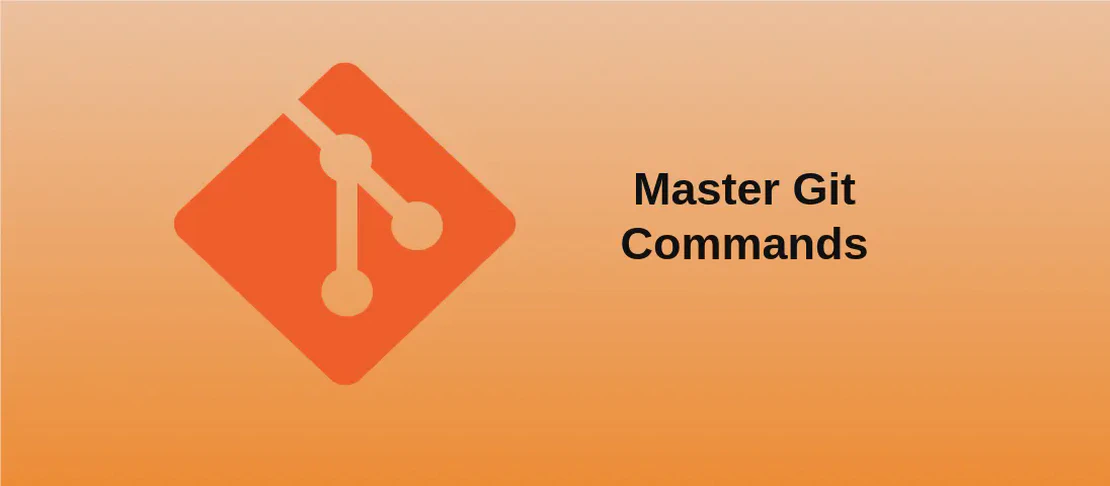
How to use the command 'git checkout' (with examples)
The git checkout
command is one of the most frequently used commands in Git workflows. It allows you to navigate between different branches, manage your working directory, and interact with specific file versions. Being able to efficiently execute these tasks is crucial for both solo development and collaborating on large codebases. This command can also be helpful for undoing changes, which is an invaluable part of version control.
Use case 1: Create and switch to a new branch
Code:
git checkout -b branch_name
Motivation:
This scenario is commonly encountered when you’re about to start work on a new feature, fix a bug, or test an experimental change without affecting the main project. Branches let you isolate your work into separate spaces, which is vital for maintaining a clean code history and simplifying the process of merging changes later.
Explanation:
git checkout
: The base command used to switch branches in Git.-b
: This flag tells Git to create a new branch before switching to it.branch_name
: This argument represents the name of the new branch you want to create. It’s a good idea to use descriptive names that denote the purpose of the branch, such asfeature/login
orbugfix/user-auth
.
Example output:
Switched to a new branch 'branch_name'
This output confirms that a new branch has been created and you are now working on it.
Use case 2: Create and switch to a new branch based on a specific reference
Code:
git checkout -b branch_name reference
Motivation:
There are instances where you want to base your new branch on a specific reference point, such as an existing branch, remote branch, or tag. This is useful if you want to start your work from a particular commit or align with someone else’s progress on a collaborative project.
Explanation:
git checkout
: The command used to switch branches or checkout paths in your working tree.-b
: Indicates that you want to create a new branch.branch_name
: Specifies the name of the new branch being created.reference
: This refers to the starting point for your new branch. This could be another branch, a specific commit hash, or a tag.
Example output:
Switched to a new branch 'branch_name'
This output indicates that the new branch has been created from the specified reference and you’ve successfully switched to it.
Use case 3: Switch to an existing local branch
Code:
git checkout branch_name
Motivation:
Switching to an existing branch is crucial when you need to continue working on different features or bugs that you’ve started previously. This allows you to seamlessly continue from where you left off without disturbing other branches and keeps your work organized.
Explanation:
git checkout
: The command for switching branches.branch_name
: Represents the name of the existing local branch you want to switch to.
Example output:
Switched to branch 'branch_name'
This output confirms that you have successfully navigated to the existing local branch.
Use case 4: Switch to the previously checked-out branch
Code:
git checkout -
Motivation:
This use case is particularly handy when you’re juggling multiple branches and need to return to the last branch you were working on. It saves time by allowing quick navigation back and forth between two branches without typing out full branch names.
Explanation:
git checkout
: The command to switch branches.-
: This serves as a shortcut to switch back to the previous branch you were on.
Example output:
Switched to branch 'previous_branch_name'
This confirms that you’ve switched back to the last branch you were on prior to your current one.
Use case 5: Switch to an existing remote branch
Code:
git checkout --track remote_name/branch_name
Motivation:
Collaborative workflows often involve pulling down branches from a remote repository. This allows you to track someone else’s branch, work in parallel, or review and test ongoing changes. Tracking a remote branch makes it easier to manage remote updates locally.
Explanation:
git checkout
: Command for switching branches.--track
: Instructs Git to set up a local branch that tracks the remote branch.remote_name/branch_name
: Indicates the remote repository and the specific branch you want to track.
Example output:
Branch 'branch_name' set up to track remote branch 'branch_name' from 'remote_name'.
Switched to a new branch 'branch_name'
This shows that you’ve set up tracking for the remote branch locally and switched to it.
Use case 6: Discard all unstaged changes in the current directory
Code:
git checkout .
Motivation:
Sometimes, you’ll make changes that you’re not happy with and want to revert your working directory to the last committed state. This could be useful for experimental changes that didn’t pan out or for quick reversals after realizing a mistake.
Explanation:
git checkout
: The primary command in use here..
: This indicates the current directory, signaling Git to discard all unstaged changes within it.
Example output:
(No output is returned for this command)
The lack of output signifies that all unstaged changes in the current directory have been undone.
Use case 7: Discard unstaged changes to a given file
Code:
git checkout path/to/file
Motivation:
While working on a project, you might decide to discard changes to a specific file without touching others. This is particularly useful when you’ve changed a file that was only part of a larger set of changes, and you realize you no longer need those specific modifications.
Explanation:
git checkout
: Command used for reverting changes.path/to/file
: Specifies the path to the file whose changes you want to discard.
Example output:
(No output is returned for this command)
Again, the absence of output indicates that the changes to the specified file have been reverted.
Use case 8: Replace a file in the current directory with the version of it committed in a given branch
Code:
git checkout branch_name -- path/to/file
Motivation:
Reverting a single file to its state from another branch can be crucial when you inadvertently introduce bugs and want to replace your changes with a known good version. It can also be helpful if you want to test changes from a different branch on your current setup.
Explanation:
git checkout
: This command is used to switch branches or revert file changes.branch_name
: Indicates the branch that holds the version of the file you want to use.--
: This separates the branch name from the path to the file, clarifying the command’s intention.path/to/file
: Represents the path to the file that you want to replace in your current directory.
Example output:
(No output is returned for this command)
Again, the lack of output suggests that the file has been successfully replaced with the version from the specified branch.
Conclusion:
The git checkout
command is indispensable within Git, providing flexibility in managing branches and variations in file versions. From creating and switching branches to reverting changes and managing remotes, mastering these use cases can significantly streamline your workflow, enabling efficient and organized version control practices.