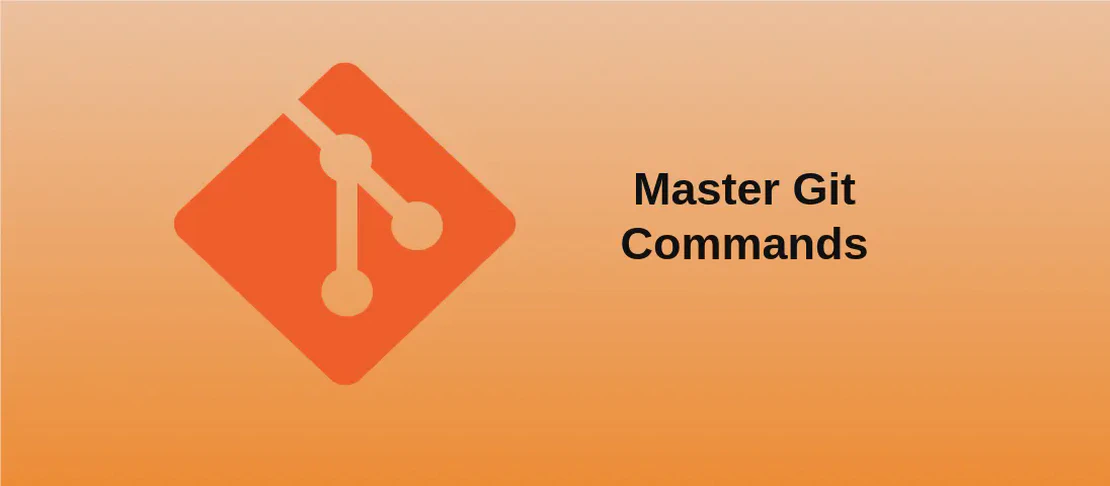
How to Use the Command 'git clean' (with examples)
The git clean
command is a useful utility in Git that allows developers to remove untracked files from their working directory. Untracked files are files that have not been added to the version control system and are not under Git’s tracking. This command helps to maintain a clean working directory by removing unnecessary files, and it is especially helpful when you want to reset your working environment to a known state.
Use case 1: Delete untracked files
Code:
git clean
Motivation: In the chaotic setup of a bustling project, a developer might find themselves with numerous temporary files, such as logs, test reports, or generated files, cluttering their workspace. These files, being untracked, do not contribute to version control. By using git clean
, one can swiftly remove these files, allowing for a more organized and efficient working directory conducive to productive development.
Explanation: The plain use of git clean
command triggers Git to prepare for cleaning operations — although, at this stage, it will not execute due to lack of enforcement. The command requires a force flag to actually delete the files, ensuring that files are not accidentally removed.
Example Output:
fatal: clean.requireForce defaults to true and neither -i, -n, nor -f given; refusing to clean
Use case 2: Interactively delete untracked files
Code:
git clean -i
Motivation: This approach is indispensable when developers are uncertain about the untracked files present in the directory or when they wish to meticulously manage which files to remove. The interactive mode invites users into a detailed selection process, ensuring that crucial files are not accidentally deleted, granting precision and control over the cleaning process.
Explanation: The -i
or --interactive
flag opens an interactive prompt within Git, allowing developers to selectively choose which files should be deleted. This is essential for projects where untracked files might play a vital role temporarily and discretion is necessary.
Example Output:
*** Commands ***
1: clean 2: filter by pattern 3: select by numbers
4: ask each 5: quit 6: help
What now>
Use case 3: Show which files would be deleted without actually deleting them
Code:
git clean --dry-run
Motivation: Before committing to a clean operation, developers may wish to understand the potential impacts and scope of the action. By simulating the cleanup process, this option presents an opportunity to review the list of untracked files, allowing for a safe and planned actual deletion later.
Explanation: The --dry-run
flag ensures that no actual cleaning occurs. Instead, it lists the files that would be deleted if clean was executed for real. This serves as an excellent precautionary measure to evaluate the consequences of cleaning operations.
Example Output:
Would remove temp.txt
Would remove logs/output.log
Use case 4: Forcefully delete untracked files
Code:
git clean -f
Motivation: Developers often encounter situations where the buildup of untracked files becomes substantial, affecting the performance and clarity of the workspace. This direct and forceful command initiates an immediate declutter of the repository without any prompt for confirmation, saving time for developers who are confident in their cleanup requirements.
Explanation: The -f
or --force
flag compels Git to proceed with the deletion of untracked files immediately, overriding the default preventions that require confirmation, thus streamlining the cleanup in confident scenarios.
Example Output:
Removing temp.txt
Removing logs/output.log
Use case 5: Forcefully delete untracked directories
Code:
git clean -f -d
Motivation: In dynamic projects, entire directories of untracked files can accumulate, such as when performing test compiles or storing build artifacts temporarily. When these directories are no longer needed, removing them along with their contents can simplify the project structure and free up space.
Explanation: The combination of -f
and -d
flags advises Git not only to delete untracked files forcefully but also to include directories in the deletion. This comprehensive approach ensures that no remnants are left behind, effectively purging untracked spaces in the repository.
Example Output:
Removing cache/
Removing tmp/
Use case 6: Delete untracked files, including excluded files
Code:
git clean -x
Motivation: Over time, developers might accumulate files that are typically ignored by Git via .gitignore
or .git/info/exclude
. Occasionally, it becomes necessary to override these exclusions during clean operations, either to achieve a thorough reset or address disk space concerns, ensuring no hidden or overlookable files linger.
Explanation: The -x
flag directs Git to override ignore settings and remove not just untracked files, but also those deemed unnecessary according to various ignore rules. This is particularly helpful for exhaustive cleaning sessions or before closing a project.
Example Output:
Removing .DS_Store
Removing node_modules/
Conclusion:
The git clean
command offers developers powerful options to manage their working directories, ensuring an efficient and organized environment for coding. By understanding and utilizing its different use cases, developers can maintain clarity and focus within their projects without the distractions of clutter and the potential risks of mismanaging vital files. Whether interacting, forcefully deleting, or including excluded files, these examples demonstrate a range of practical applications for keeping your Git repository as productive as it can be.