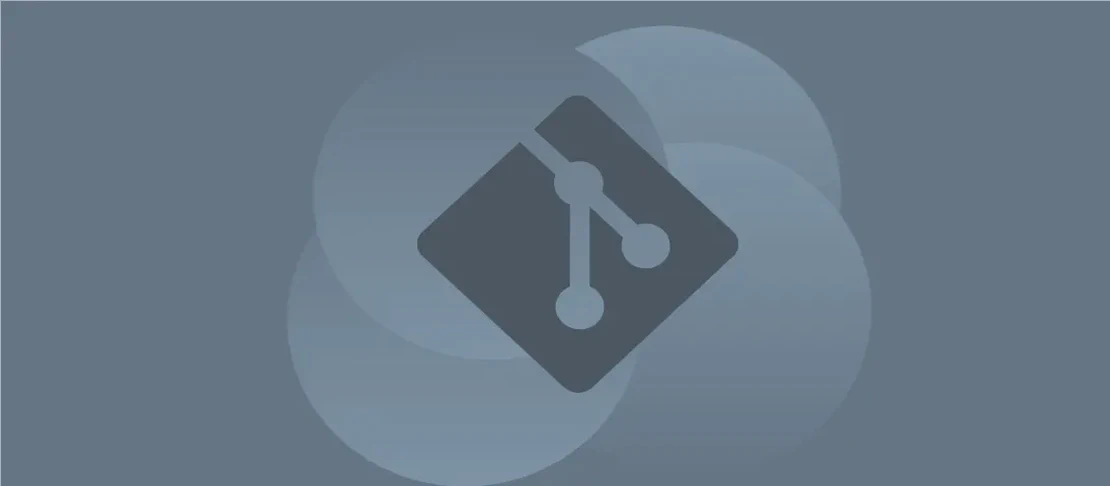
How to use the command 'git apply' (with examples)
The git apply
command is an essential tool in the Git ecosystem that allows developers to apply patches directly to files or the index without immediately creating a commit. This feature is particularly useful when you want to test changes or apply remote patches without altering the commit history right away. Unlike git am
, which also applies a patch but creates a commit, git apply
gives you the flexibility to control how and when a patch is committed. Let’s explore various usage scenarios of the git apply
command, each tailored for different practical situations that you may encounter during development.
Use case 1: Print messages about the patched files
Code:
git apply --verbose path/to/file
Motivation:
Using the --verbose
flag with git apply
can be incredibly useful for developers who need insight into which files have been affected by applying a patch. This can be especially beneficial in larger projects where multiple files are updated simultaneously, and understanding the scope of these changes is crucial for ensuring consistency and detecting unintended edits.
Explanation:
git apply
: The base command for applying patches.--verbose
: This flag instructs Git to print detailed messages about what is being done with the patch. It helps in logging the patch application process, which can be used for debugging or review purposes.path/to/file
: The path to the patch file you wish to apply.
Example output:
Checking patch somefile.txt...
Applied patch somefile.txt cleanly.
Use case 2: Apply and add the patched files to the index
Code:
git apply --index path/to/file
Motivation:
The --index
option is ideal when you want the changes to be staged for commit immediately after applying the patch. This is particularly useful in a workflow where you want to apply patches and prepare them for commit in one seamless step, thus reducing manual intervention and potential errors.
Explanation:
git apply
: The command initiates the patch application process.--index
: This argument updates the index (or staging area) with the patch’s changes. It sets the stage for these changes to be committed subsequently, maintaining a clean and organized workflow.path/to/file
: The path indicates which patch file contains the changes to be applied.
Example output:
Checking patch anotherfile.txt...
Applied patch anotherfile.txt cleanly and added to the index.
Use case 3: Apply a remote patch file
Code:
curl -L https://example.com/file.patch | git apply
Motivation:
In open-source projects or collaborative environments, you may need to apply patches hosted on remote servers. This technique allows you to apply a patch directly from a URL without manually downloading the file, streamlining the process when working with patch contributors or remote team members.
Explanation:
curl -L
: Here,curl
fetches files from URLs, with-L
ensuring that all redirects are followed.https://example.com/file.patch
: This URL points to the remote patch file that you intend to apply, illustrating flexibility in source options.| git apply
: This pipeline immediately pipes the patch file’s content into thegit apply
command, ready to modify the local codebase.
Example output:
Checking patch remotefile.txt...
Applied patch remotefile.txt cleanly.
Use case 4: Output diffstat for the input and apply the patch
Code:
git apply --stat --apply path/to/file
Motivation:
Diffstat provides a summarized view of changes introduced by a patch in terms of line modifications, offering a quick overview of added, removed, or modified lines across affected files. This is useful for developers looking to evaluate the scale of changes at a glance before integrating them into the project.
Explanation:
git apply
: Initiates the process of patch application.--stat
: Generates and displays a summary of changes made by the patch.--apply
: Ensures that after computing the diffstat, the patch is applied as well.path/to/file
: Specifies the path of the patch file you want to analyze and apply.
Example output:
somefile.txt | 10 +++++-----
1 file changed, 5 insertions(+), 5 deletions(-)
Use case 5: Apply the patch in reverse
Code:
git apply --reverse path/to/file
Motivation:
The ability to reverse a patch is key for undoing changes introduced by a previous patch, especially useful when a patch was applied mistakenly or if a regression testing reveals unforeseen bugs. This feature provides an easy mechanism to revert changes without extensive manual intervention.
Explanation:
git apply
: Begins the process for applying or reversing patches.--reverse
: Instructs Git to unapply the patch, effectively reverting the changes introduced by the patch file.path/to/file
: Specifies the patch file whose changes need to be reversed.
Example output:
Checking patch anotherfile.txt...
Reversed patch anotherfile.txt cleanly.
Use case 6: Store the patch result in the index without modifying the working tree
Code:
git apply --cache path/to/file
Motivation:
By using the --cache
option with git apply
, developers can stage the changes from a patch without altering the working tree. This aids in preserving the current state of the working directory while keeping the changes ready for future commit, a beneficial approach when working on new experimental changes that need staging without disruption.
Explanation:
git apply
: Activates the patch application protocol.--cache
: Stages changes to the index without altering the files in the working directory, allowing developers to maintain workspace continuity.path/to/file
: Points to the patch file whose changes are to be prepared for commit.
Example output:
Staged patch result for somefile.txt in the index without changing working tree.
Conclusion:
Incorporating git apply
into your workflow can significantly enhance your ability to manage patches effectively. Whether you’re staging changes, applying remote patches, reversing unintended changes, or simply wanting to review the impact of a patch before applying it, git apply
provides versatile options to best suit your development needs. Whether you are an individual developer or part of a team, mastering these use cases can streamline your code management processes.