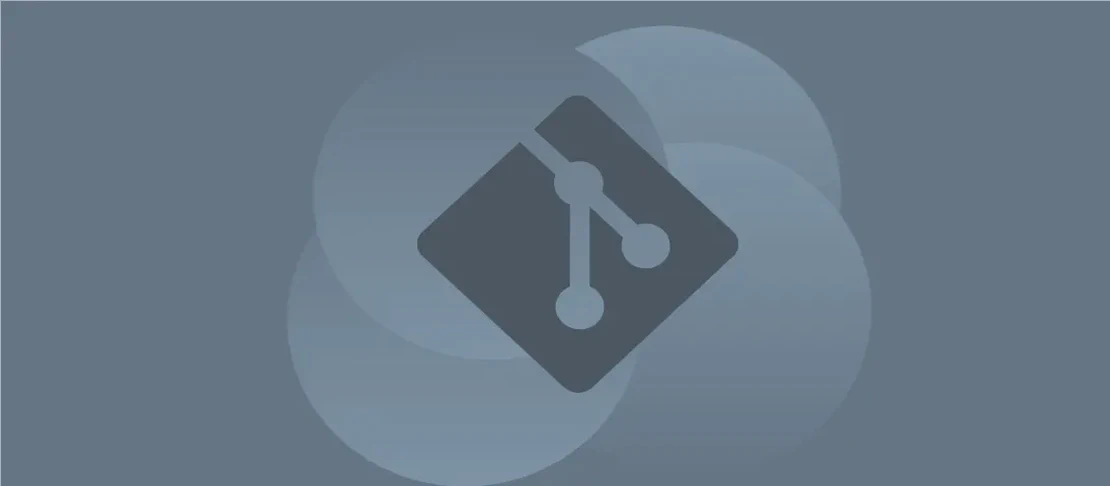
Harnessing 'git bulk' for Efficient Multi-Repo Management (with examples)
The git bulk
command is a part of the git-extras
suite and is designed to streamline operations across multiple Git repositories. It offers a powerful way to manage various Git tasks concurrently, saving time and reducing the complexity associated with handling multiple repositories individually. This command is especially useful in large-scale projects involving multiple interconnected repositories.
Use case 1: Register the Current Directory as a Workspace
Code:
git bulk --addcurrent workspace_name
Motivation:
Registering the current directory as a workspace simplifies organizing and executing batch operations on multiple repositories housed within. This use case is particularly helpful when you frequently work with a group of Git repositories situated in the same directory, allowing for seamless and efficient batch processing.
Explanation:
--addcurrent
: This option tellsgit bulk
to register the current directory as a workspace. It works by marking the current directory, thereby enabling batch operations within this workspace.workspace_name
: This is the name given to the workspace for easy identification and access. Naming workspaces helps keep your projects organized and easily accessible.
Example Output:
Successfully registered current directory as workspace 'workspace_name'.
Use case 2: Register a Workspace for Bulk Operations
Code:
git bulk --addworkspace workspace_name /absolute/path/to/repository
Motivation:
This use case is essential when you wish to perform bulk operations across repositories located in various directories. By specifying an absolute path, you ensure that the repositories are correctly located and batch operations are executed smoothly.
Explanation:
--addworkspace
: This flag instructsgit bulk
to add a new workspace using the provided path.workspace_name
: As earlier, this designates the workspace for future reference and commands./absolute/path/to/repository
: This specifies the exact file system location of the repository, ensuring precise actions on the desired repositories.
Example Output:
Workspace 'workspace_name' successfully registered for path '/absolute/path/to/repository'.
Use case 3: Clone a Repository Inside a Specific Directory, Then Register the Repository as a Workspace
Code:
git bulk --addworkspace workspace_name /absolute/path/to/parent_directory --from remote_repository_location
Motivation:
Cloning and registering repositories simultaneously is incredibly efficient for initializing new projects or synchronizing changes across multiple repositories. This use case is ideal for developers onboarding new projects with multiple repositories.
Explanation:
--addworkspace
: Initializes a new workspace as described in previous cases.workspace_name
: Label for the newly created workspace./absolute/path/to/parent_directory
: Specifies the parent directory where the repository will be cloned.--from
: Indicates the remote source location from which the repository should be cloned.remote_repository_location
: Provides the exact URL or path to the Git repository to be cloned.
Example Output:
Cloned repository from 'remote_repository_location' to '/absolute/path/to/parent_directory' and registered as workspace 'workspace_name'.
Use case 4: Clone Repositories from a Newline-Separated List of Remote Locations, Then Register Them as Workspaces
Code:
git bulk --addworkspace workspace_name /path/to/root/directory --from /path/to/file
Motivation:
Automating the cloning and registration of multiple repositories using a list simplifies bulk operations and project management tasks, making it indispensable for managing large development environments or setting up resources for teams.
Explanation:
--addworkspace
: Adds a new workspace, facilitating batch processing.workspace_name
: Identifier for the workspace./path/to/root/directory
: Destination directory for all the cloned repositories.--from
: Directs the command to read remote locations from a specified file./path/to/file
: Path to a file containing a newline-separated list of repository URLs to be cloned.
Example Output:
Cloned repositories listed in '/path/to/file' to '/path/to/root/directory' and registered as workspaces under 'workspace_name'.
Use case 5: List All Registered Workspaces
Code:
git bulk --listall
Motivation:
Listing all registered workspaces provides a quick overview of your current setup, ensuring you can track which directories are being managed and facilitating easier navigation and batch command execution across different projects.
Explanation:
--listall
: This flag requests a list of all currently registered workspaces, displaying their names and paths.
Example Output:
Workspace: workspace_name_1 at '/path/to/repo_1'
Workspace: workspace_name_2 at '/path/to/repo_2'
Use case 6: Run a Git Command on the Repositories of the Current Workspace
Code:
git bulk command command_arguments
Motivation:
Executing a Git command across multiple repositories in a workspace automates repetitive tasks, like updating branches or pulling the latest changes, enhancing productivity by reducing manual intervention.
Explanation:
command
: This placeholder represents the Git command you wish to execute.command_arguments
: These are any additional arguments that the command might require.
Example Output:
Executed 'command' with arguments 'command_arguments' on all repositories in the workspace.
Use case 7: Remove a Specific Workspace
Code:
git bulk --removeworkspace workspace_name
Motivation:
Removing obsolete or unnecessary workspaces declutters your configuration and ensures that only actively managed repositories are kept for efficient workflow and organization.
Explanation:
--removeworkspace
: Command used to delete a specified workspace.workspace_name
: Name of the workspace you wish to remove, ensuring targeted removal.
Example Output:
Workspace 'workspace_name' has been removed successfully.
Use case 8: Remove All Workspaces
Code:
git bulk --purge
Motivation:
Purging all workspaces is useful during significant project transitions, like a major pivot or organizational change that necessitates a complete restructuring or reinitialization of workspaces.
Explanation:
--purge
: A powerful command that deletes all currently registered workspaces.
Example Output:
All workspaces have been purged.
Conclusion:
The git bulk
command is an invaluable tool in the arsenal of any developer or team managing multiple Git repositories. By facilitating batch operations and offering efficient workspace management, it greatly enhances productivity and streamlines multi-repo project workflows. Whether you’re setting up new environments, maintaining multiple projects, or looking to automate routine commands, git bulk
provides the strategies and functionalities you need.