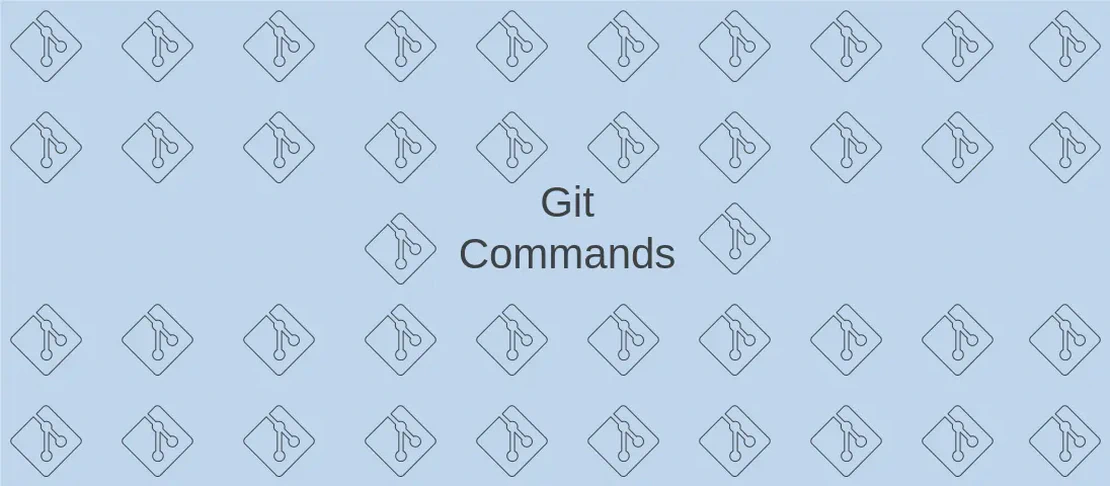
How to use the command 'git bundle' (with examples)
Git is a powerful and versatile version control system, and one of its lesser-known yet incredibly useful commands is git bundle
. This command allows you to package objects and references in a repository into an archived file, known as a bundle. Bundles can be used for transferring repositories, backing up data, or sharing code between developers in environments where direct network connectivity isn’t possible. Bundles are not only compact but also versatile, offering various ways to create and use them. Below are examples of how to effectively use the git bundle
command.
Use case 1: Create a bundle file that contains all objects and references of a specific branch
Code:
git bundle create path/to/file.bundle branch_name
Motivation:
Creating a bundle file for a specific branch is particularly helpful when you want to share that branch with another developer or team without providing full repository access. It can be a secure and efficient way to transfer only needed code and its history.
Explanation:
git bundle create
: This initializes the creation of a bundle file.path/to/file.bundle
: Specifies the filesystem path where the bundle file will be created.branch_name
: Indicates the specific branch to include in the bundle.
Example Output:
Counting objects: 100, done.
Writing objects: 100, 8.61 KiB, done.
Total 100 (delta 0), reused 0 (delta 0)
Use case 2: Create a bundle file of all branches
Code:
git bundle create path/to/file.bundle --all
Motivation:
When you need to create an offline backup that captures the entire state of your repository or share an entire repository with others, bundling all branches can be the solution. This ensures that all current branches and their histories are preserved and transferrable.
Explanation:
--all
: This flag includes every branch in the repository, ensuring you capture all the branch data.
Example Output:
Counting objects: 200, done.
Writing objects: 200, 12.34 KiB, done.
Total 200 (delta 0), reused 0 (delta 0)
Use case 3: Create a bundle file of the last 5 commits of the current branch
Code:
git bundle create path/to/file.bundle -5 HEAD
Motivation:
In instances where you want to compress just the recent work on a branch, creating a bundle of the last few commits can be potent. It’s ideal for sending your latest changes without unnecessarily large files.
Explanation:
-5
: Specifies that the bundle should only include the last five commits.HEAD
: Refers to the tip of the current branch.
Example Output:
Counting objects: 35, done.
Writing objects: 35, 3.21 KiB, done.
Total 35 (delta 0), reused 0 (delta 0)
Use case 4: Create a bundle file of the latest 7 days
Code:
git bundle create path/to/file.bundle --since 7.days HEAD
Motivation:
This use case is beneficial for capturing work done in a defined time frame, such as weekly progress reports. It helps in tracking and transferring the code changes made over recent days.
Explanation:
--since 7.days
: This filter only includes commits made in the last seven days.HEAD
: Indicates the reference point for the commits on the current branch.
Example Output:
Counting objects: 25, done.
Writing objects: 25, 2.07 KiB, done.
Total 25 (delta 0), reused 0 (delta 0)
Use case 5: Verify that a bundle file is valid and can be applied to the current repository
Code:
git bundle verify path/to/file.bundle
Motivation:
Before applying a bundle to a repository, it’s crucial to ensure the bundle’s integrity and correctness. Verifying a bundle ensures no corruption occurred during its creation or transfer.
Explanation:
verify
: This command checks the validity of the specified bundle.path/to/file.bundle
: The path of the bundle file to verify.
Example Output:
The bundle contains these 1 ref
5678abcdef branch_name
The bundle record is valid.
Use case 6: Print to stdout the list of references contained in a bundle
Code:
git bundle unbundle path/to/file.bundle
Motivation:
Before applying a bundle or when auditing what a bundle contains, listing its references can clarify what branches or commits are included and help avoid mistakes.
Explanation:
unbundle
: Used here to output the list of references in the bundle.path/to/file.bundle
: Specifies the bundle file to inspect.
Example Output:
unbundling refs/heads/branch_name
The bundle file contains 1 ref:
5678abcdef branch_name
Use case 7: Unbundle a specific branch from a bundle file into the current repository
Code:
git pull path/to/file.bundle branch_name
Motivation:
When you’ve received a bundle and want to integrate those changes into your existing repository, pulling a specific branch makes it easy to incorporate that code seamlessly.
Explanation:
pull
: Fetches the branches and integrates (merges) them into the current branch.path/to/file.bundle
: The bundle file to pull from.branch_name
: The branch to be pulled from the bundle.
Example Output:
From path/to/file.bundle
* branch_name -> FETCH_HEAD
Use case 8: Create a new repository from a bundle
Code:
git clone path/to/file.bundle
Motivation:
If you’re looking to recreate a repository from a bundle file, cloning enables you to initialize a new repository with the bundle’s contents, effectively restoring the repository to its original state as captured by the bundle.
Explanation:
clone
: Creates a new directory and imports all the contents of the bundle into this new repository.path/to/file.bundle
: The bundled archive to clone from.
Example Output:
Cloning into 'file'...
Receiving objects: 100%, done.
Resolving deltas: 100%, done.
Conclusion:
Utilizing git bundle
can provide significant benefits for repository management, from efficient data transfer to secure backups and seamless code sharing. These examples outline its flexibility, showcasing scenarios from basic branch sharing to comprehensive repository reconstruction. With this knowledge, developers can leverage git bundle
to streamline their workflows in disconnected, secure, or backup contexts.