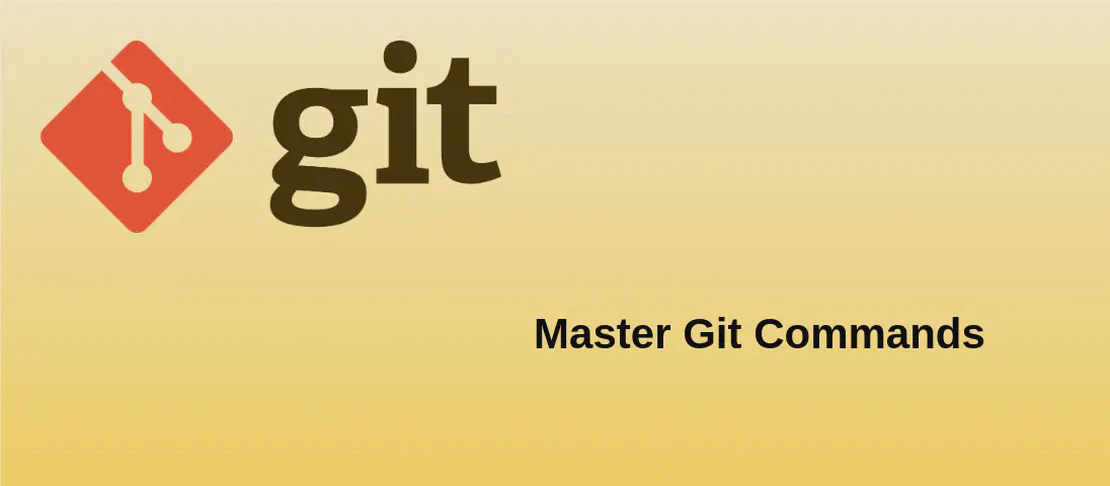
How to use the command 'git branch' (with examples)
Git branches allow you to work on different versions of your project simultaneously. The git branch
command is the main command in Git for working with branches. It can be used to manage branches, display information about branches, and switch between branches.
Use case 1: List all branches (local and remote; the current branch is highlighted by *
):
Code:
git branch --all
Motivation: This command is useful when you want to see a list of all branches in your project, including both the local and remote branches. The current branch is highlighted by the *
symbol. This can help you keep track of the branches in your project and determine which branch you are currently working on.
Explanation: The --all
option is used to include both local and remote branches in the output. It displays the list of branches in the repository along with the commit SHA hash and the branch name. The branch name with *
indicates the current branch.
Example output:
* main
feature-branch
remotes/origin/main
remotes/origin/feature-branch
Use case 2: List which branches include a specific Git commit in their history:
Code:
git branch --all --contains commit_hash
Motivation: This command is helpful when you want to identify which branches contain a specific Git commit. It allows you to easily track which branches are affected by a particular change and helps with code review and merging decisions.
Explanation: The --contains
option is used to filter the branches that include the specified Git commit in their commit history. You need to replace commit_hash
with the actual SHA hash of the commit you want to search for.
Example output:
main
* feature-branch
remotes/origin/main
remotes/origin/feature-branch
Use case 3: Show the name of the current branch:
Code:
git branch --show-current
Motivation: This command is useful when you want to quickly identify the name of the branch you are currently working on. It can be especially handy when scripting or automating tasks that require the current branch name.
Explanation: The --show-current
option is used to only show the name of the current branch. It provides a clean output with just the branch name.
Example output:
feature-branch
Use case 4: Create new branch based on the current commit:
Code:
git branch branch_name
Motivation: This command allows you to create a new branch based on the current commit. It is useful when you want to work on a new feature or bug fix without modifying the existing branch you are currently on.
Explanation: The branch_name
argument specifies the name of the new branch. The command creates a new branch at the same commit the current branch is pointing to.
Example output:
No output is displayed, the branch is created silently.
Use case 5: Create new branch based on a specific commit:
Code:
git branch branch_name commit_hash
Motivation: In some cases, you may want to create a new branch at a specific commit rather than the current commit. This command allows you to create a new branch based on a specific commit in the Git history.
Explanation: The branch_name
argument specifies the name of the new branch. The commit_hash
argument is the SHA hash of the commit you want to base the new branch on. The command creates a new branch at the specified commit.
Example output:
No output is displayed, the branch is created silently.
Use case 6: Rename a branch (must not have it checked out to do this):
Code:
git branch -m old_branch_name new_branch_name
Motivation: Sometimes, you may need to rename a branch to better reflect its purpose or to follow a naming convention. This command allows you to rename a branch, but you must not have the branch checked out when performing this operation.
Explanation: The -m
option is used to rename the branch. The old_branch_name
argument specifies the current name of the branch, and the new_branch_name
argument specifies the new name of the branch.
Example output:
No output is displayed, the branch is renamed silently.
Use case 7: Delete a local branch (must not have it checked out to do this):
Code:
git branch -d branch_name
Motivation: When you have finished working on a branch and no longer need it, you can delete it to keep your Git repository clean and organized. This command allows you to delete a local branch, but you must not have the branch checked out when performing this operation.
Explanation: The -d
option is used to delete the branch. The branch_name
argument specifies the name of the branch to be deleted.
Example output:
No output is displayed, the branch is deleted silently.
Use case 8: Delete a remote branch:
Code:
git push remote_name --delete remote_branch_name
Motivation: When you have pushed a branch to a remote repository and no longer need it, you can delete the remote branch to remove it from the remote repository.
Explanation: The push
command with the --delete
option is used to delete a remote branch. The remote_name
argument specifies the name of the remote repository, and the remote_branch_name
argument specifies the name of the branch to be deleted from the remote repository.
Example output:
To https://github.com/example/repo.git
- [deleted] remote_branch_name
Conclusion:
The git branch
command is a versatile command that allows you to manage branches, display information about branches, and switch between branches in Git. Understanding the various use cases and options of this command can greatly help in managing your project’s branches effectively.