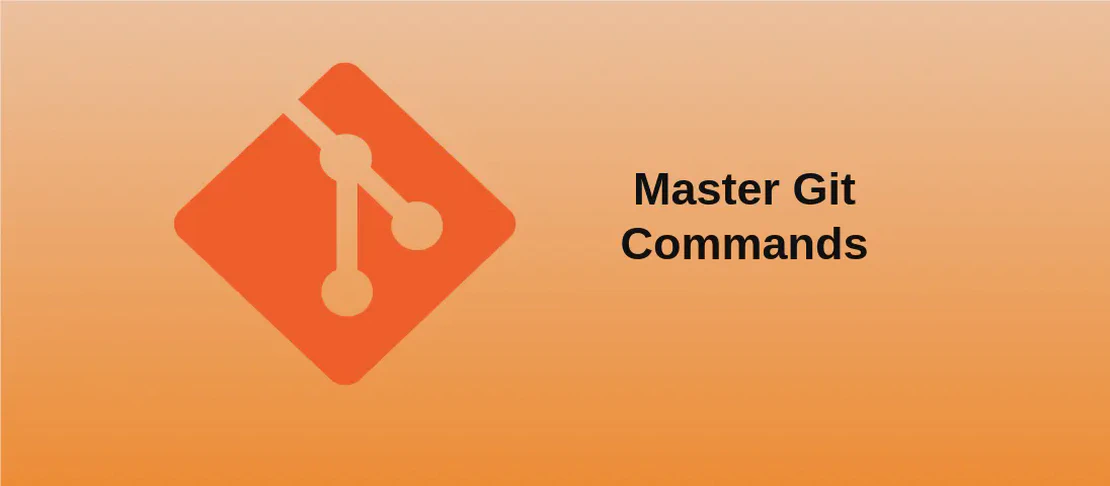
How to use the command git bisect (with examples)
Git bisect is a command in Git that allows users to perform a binary search to find the commit that introduced a bug. It automatically jumps back and forth in the commit graph to progressively narrow down the faulty commit.
Use case 1: Start a bisect session on a commit range
Code:
git bisect start bad_commit good_commit
Motivation: This use case is used to start a bisect session on a commit range bounded by a known buggy commit and a known clean commit. By specifying these two commits, git bisect can perform a binary search within this range to find the faulty commit.
Explanation:
bad_commit
: The commit that introduced the bug.good_commit
: A clean commit, typically older than thebad_commit
.
Example output:
Bisecting: 25 revisions left to test after this (roughly 5 steps)
[87ad6c2f567] Add new feature
Use case 2: Mark a commit as “bad” or “good” after testing
Code:
git bisect good|bad
Motivation: After each commit is selected by git bisect
during the binary search, it needs to be tested for the presence or absence of the bug. Based on the result of the test, the commit is marked as either “bad” or “good” to narrow down the range further.
Explanation:
good
: Mark the selected commit as good, indicating that the bug is not present.bad
: Mark the selected commit as bad, indicating that the bug is present.
Example output:
Bisecting: 12 revisions left to test after this (roughly 4 steps)
[9a72b38ef24] Fix issue with data validation
Use case 3: End a bisect session and return to the previous branch
Code:
git bisect reset
Motivation: After git bisect
pinpoints the faulty commit, it is necessary to end the bisect session and return to the previous branch. This allows further investigation or fixing of the bug.
Explanation:
- This command ends the bisect session and resets the repository to the state it was in before the session began.
Example output: No output, the session is ended and the repository is reset.
Use case 4: Skip a commit during a bisect
Code:
git bisect skip
Motivation: Sometimes, during a bisect session, a commit may be encountered that fails the tests due to a different issue. In such cases, it is necessary to skip that commit to ensure an accurate search for the faulty commit.
Explanation:
- This command instructs
git bisect
to skip the current selected commit and proceed to the next one.
Example output:
Bisecting: 6 revisions left to test after this (roughly 3 steps)
[5622998dcf1] Refactor code structure
Use case 5: Display the log of bisect session
Code:
git bisect log
Motivation: During a bisect session, it can be helpful to view a log of what has been done so far. The log provides a summary of the steps taken by git bisect
and the marked commits.
Explanation:
- This command displays the log of the bisect session, including the marked commits, skipped commits, and other relevant information.
Example output:
git bisect start
# bad: [bad_commit] Implement new feature
git bisect bad fd66cc758171d241b21e1a93d9a396061f6b2ced
# good: [good_commit] Initial commit
git bisect good a924b60ab5c496b24d5b4c946390d786f2e4fe96
Conclusion:
The git bisect
command is a powerful tool for locating the commit that introduced a bug. By carefully selecting and marking commits during a bisect session, developers can efficiently narrow down the faulty commit and address the issue.