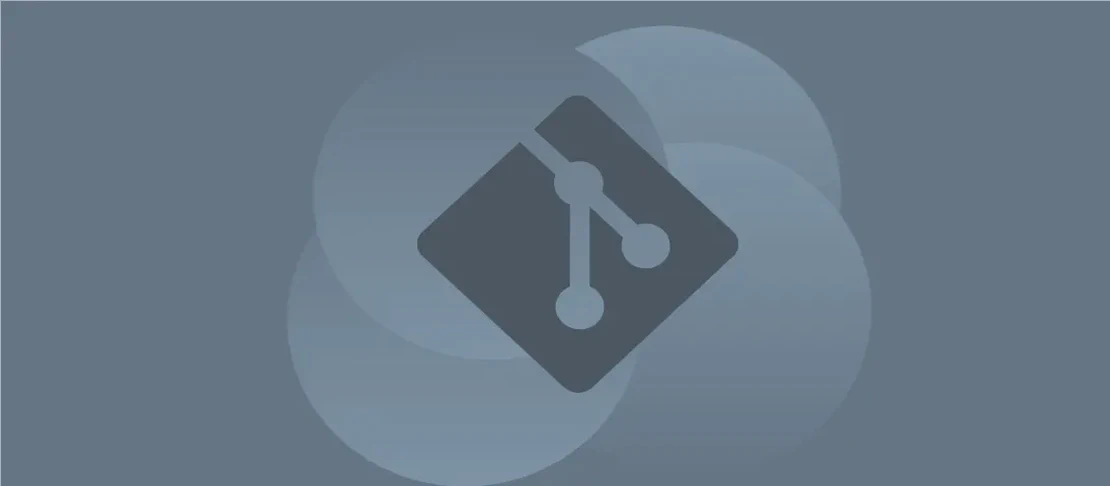
How to Use the Command 'git blame' (with Examples)
git blame
is a powerful tool in the Git version control system that assigns responsibility for each line within a file by showing which user last modified a specific line of code, along with the commit hash. This command can be invaluable for understanding the history of a project, identifying who made a particular change, and tracing when a piece of code was altered. All of these can be essential when debugging or reviewing code changes over time.
Use Case 1: Print File with Author Name and Commit Hash on Each Line
Code:
git blame path/to/file
Motivation:
Using this command provides a clear and concise way to gain insights into the historical changes made to a specific file. This can be highly useful during code reviews or when trying to understand the evolution of a piece of code. Specifically, if a bug is identified, knowing who last modified each line can expedite communication with the right developers for potential fixes.
Explanation:
git blame
: This initiates the blame command in Git to show details of file modifications.path/to/file
: This is the path to the target file within the repository, for which you want to view the line-by-line change history.
Example Output:
a1b2c3d4 (Alice Ferguson 2023-09-15 10:01:00 +0000 1) def function():
e5f6g7h8 (Bob Marley 2023-09-16 11:18:22 +0000 2) print("Hello, World!")
d9e0f1g2 (Alice Ferguson 2023-09-17 09:12:11 +0000 3) return True
In this example output, each line of the file is prefixed with the commit hash, author name, and date of the last modification. This can help in identifying when and by whom changes were made.
Use Case 2: Print File with Author Email and Commit Hash on Each Line
Code:
git blame -e path/to/file
Motivation:
Displaying the email addresses of contributors alongside commits can be useful for projects that involve distributed teams or open-source communities, where user names might be ambiguous or duplicate. By getting an email address, it becomes easier to ensure accurate and timely communication regarding specific changes.
Explanation:
git blame -e
: The-e
flag is used to show the email address of the authors instead of just their name.path/to/file
: This parameter specifies the file whose history you need to investigate further.
Example Output:
a1b2c3d4 (alice@domain.com 2023-09-15 10:01:00 +0000 1) def function():
e5f6g7h8 (bobm@music.net 2023-09-16 11:18:22 +0000 2) print("Hello, World!")
d9e0f1g2 (alice@domain.com 2023-09-17 09:12:11 +0000 3) return True
In this case, the author’s email ensures more precise identification of contributors, aiding robust communication within globally distributed teams.
Use Case 3: Print File with Author Name and Commit Hash on Each Line at a Specific Commit
Code:
git blame commit path/to/file
Motivation:
Identifying line-by-line changes at a specific commit can be crucial during code reviews or historical investigation. It allows you to examine what the file looked like at a given commit and understand the modifications made up to that point, helping in understanding specific updates or reverts to the codebase.
Explanation:
git blame
: This begins the blame operation.commit
: The specific commit hash you want to investigate is provided here.path/to/file
: Indicates the file of interest.
Example Output:
a1b2c3d4 (Alice Ferguson 2023-09-15 10:01:00 +0000 1) def function():
b3c4d5e6 (Carol Johnson 2023-09-14 14:28:37 +0000 2) print("Goodbye!")
d9e0f1g2 (Alice Ferguson 2023-09-17 09:12:11 +0000 3) return True
Here, the file’s state is displayed at a specific commit, showcasing lines as they were at that point in the project’s history.
Use Case 4: Print File with Author Name and Commit Hash on Each Line Before a Specific Commit
Code:
git blame commit~ path/to/file
Motivation:
Understanding the state of a file just before a particular commit can be critical when identifying a bug introduced after that commit. This comparison helps developers quickly pinpoint changes that might have caused any regression in the codebase, facilitating an effective debug process.
Explanation:
git blame
: This starts the blame operation.commit~
: This indicates the commit immediately before the provided commit hash.path/to/file
: The specific file being investigated for its previous state.
Example Output:
h3i4j5k6 (David Brown 2023-09-10 08:45:09 +0000 1) def function():
l7m8n9o0 (Elaine Benes 2023-09-11 09:22:47 +0000 2) print("Hi there!")
d9e0f1g2 (Alice Ferguson 2023-09-17 09:12:11 +0000 3) return True
Here, the output illustrates the file’s state before the specific commit, showing how it contributed to the eventual state seen after the commit.
Conclusion
The git blame
command is exceptionally useful for exploring the detailed history of a file in a Git repository. By identifying who touched each line and when, developers can gain invaluable insights into the evolution of the code, which can aid in both debugging and collaboration efforts. With different options available, git blame
can be tailored to show names, emails, and specific points in time, giving developers the flexibility to adapt it to their specific needs.