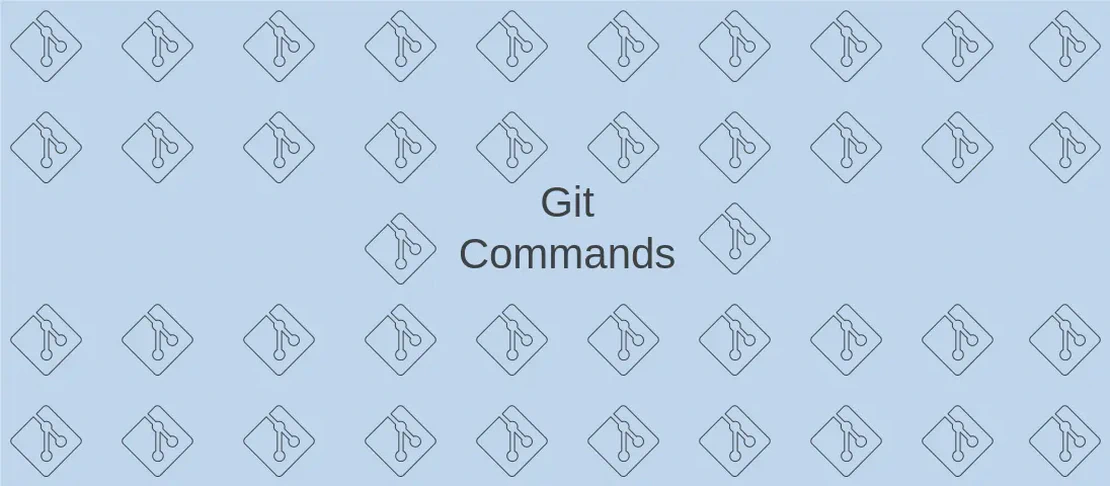
How to Use the Command 'git commit-tree' (with Examples)
The git commit-tree
command is a low-level utility in Git that allows you to manually create commit objects. This command provides granular control over how you construct a commit by allowing you to specify the tree, message, and parent commit directly. While it’s less commonly used than the more user-friendly git commit
command, git commit-tree
is invaluable for advanced version control operations that require a higher degree of customization.
Use Case 1: Create a Commit Object with the Specified Message
Code:
git commit-tree tree -m "message"
Motivation:
This use case is useful when you need to create a commit object programmatically, outside the scope of a typical git commit
process, potentially during scripting or automation tasks. By explicitly specifying the tree and message, you can ensure that the commit accurately reflects the changes you intend to register, with no window for an interactive interface that may introduce errors or delays.
Explanation:
tree
: This represents the SHA-1 hash of the tree object that you wish to commit. The tree object contains the state of the directories and contents.-m "message"
: This flag allows you to directly specify the commit message, which documents the purpose or changes included in the commit, essential for tracking the history of an evolving project.
Example Output:
de5f3c7dd9c7fc5d9f4efb6c5e8adefc8c8595df
In this example, the output is the commit SHA-1 hash of the newly created commit object.
Use Case 2: Create a Commit Object Reading the Message from a File
Code:
git commit-tree tree -F path/to/file
Motivation:
Sometimes, commit messages can be quite detailed or canonicalized. Storing them in a file before creating a commit can enhance organization and readability. This method is particularly beneficial when creating commit messages from automated processes, where reading from files can ensure consistency and reduce potential errors.
Explanation:
tree
: Again, this represents the SHA-1 hash of the tree object.-F path/to/file
: This flag indicates that the commit message should be read from the specified file. The message contained in the file should ideally be well-structured and ready for direct application.
Example Output:
fa4b6d78dde364f40fa56c3fb57979e4dfa877e2
The output is the commit SHA-1 hash, confirming the creation of the commit object with the message read from the file.
Use Case 3: Create a GPG-Signed Commit Object
Code:
git commit-tree tree -m "message" --gpg-sign
Motivation:
With increasing concerns over security and integrity in software projects, GPG-signing commits has become a best practice. This use case demonstrates how to sign a commit object cryptographically, ensuring its authenticity and undue tampering during its lifespan. This practice is crucial for projects that prioritize a secure development environment.
Explanation:
tree
: The SHA-1 hash of the tree object to be committed.-m "message"
: The commit message, which should be concise yet informative.--gpg-sign
: This flag instructs Git to cryptographically sign the commit using GPG (GNU Privacy Guard), adding a layer of security by confirming the authenticity of the commit with a digital signature.
Example Output:
gpg: Signature made Fri 15 Oct 2023 03:45:23 AM PDT using RSA key ID ABC1234
gpg: Good signature from "Your Name <youremail@example.com>"
9f8ec1e710de8e811f4bc5a88d10b7d36e9cb660
The outputs indicate a successfully signed commit, alongside the SHA-1 hash.
Use Case 4: Create a Commit Object with the Specified Parent Commit Object
Code:
git commit-tree tree -m "message" -p parent_commit_sha
Motivation:
In Git’s history, each commit typically references one or more parent commits, maintaining a linked chronology of changes. This use case specifically sets the parent for the new commit, which might be necessary when orchestrating specific branching strategies or rebasing efforts manually.
Explanation:
tree
: The SHA-1 hash depicting the state tree you’re committing.-m "message"
: The commit message.-p parent_commit_sha
: This parameter specifies the SHA-1 hash of the parent commit. It tells Git which commit this new commit should follow in the history. It is essential for branching and merging strategies where continuity is a must.
Example Output:
b7d34567eab9289756e04ddf5a1dbad9c1234567
The above output is the SHA-1 hash for the new commit with the parent commit set as specified.
Conclusion:
The git commit-tree
command provides an advanced level of control for users needing to create commit objects manually. Whether you’re working on automated processes, complex merging strategies, ensuring security through GPG signing, or precise commit tracking, each of these use cases demonstrates the versatility and power of this command. While primarily leveraged by advanced users and for specific use cases, mastering git commit-tree
can vastly improve how you tailor and manage Git repositories in specialized scenarios.