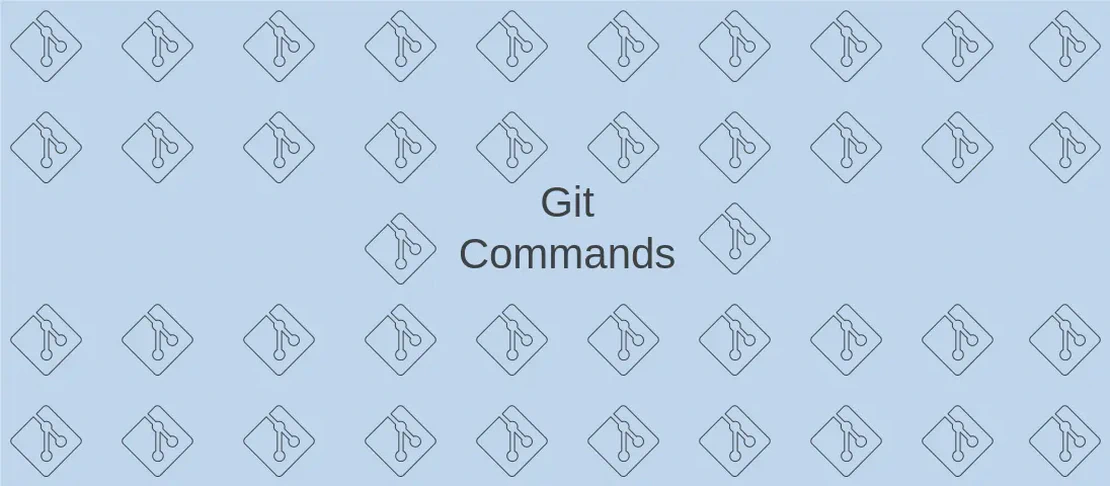
How to use the command 'git check-ignore' (with examples)
Git is a widely used version control system that allows developers to track changes in their codebase. The git check-ignore
command is a helpful tool for analyzing and debugging Git ignore/exclude (".gitignore") files. It allows you to check whether a file or directory is ignored based on the rules defined in the .gitignore
file. This article will provide examples of different use cases of the git check-ignore
command.
Use case 1: Check whether a file or directory is ignored
Code:
git check-ignore path/to/file_or_directory
Motivation: This use case is useful when you want to verify whether a specific file or directory is being ignored by Git. By checking if a file or directory is ignored, you can ensure that sensitive or generated files are not accidentally added to the repository.
Explanation: The git check-ignore
command is followed by the path to the file or directory you want to check. The command will analyze the .gitignore
rules and determine if the specified file or directory is ignored.
Example output:
.gitignore:3:path/to/file_or_directory
In the example output, .gitignore:3
specifies that the file or directory is ignored based on the rules defined in the .gitignore
file.
Use case 2: Check whether multiple files or directories are ignored
Code:
git check-ignore path/to/file path/to/directory
Motivation: In scenarios where you need to check multiple files or directories, this use case proves handy. It helps you quickly validate whether these items are being ignored by Git.
Explanation: The git check-ignore
command can accept multiple paths as arguments. Simply specify the paths of the files or directories you want to check, separated by spaces.
Example output:
.gitignore:2:path/to/file
.gitignore:3:path/to/directory
The example output shows that both “path/to/file” and “path/to/directory” are ignored according to the corresponding rules defined in the .gitignore
file.
Use case 3: Use pathnames from stdin
Code:
git check-ignore --stdin < path/to/file_list
Motivation: This use case becomes relevant when you have a list of file paths stored in a file, and you want to check their ignored status. By utilizing the --stdin
flag, you can process these paths directly from the standard input.
Explanation: The git check-ignore --stdin
command reads the list of file paths from the standard input instead of providing them as individual arguments. The file paths should be separated by newlines.
Example output:
.gitignore:1:path/to/file1
.gitignore:2:path/to/file2
.gitignore:3:path/to/file3
Here, each file path from the path/to/file_list
is checked against the .gitignore
rules, and the output displays the matching results.
Use case 4: Do not check the index
Code:
git check-ignore --no-index path/to/files_or_directories
Motivation: If you encounter a situation where you want to understand why certain paths are being tracked instead of being ignored, this use case is helpful. By excluding the index from the check, you can troubleshoot the reasons behind the tracking behavior.
Explanation: The git check-ignore --no-index
command instructs Git to skip the index while performing the check. The index is the staging area where changes are prepared before committing. By excluding the index, you can determine why certain files or directories are being tracked (not ignored).
Example output:
.gitignore:3:path/to/file_or_directory
The output shows that the specified path is being tracked because it does not match any rules in the .gitignore
file.
Use case 5: Include details about the matching pattern for each path
Code:
git check-ignore --verbose path/to/files_or_directories
Motivation: In certain cases, you may need to find out the specific rule in the .gitignore
file that causes a file or directory to be ignored. The --verbose
flag provides additional details about the matching patterns used.
Explanation: By using the --verbose
flag, the git check-ignore
command displays the pattern that matches each path being checked. This can help you understand which specific rule in the .gitignore
file applies to a particular file or directory.
Example output:
.gitignore:3:path/to/file_or_directory (example/*.txt)
The example output indicates that the specified path matches the pattern example/*.txt
defined in the .gitignore
file.
Conclusion
The git check-ignore
command is a valuable tool for analyzing and debugging Git ignore/exclude files. By understanding the various use cases provided in this article, you can effectively determine whether files or directories are ignored based on the rules defined in the .gitignore
file. This command helps ensure that your repository only tracks the necessary and desired files, while omitting any unwanted or sensitive files.