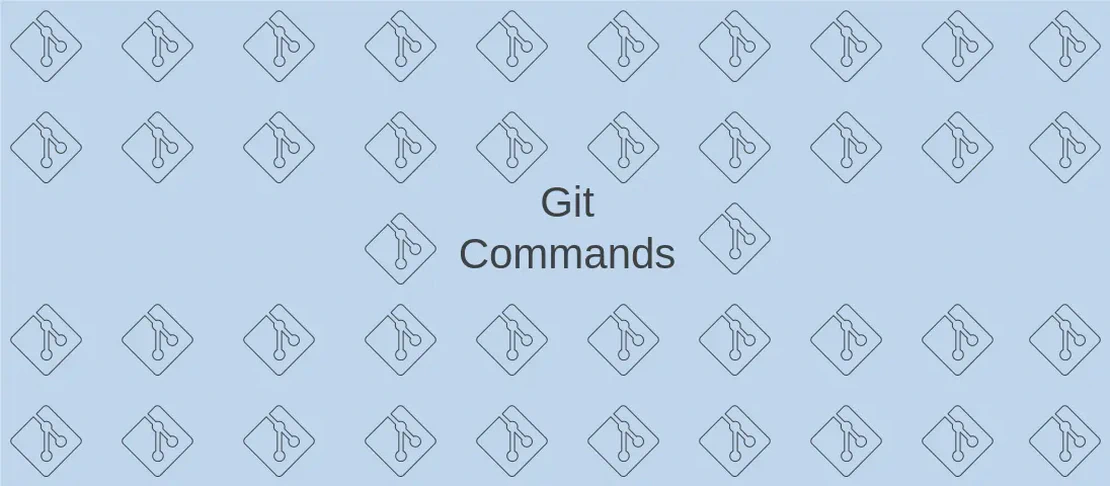
How to use the command 'git archive' (with examples)
The git archive
command is a powerful tool in the Git ecosystem, primarily used to create archived (compressed) versions of your project’s snapshot. It provides flexibility by enabling developers to produce archives in various formats, such as tar and zip, while also allowing customization of the archive’s contents, directory paths, and even compression levels. By encapsulating the repository’s state at a specific point, it supports easy transfer, backup, and distribution of the codebase.
Use case 1: Create a tar archive from the contents of the current HEAD and print it to stdout
Code:
git archive -v HEAD
Motivation:
In many scenarios, developers might want to create a quick and temporary archive to check its contents or pipe it into another command for further processing. By archiving the current commit state of the HEAD and printing it to stdout
, you can directly view or stream the archive without creating a file on the disk.
Explanation:
git archive
: The command used to create an archive.-v
or--verbose
: Provides more details about the progress and the files being archived, useful for confirming the command’s execution.HEAD
: Refers to the latest commit of the current branch, which serves as the basis for the archive.
Example Output:
This will provide an output within the terminal displaying the files included in the tar archive, but since it’s printed to stdout
, the content might show encoded data.
Use case 2: Use the Zip format and report progress verbosely
Code:
git archive -v --format zip HEAD
Motivation: Choosing a zip format can be crucial for interoperability across various platforms where tar files may not be supported natively or easily extractable. This use case is especially relevant when preparing code bundles for users on systems with limited tar support.
Explanation:
--format zip
: Specifies that the archive should be created in the .zip format, making it more accessible for Windows users or situations where only zip format is preferred.HEAD
: Like in the earlier example, this specifies the latest commit as the snapshot source for the archive.
Example Output:
The zip data stream is sent to stdout
, allowing you to redirect or store it using additional shell commands. This provides a verbose output to the console, indicating the progress and content of the operation.
Use case 3: Output the Zip archive to a specific file
Code:
git archive -v --output path/to/file.zip HEAD
Motivation:
Storing an archive in a specific location rather than printing it to stdout
is often required for distribution, backup, or ensuring that the archive is accessible later. When a definitive file is needed for automation scripts or distribution packages, this approach is ideal.
Explanation:
--output path/to/file.zip
: Directs the archive output to a file specified by the path, facilitating easier handling and manipulation after creation.HEAD
: The current HEAD is used again as the base of the archived project contents.
Example Output:
A file named file.zip
will be created at the specified path. This file is a zip archive containing all the files from the current state of the HEAD.
Use case 4: Create a tar archive from the contents of the latest commit of a specific branch
Code:
git archive --output path/to/file.tar branch_name
Motivation: Under collaboration or when splitting work into branches, consolidating the latest updates from a specific branch can be necessary. Whether it is for a release or testing, this command facilitates the process by archiving that branch’s latest state.
Explanation:
--output path/to/file.tar
: This option saves the archive directly to a tar file in the specified location.branch_name
: Instead of using HEAD, it directs to a specific branch, providing the flexibility to choose the source state.
Example Output:
A tar archive, file.tar
, is created, encapsulating the files from the named branch’s latest commit.
Use case 5: Use the contents of a specific directory
Code:
git archive --output path/to/file.tar HEAD:path/to/directory
Motivation: Sometimes, archiving the entire repository is unnecessary, and you only need a specific subdirectory. This is particularly useful for compartmentalized projects or when distributing only a subset of the project’s components.
Explanation:
--output path/to/file.tar
: Specifies where the completed tar archive should be saved.HEAD:path/to/directory
: Points the archive to only envelop the contents of the specified directory within the current HEAD.
Example Output:
The resultant tar file, file.tar
, includes only the files located in path/to/directory
.
Use case 6: Prepend a path to each file to archive it inside a specific directory
Code:
git archive --output path/to/file.tar --prefix path/to/prepend/ HEAD
Motivation: When extracting an archive, having the files contained within a parent directory is neat and prevents overlap with the existing filesystem structure. This use case ensures that all the archived contents are prefixed, making them tidy upon extraction.
Explanation:
--output path/to/file.tar
: Designates where to create the tar archive.--prefix path/to/prepend/
: Adds the specified path as a prefix inside the archive, sorting contents under this directory.HEAD
: Reflects the repository’s current state to form the archive base.
Example Output:
The tar archive file.tar
will have its contents organized under path/to/prepend/
, with all internal paths prepended accordingly.
Conclusion:
The git archive
command offers a versatile and efficient way of handling project snapshots by providing different formats and options to customize archive output. Its use cases extend from quickly generating snapshots to preparing distribution-ready packages, making it a valuable tool for developers needing precise control over their project’s archival output.