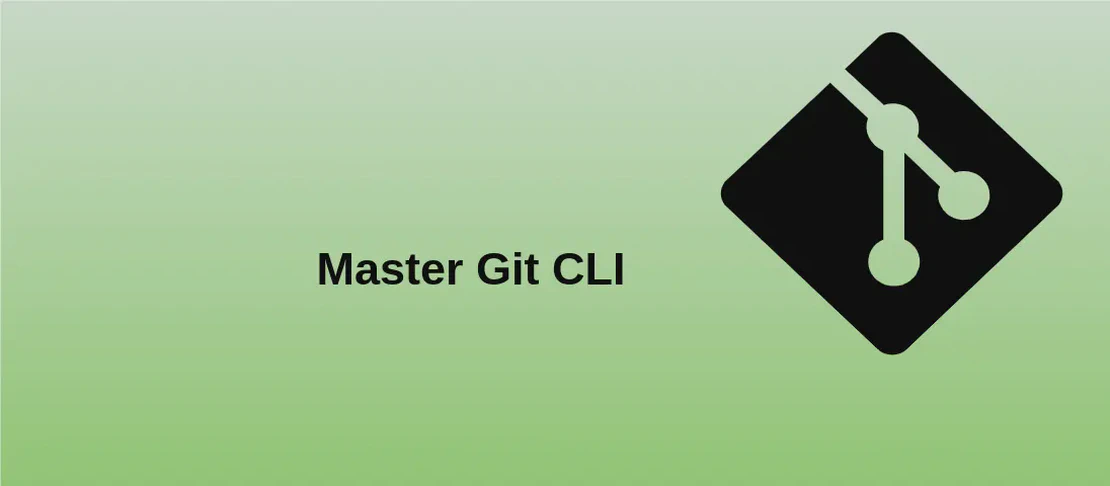
How to use the command 'git commit' (with examples)
The git commit
command is a fundamental part of using the Git version control system. It is used to record changes to the local repository. Committing files creates a checkpoint in the development history, allowing you to keep track of progress and revisit previous versions of your code if necessary. A commit is often accompanied by a message that describes the changes made, helping team members understand the project’s evolution over time.
Commit staged files to the repository with a message
Code:
git commit --message "Add initial project files"
Motivation:
This form of the git commit
command is very commonly used when you have staged files that are ready to be permanently added to your project’s history and you want to document what the changes involve. By including a message, you provide context about this specific checkpoint in your project, which is useful for both personal reference and collaboration with others.
Explanation:
--message
: This flag allows you to include a short, descriptive message that explains the changes in this commit.
Example output:
[main f3aef57] Add initial project files
5 files changed, 100 insertions(+), 0 deletions(-)
Commit staged files with a message read from a file
Code:
git commit --file path/to/commit_message_file
Motivation:
Reading a commit message from a file can be advantageous when the message is too lengthy or complex to be passed as a command-line argument. It also allows for collaboration, where a commit message can be drafted by one team member and reviewed by others before committing.
Explanation:
--file
: This flag specifies the path to a file that contains your commit message. This is particularly useful for commit messages requiring detailed explanations or multiple points.
Example output:
[main 8c7d45a] Feature update with detailed explanation
10 files changed, 250 insertions(+), 20 deletions(-)
Auto stage all modified and deleted files and commit with a message
Code:
git commit --all --message "Fix bugs and clean up code"
Motivation:
This command is ideal when you’ve made numerous changes across different files and wish to efficiently stage and commit them all in one step. This ensures that any modifications or deletions are not missed out, providing a comprehensive update to your repository.
Explanation:
--all
: Automatically stages all tracked files that are modified or deleted. It’s a convenient way of ensuring that any file changes are prepared for the commit.--message
: As before, this is used to specify the commit message.
Example output:
[main 4b92033] Fix bugs and clean up code
7 files changed, 36 insertions(+), 15 deletions(-)
Commit staged files and sign them with the specified GPG key
Code:
git commit --gpg-sign key_id --message "Secure commit with GPG"
Motivation:
Signing commits with a GPG key adds a layer of authenticity and security, ensuring that the commit was indeed made by you and hasn’t been tampered with. This is especially important in collaborative projects or open-source communities where maintaining code integrity is vital.
Explanation:
--gpg-sign key_id
: Uses the specified GPG key to sign the commit. If no key ID is provided, Git will use the default GPG key configured for your user.--message
: Includes a brief message explaining the purpose of the commit.
Example output:
[main d1e692b] Secure commit with GPG
gpg: Signature made Mon 03 Oct 2023 02:04:15 PM UTC
gpg: using RSA key ABCD1234EF56789G
gpg: Good signature from "Your Name <you@example.com>"
Update the last commit by adding the currently staged changes, changing the commit’s hash
Code:
git commit --amend
Motivation:
The --amend
option is beneficial when you want to alter the most recent commit, perhaps by adding forgotten changes or refining the commit message. This resolves minor issues without cluttering your project’s history with multiple corrective commits.
Explanation:
--amend
: Applies the currently staged changes to the last commit, updating it. Note that this will change the commit’s hash.
Example output:
[main 3a2e9f8] Update feature code
Date: Mon Oct 3 14:10:23 2023 +0000
Commit only specific (already staged) files
Code:
git commit path/to/file1 path/to/file2 --message "Update specific components"
Motivation:
This approach is suited to situations where you only want to commit certain files that have been staged, allowing for selective commits. This is useful for restructuring commit content to logically separate changes across different areas of your code, enhancing readability and history tracking.
Explanation:
path/to/file1 path/to/file2
: Specifies the exact files that should be included in the commit.--message
: Adds a message describing the committed modifications.
Example output:
[main 7f6aebc] Update specific components
2 files changed, 30 insertions(+), 5 deletions(-)
Create a commit, even if there are no staged files
Code:
git commit --message "Establish project structure" --allow-empty
Motivation:
Creating an empty commit may seem counterintuitive at first, but it’s quite practical for adding a marker in your project history, such as tagging the start of a new feature or denoting a significant milestone, without necessarily tying it to specific file changes.
Explanation:
--message
: Provides context or information about this empty commit.--allow-empty
: Permits the creation of a commit even if there are no changes to stage.
Example output:
[main e2345ab] Establish project structure
Conclusion:
The git commit
command is very rich in functionality, allowing developers to maintain a well-documented and structured history of their projects. Through various flags and options, users can tailor the command to fit different scenarios, whether it’s making quick fixes, ensuring project security, or marking important project milestones. Understanding and utilizing these options correctly can significantly enhance the development workflow, making code management and collaboration more effective.