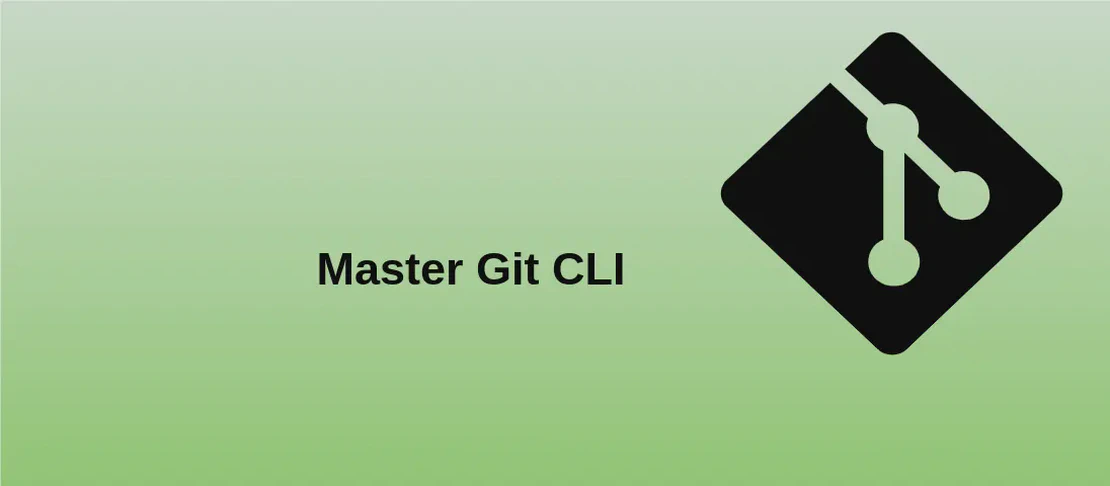
How to use the command 'git' (with examples)
Git is a distributed version control system widely used for tracking changes in source code during software development. Its primary aim is to manage projects with speed, efficiency, and precision. With Git, multiple developers can work on the same project simultaneously, see changes made by others, and revert to previous versions if necessary. The flexibility and functionality of Git make it an indispensable tool in any developer’s toolkit.
Execute a Git subcommand
Code:
git subcommand
Motivation: Software development often requires specific actions to be carried out on a repository, such as committing changes, checking the status of the repository, or viewing the history of modifications. Each of these actions is performed using a “subcommand.” The ability to execute subcommands is fundamental as it enables developers to perform different tasks on a project depending on what is required at each stage of development.
Explanation:
git
: This is the command-line tool used to interact with Git repositories.subcommand
: This placeholder represents a task you want to execute, such ascommit
,add
, orstatus
. Each subcommand allows you to perform specific actions related to your source code management needs.
Example Output:
For a typical subcommand like git status
, the output might be:
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
Execute a Git subcommand on a custom repository root path
Code:
git -C path/to/repo subcommand
Motivation:
In a software development environment, you might have multiple projects stored in different directories on your machine. You may wish to execute Git commands on a repository that is not located in your current working directory. The -C
option allows you to specify the custom location of the repository, making it convenient to manage multiple repositories from a single terminal session without having to navigate to each project’s directory individually.
Explanation:
git
: The command-line interface for Git.-C path/to/repo
: This option tells Git to change to the specified directory before executing the given subcommand.path/to/repo
should be replaced by the path to the repository you want to work on.subcommand
: Represents the Git operation you want to perform on the specified repository.
Example Output:
Running git -C /home/user/projects/world-domination init
will output:
Initialized empty Git repository in /home/user/projects/world-domination/.git/
Execute a Git subcommand with a given configuration set
Code:
git -c 'config.key=value' subcommand
Motivation: Occasionally, you need to temporarily override configuration settings without changing the global or local Git configuration files. For example, you might want to specify a different user name or email for a commit in a collaborative project. This flexibility is vital for making context-specific changes that don’t affect the overall setup of the Git environment.
Explanation:
git
: This accesses the Git software.-c 'config.key=value'
: A flag that allows you to specify a temporary configuration setting for the duration of that command. Replaceconfig.key
with the actual configuration key andvalue
with the desired setting.subcommand
: Indicates the specific Git operation to perform using the specified configuration settings.
Example Output:
Running git -c 'user.name=temporary_user' commit -m "Test commit"
might yield:
[main c8e8bfe] Test commit
1 file changed, 0 insertions(+), 0 deletions(-)
create mode 100644 test.txt
Display help
Code:
git --help
Motivation:
With numerous commands and options, Git can seem overwhelming to new users. The --help
command provides guidance and reference material directly from the terminal, making it a go-to resource when you’re unsure about specific commands or usage parameters.
Explanation:
git
: Activates the Git tool.--help
: This argument displays general help and guidance documentation for using Git, covering various subcommands and options available.
Example Output:
usage: git [--version] [--help] [-C <path>] [-c name=value]
[--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p | --paginate | -P | --no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
<command> [<args>]
The most commonly used git commands are:
add Add file contents to the index
bisect Use binary search to find the commit that introduced a bug
branch List, create, or delete branches
checkout Switch branches or restore working tree files
'git help -a' and 'git help -g' list available subcommands and some
concept guides. See 'git help <command>' or 'git help <concept>'
to read about a specific subcommand or concept.
Display help for a specific subcommand
Code:
git help subcommand
Motivation: When working with Git, you often need detailed instructions or examples for specific subcommands. Understanding how a particular subcommand works can improve workflow efficiency and prevent errors. By accessing subcommand-specific help documentation, users can learn about the options and flags available for their specific needs.
Explanation:
git
: Engages Git’s command-line interface.help subcommand
: Asks Git to show help documentation for the specified subcommand. Replacesubcommand
with the name of the Git command you need information on.
Example Output:
Running git help clone
might produce an output like:
NAME
git-clone - Clone a repository into a new directory
SYNOPSIS
git clone [--template=<template_directory>]
[-l | --local] [--no-hardlinks] [-s | --shared]
[-q | --quiet] [-n | --no-checkout] [--progress]
[--bare] [--mirror]
...
Display version
Code:
git --version
Motivation: Knowing which version of Git you are using can be crucial for compatibility with software tools or ensuring that specific features are available. As Git gets updated, new functionalities, optimizations, and fixes become available in newer versions. Identifying your installed version helps manage expectations and guides you on when to perform upgrades.
Explanation:
git
: The command to interact with Git.--version
: This flag displays the current version of Git installed on your machine.
Example Output:
git version 2.34.1
Conclusion:
By mastering these Git usage patterns, you can tailor your source control activities for efficiency and precision. Each specific command, from executing basic commands to adjusting configuration settings, empowers you to manage and maintain your repositories effectively, ensuring high productivity in development environments.