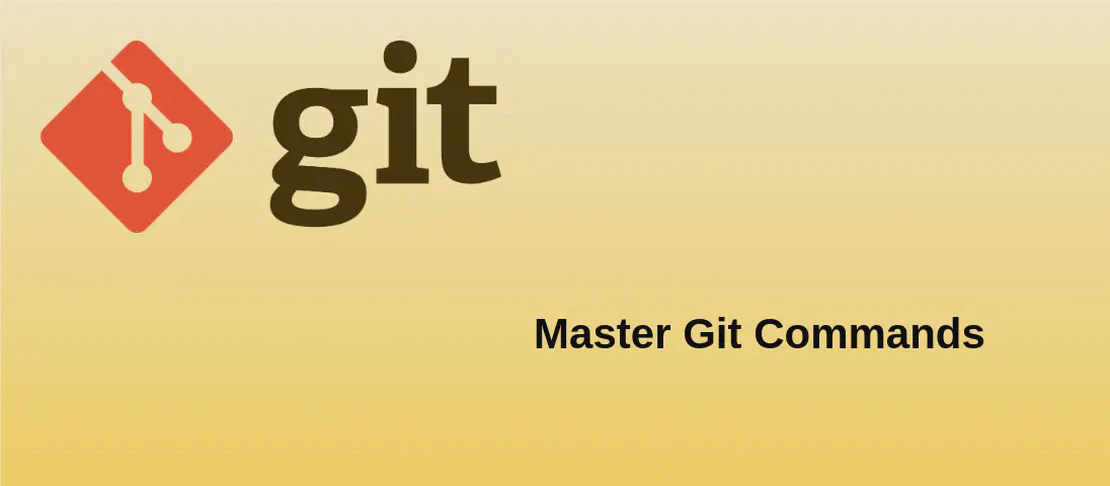
How to Use the Command 'git cherry-pick' (with examples)
The git cherry-pick
command is a versatile tool in Git that allows developers to apply changes introduced by existing commits onto the current branch. This feature is particularly useful when you want to extract and apply specific commits from one branch to another without merging entire branches. This can be done for various reasons, such as bug fixes or implementing specific features, without including unwanted changes. The power of this command lies in its flexibility to cherry-pick both single and multiple commits, as well as a range of commits, adapting to the developer’s specific needs in version control.
Apply a Commit to the Current Branch
Code:
git cherry-pick commit
Motivation:
Imagine you have a commit on a feature branch that fixes a critical bug. You want to apply this specific commit to the master branch, which is currently in production, without incorporating all the other alterations on the feature branch. This is where git cherry-pick
shines, allowing you to selectively apply just the bug fix.
Explanation:
git
: This invokes the Git version control system.cherry-pick
: This command tells git to cherry-pick the commit you specify.commit
: This refers to the hash or identifier of the specific commit whose changes you want to apply to the current branch.
Example Output:
The specified commit is applied on the current branch. If the cherry-picked commit has been successfully applied, you might see something like:
Finished one cherry-pick.
Apply a Range of Commits to the Current Branch
Code:
git cherry-pick start_commit~..end_commit
Motivation:
You have a series of sequential commits on a branch that collectively represent a complete feature or bug fix. If you want these commits to be applied to another branch without including non-related changes, you can cherry-pick the range of commits. This ensures that all necessary related changes are brought over effectively.
Explanation:
git
: Invoking Git as usual to carry out the operation.cherry-pick
: Again, specifies the command for cherry-picking.start_commit~..end_commit
: This specifies the range of commits fromstart_commit
toend_commit
. The~
denotes inclusion from the first parent ofstart_commit
up toend_commit
.
Example Output:
All the commits from the specified range are applied to the branch. You may see:
[cherry-pick] A number of commits added with messages indicating success.
Apply Multiple (Non-Sequential) Commits to the Current Branch
Code:
git cherry-pick commit1 commit2 ...
Motivation:
If you have various important changes spread across multiple commits that are not sequential, and you wish to apply these to the current branch, this ability to specifically select multiple non-sequential commits is ideal. This use case is particularly common when pulling in bug fixes from different parts of a feature branch.
Explanation:
git
: Standard invocation for Git.cherry-pick
: Initiating the cherry-pick process.commit1 commit2 ...
: A list of the specific commits by their unique identifiers that need to be applied. You can specify as many as you’d like.
Example Output:
Individual messages for each successful cherry-pick might appear:
Successfully cherry-picked commit1.
Successfully cherry-picked commit2.
...
Add the Changes of a Commit to the Working Directory, Without Creating a Commit
Code:
git cherry-pick --no-commit commit
Motivation:
Sometimes, you want to apply changes from a commit but have the flexibility to review or amend them before forming a new commit. Using this option allows you to introduce changes to your working directory without automatically committing them, which is beneficial for adjustments and optimizations.
Explanation:
git
: Calls Git to perform the operation.cherry-pick
: The main command.--no-commit
: This flag prevents creating a commit automatically with the applied changes.commit
: The identifier of the commit from which changes will be applied.
Example Output:
The changes will be present in the working directory, waiting for a commit:
Changes applied to working directory but not committed.
Conclusion
The git cherry-pick
command is a powerful tool in a developer’s arsenal for managing changes across branches. It provides the flexibility to selectively incorporate changes without disrupting the overall structure of your project or introducing unwanted code. Each use case presented highlights different scenarios where git cherry-pick
proves indispensable, from applying standalone fixes to grouping feature work, giving developers control over their branch management and integration strategies.