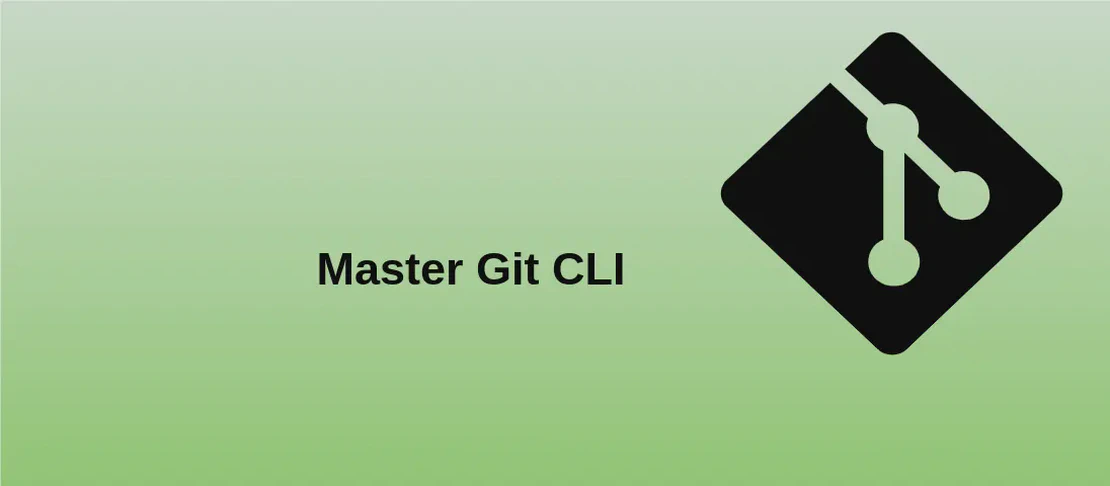
Git Clone: The Essential Guide (with examples)
Git is a powerful version control system that enables developers to collaborate on projects effectively. One of the fundamental operations in Git is the git clone
command. This command is essential for creating a local copy of a remote repository, setting the stage for offline work, code reviews, and collaboration. Whether you are working on open source projects or private repositories, mastering the git clone
command expands your ability to manage and manipulate codebases efficiently.
Use case 1: Clone an existing repository into a new directory
Code:
git clone remote_repository_location path/to/directory
Motivation:
When starting work on a project, you often need to have a local copy of the repository on your machine. By cloning an existing repository, you capture all its files, commit history, and branches. This use case is typically the first step before contributing to a codebase or conducting a comprehensive code review.
Explanation for every argument:
remote_repository_location
: This is the URL or path to the repository you want to clone. It can be an HTTP/HTTPS URL, an SSH URL, or a file path for local repositories.path/to/directory
: This specifies where you want the clone to be stored on your local machine. If this is omitted, Git will use the repository’s name as the directory name.
Example output:
Cloning into 'directory'...
remote: Enumerating objects: 100, done.
remote: Counting objects: 100, done.
remote: Compressing objects: 100% (75/75), done.
Receiving objects: 100% (100/100), 20.00 KiB | 2.00 MiB/s, done.
Resolving deltas: 100% (40/40), done.
Use case 2: Clone an existing repository and its submodules
Code:
git clone --recursive remote_repository_location
Motivation:
Some projects include submodules, which are repositories nested inside the main repository. If these aren’t cloned, you might miss out on crucial parts of the project. Cloning recursively ensures you have everything you need to get started without having to initialize submodules manually.
Explanation for every argument:
--recursive
: This flag tells Git to clone not only the main repository but also any submodules associated with it.
Example output:
Cloning into 'repository'...
remote: Enumerating objects: 300, done.
remote: Counting objects: 300, done.
remote: Compressing objects: 100% (230/230), done.
Receiving objects: 100% (300/300), 1.50 MiB | 3.00 MiB/s, done.
Resolving deltas: 100% (100/100), done.
Submodule 'submodule_name' (git://example.com/submodule) registered for path 'submodule_path'
Cloning into 'repository/submodule_path'...
Submodule path 'submodule_path': checked out 'abcdef1234567890abcdef1234567890abcdef12'
Use case 3: Clone only the .git
directory of an existing repository
Code:
git clone --no-checkout remote_repository_location
Motivation:
There are occasions when you may only be interested in the commit history or need to manipulate the repository without an initial checkout of files. This approach is faster, especially for large repositories, because it avoids copying file contents.
Explanation for every argument:
--no-checkout
: This option prevents the default checkout of the HEAD. Instead, it clones the directory without placing files in the working directory.
Example output:
Cloning into 'repository'...
remote: Enumerating objects: 350, done.
remote: Counting objects: 350, done.
remote: Compressing objects: 100% (300/300), done.
Receiving objects: 100% (350/350), 1.50 MiB | 3.00 MiB/s, done.
Resolving deltas: 100% (150/150), done.
Use case 4: Clone a local repository
Code:
git clone --local path/to/local/repository
Motivation:
Duplicating a repository on the same filesystem can be crucial when performing experimental changes or maintaining different versions in parallel development environments without affecting the original repository.
Explanation for every argument:
--local
: This flag utilizes hard links to objects under.git/objects/
, saving storage space and time when cloning from the same filesystem.
Example output:
Cloning into 'repository'...
done.
Use case 5: Clone quietly
Code:
git clone --quiet remote_repository_location
Motivation:
In scripting or automated environments, you might want to suppress the detailed output typically produced by Git commands to maintain clean logs or prevent unnecessary console output.
Explanation for every argument:
--quiet
: This lowers the verbosity of the output typically given by the clone process, only reducing it to warnings and errors.
Example output:
(No output is provided unless there are warnings or errors.)
Use case 6: Clone an existing repository only fetching the 10 most recent commits on the default branch
Code:
git clone --depth 10 remote_repository_location
Motivation:
Fetching the entire history of a large project can be resource-intensive. If you only need recent changes, shallow cloning is an efficient way to save bandwidth and speed up the cloning process.
Explanation for every argument:
--depth 10
: This limits the history to the past 10 commits on the default branch, creating a shallow clone.
Example output:
Cloning into 'repository'...
remote: Enumerating objects: 40, done.
remote: Counting objects: 40, done.
remote: Compressing objects: 100% (30/30), done.
Receiving objects: 100% (40/40), 1.20 MiB | 4.00 MiB/s, done.
Resolving deltas: 100% (10/10), done.
Use case 7: Clone an existing repository only fetching a specific branch
Code:
git clone --branch name --single-branch remote_repository_location
Motivation:
When working on a particular feature or exploring a specific branch, there is no need to clone the entire repository with all its branches. This selective approach helps you stay focused and organized.
Explanation for every argument:
--branch name
: Specifies which branch to clone.--single-branch
: Limits fetching to a single branch, ignoring others during clone.
Example output:
Cloning into 'repository'...
remote: Enumerating objects: 40, done.
remote: Counting objects: 40, done.
remote: Compressing objects: 100% (30/30), done.
Receiving objects: 100% (40/40), 1.00 MiB | 4.00 MiB/s, done.
Resolving deltas: 100% (10/10), done.
Use case 8: Clone an existing repository using a specific SSH command
Code:
git clone --config core.sshCommand="ssh -i path/to/private_ssh_key" remote_repository_location
Motivation:
Security requirements or multiple account scenarios often necessitate the use of specific SSH keys. This command allows you to instruct Git to use a particular SSH configuration for cloning.
Explanation for every argument:
--config core.sshCommand="ssh -i path/to/private_ssh_key"
: Configures a specific command for SSH operations, particularly useful for setting a custom identity file for authentication.
Example output:
Cloning into 'repository'...
remote: Enumerating objects: 60, done.
remote: Counting objects: 60, done.
remote: Compressing objects: 100% (55/55), done.
Receiving objects: 100% (60/60), 2.00 MiB | 5.00 MiB/s, done.
Resolving deltas: 100% (30/30), done.
Conclusion:
Mastering the use of git clone
with its various options can significantly improve your workflow efficiency and adaptability when it comes to handling repositories in different scenarios. Whether you need to work with submodules, manage sparse checkouts, or configure specific SSH commands, understanding these use cases empowers you to execute precise and effective cloning operations.