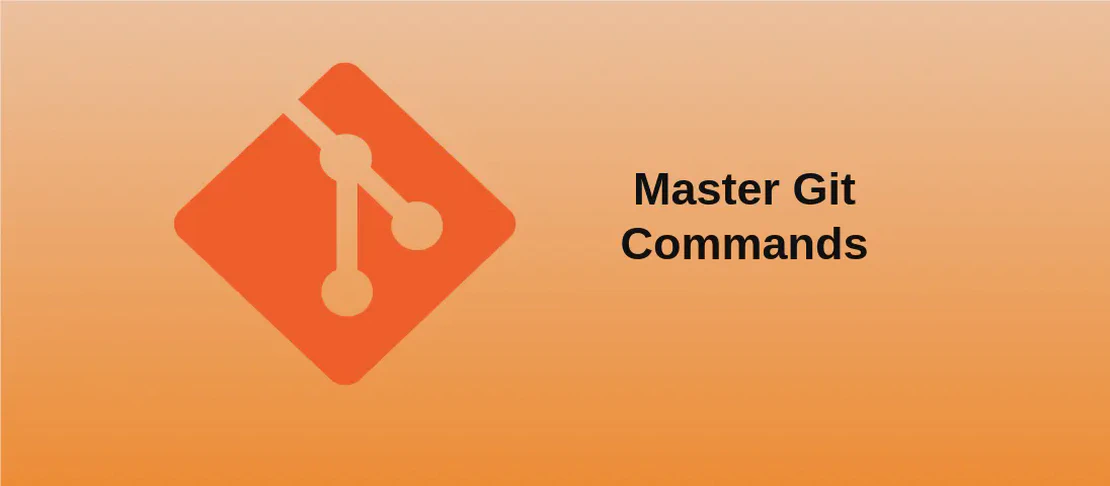
How to use the command 'git checkout-index' (with examples)
The ‘git checkout-index’ command is a somewhat lesser-known but powerful tool in the Git toolkit. It allows users to copy files from the index (staging area) to the working tree. This can be particularly useful for restoring deleted files, recovering lost changes, and exporting a specific snapshot of your working directory to another location. The command is versatile and offers various options to cater to different needs in version control and file management.
Use case 1: Restore any files deleted since the last commit
Code:
git checkout-index --all
Motivation:
Imagine you are working on a project and have accidentally deleted a few files that you have not yet committed. The file system crash or accidental directory deletion could leave your working tree lacking essential files. The command git checkout-index --all
can come to the rescue by restoring all files in the working tree that have been deleted. This ensures that you can continue your work without worrying about manually recovering each file.
Explanation:
git checkout-index
: This is the base command used to copy files from the index to the working tree.--all
: This option specifies that you want to operate on all files in the index. It is particularly useful if you are unsure about which files were deleted and don’t want to miss any file that might need recovery.
Example output:
Assuming the file example.txt
was deleted:
Restoring example.txt
Use case 2: Restore any files deleted or changed since the last commit
Code:
git checkout-index --all --force
Motivation:
In the course of software development, there are scenarios where changes in the working directory result in undesirable modifications or deletions, and you decide you want to revert to the last committed state. By executing git checkout-index --all --force
, you can swiftly revert any changes made to files since the last commit, including restoring any that have been deleted or altered. This ensures that your workspace is cleaned up and corresponds exactly to your last known working version.
Explanation:
git checkout-index
: The primary command for operating on files between the index and the working tree.--all
: Processes all files in the index. This helps in covering all occurrences of change or deletion in the working tree.--force
: This option forces the overwrite of any files in the working tree that differ from the index. It’s crucial when ensuring all changes are reverted without manual confirmation.
Example output:
For a modified example.txt
and a deleted another-file.txt
:
Restoring example.txt
Restoring another-file.txt
Use case 3: Restore any files changed since the last commit, ignoring any files that were deleted
Code:
git checkout-index --all --force --no-create
Motivation:
There are situations where you may want to restore only modified files to their state in the last commit but intentionally keep any files that have been deleted as such. This functionality can be vital when you’ve pruned unnecessary files from your directory but want to ensure other changes, perhaps bugs introduced inadvertently, are reverted safely.
Explanation:
git checkout-index
: The core utility that’s facilitating these file operations.--all
: Ensures all files in the index are considered, important when dealing with widespread changes.--force
: Overwrites changes in the working files to match those in the index.--no-create
: Specifies that new files should not be created in the working directory. This option deliberately bypasses the restoration of deleted files, which can be critical in maintaining a cleaned-up workspace.
Example output:
For a modified example.txt
but ignoring another-file.txt
deletion:
Restoring example.txt
Use case 4: Export a copy of the entire tree at the last commit to the specified directory
Code:
git checkout-index --all --force --prefix=path/to/export_directory/
Motivation:
Exporting the entire directory tree as it appeared at the last commit can be a crucial step for numerous purposes, such as creating a backup, deploying an exact snapshot, or providing a snapshot for code reviews. Using git checkout-index --all --force --prefix=path/to/export_directory/
, you can achieve this efficiently. It creates a structured archive in the specified location, using the commit as a reference, without affecting your existing working directory.
Explanation:
git checkout-index
: Establishes the process of copying files from the index to an alternative location.--all
: Collects every file present in the index.--force
: Ensures all existing files in the export directory will be overwritten.--prefix=path/to/export_directory/
: This sets a prefix path, meaning that it specifies where the files should be exported. The trailing slash is crucial as it denotes directory placement.
Example output:
For an export destination of output_directory/
:
Exporting file1.txt to output_directory/file1.txt
Exporting file2.txt to output_directory/file2.txt
....
Conclusion:
The git checkout-index
command is a powerful yet underutilized tool that can significantly aid in managing and recovering files within a repository. From restoring deleted files to exporting the project tree, its practical applications in rectifying mishaps and organizing file structures make it indispensable in the arsenal of advanced Git users. Each example discussed provides a tailored approach to specific file and directory issues, demonstrating the command’s versatility and capability.