitignore
, but you might need to include them temporarily for a particular commit. This command allows you to override those ignore rules.
Explanation:
git add
: Staging command.-f|--force
: Overrides the.gitignore
rules to stage ignored files.path/to/ignored-file
: Specific file path that is normally ignored.
Example Output:
(no output unless an error occurs; 'git status' will list the ignored file as staged)
Use case 6: Interactively stage parts of files
Code:
git add -p
Motivation:
This interactive usage is suited for when you want to selectively stage portions of changes within a file. Ideal in scenarios involving partial completion of tasks within a file, allowing only specific chunks to be staged and committed.
Explanation:
git add
: The staging routine.-p|--patch
: Initiates an interactive process to selectively stage portions of file changes.
Example Output:
<Interactive session initiates showing a diff of changes with options to stage or skip sections>
Use case 7: Interactively stage parts of a given file
Code:
git add -p path/to/file
Motivation:
If you know exactly which file contains the changes you’d like to selectively stage, this command helps optimize the selection process directly within that particular file.
Explanation:
git add
: Command to start adding files.-p|--patch
: Enables interactive staging of file changes.path/to/file
: Targets a specific file to apply the patch process.
Example Output:
<Starts an interactive prompt towards only the specified file's changes>
Use case 8: Interactively stage a file
Code:
git add -i
Motivation:
An interactive way to navigate and review all unstaged changes, providing various options like staging, modifying, or reviewing status, thus enabling a comprehensive and hands-on approach to staging.
Explanation:
git add
: The foundational staging command.-i|--interactive
: Triggers an interactive interface for managing the staging process.
Example Output:
<Interactive interface appears, showing numbered options for each uncommitted changes>
Conclusion
The git add
command is a highly versatile tool essential for effectively managing which changes are included in a Git commit. By understanding and utilizing its various options and use cases, developers can exercise precise control over their project’s version history, ensuring accurate and meaningful commit messages. Whether you’re staging all changes at once or surgically selecting parts of a file, mastering git add
is a foundational skill for any developer working with version control.