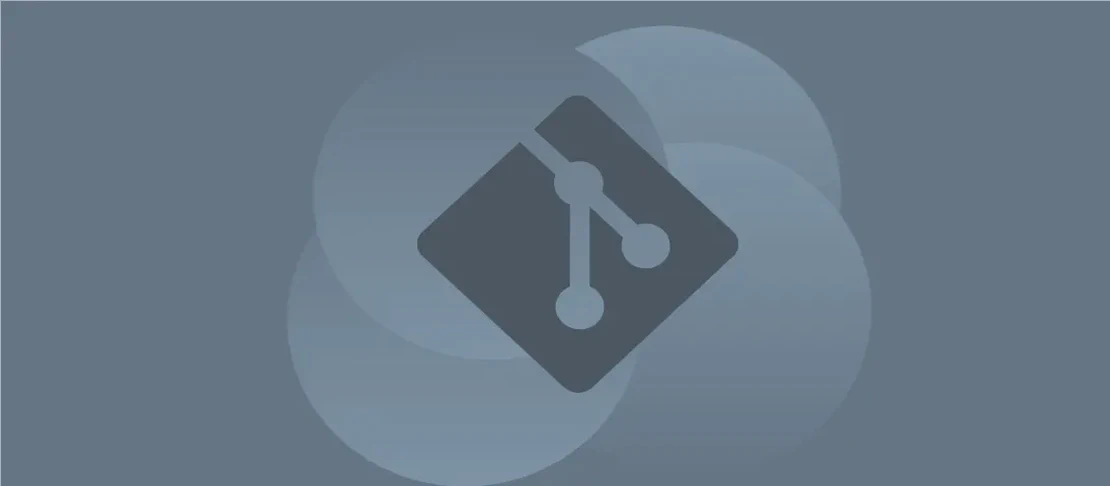
How to use the command 'git config' (with examples)
The git config
command in Git is a versatile tool that allows users to manage configurations, which can be specific to the local repository, globally for the overall user environment, or system-wide. These configurations dictate various aspects of how Git operates, such as user identity, aliasing commands, and more. Configurations can be adjusted for individual projects, or set globally to serve as the default across all repositories.
Use case 1: Globally set your name or email
Code:
git config --global user.name "Your Name"
git config --global user.email "email@example.com"
Motivation:
Setting your name and email globally is essential in Git because this information is attached to your commits. It serves as a digital signature for developers, ensuring that your work can be easily identified in collaboration and tracking. Many open-source projects require contributors to have a verifiable identity, and globally setting these configurations ensures consistency across all your repositories without needing to redefine them each time.
Explanation:
git config
: Calls the configuration management tool.--global
: Specifies that the configuration applies to all repositories for the current user.user.name
: Refers to the configuration entry for the user’s name."Your Name"
: The value being set as the user’s name.user.email
: Refers to the configuration entry for the user’s email."email@example.com"
: The value being set as the user’s email address.
Example output:
(no output on success, just sets configuration)
Use case 2: List local or global configuration entries
Code:
git config --list --local
git config --list --global
Motivation:
Listing configuration entries allows users to review their current settings, which is particularly useful when debugging issues or ensuring that certain configurations have been set correctly. Whether local or global, these listings help verify the state of your environment and ensure that your Git operations are configured as intended.
Explanation:
git config
: Calls the configuration management tool.--list
: Displays all relevant configuration settings.--local
: Filters for configurations specific to the current repository.--global
: Filters for configurations applied user-wide across all repositories.
Example output:
user.name=Your Name
user.email=email@example.com
<additional configuration settings>
Use case 3: List only system configuration entries
Code:
git config --list --system --show-origin
Motivation:
System-level configurations affect all users on a machine, making it crucial to know these settings when working in environments where multiple user settings may interfere. Listing these configurations with their file origins aids in pinpointing the source of specific behavior and potential conflicts that arise from overarching rules.
Explanation:
git config
: Calls the configuration management tool.--list
: Displays configuration settings.--system
: Targets configuration settings stored at the system level (/etc/gitconfig
).--show-origin
: Includes the file locations of each configuration entry.
Example output:
file:/etc/gitconfig diff.external=meld
file:/etc/gitconfig core.editor=vim
<additional system configuration settings>
Use case 4: Get the value of a given configuration entry
Code:
git config alias.unstage
Motivation:
Fetching a specific configuration value is important for checking the state of an entry without having to sift through a complete list. This is particularly useful for aliases or environment settings where precise values can alter workflows significantly, such as command behavior or preferred user interfaces.
Explanation:
git config
: Calls the configuration management tool.alias.unstage
: The specific configuration entry being queried.
Example output:
reset HEAD --
Use case 5: Set the global value of a given configuration entry
Code:
git config --global alias.unstage "reset HEAD --"
Motivation:
Creating aliases for complex or frequently used commands enhances efficiency by reducing the effort required for command input, reducing the likelihood of errors in command usage, and making scripts or commands more readable. By setting such an alias globally, all repositories benefit from these shorthand custom commands.
Explanation:
git config
: Calls the configuration management tool.--global
: Specifies that this alias configuration applies to all repositories for the current user.alias.unstage
: Names the configuration entry for an alias."reset HEAD --"
: The command that the aliasunstage
represents, streamlining the process of undoing staged changes.
Example output:
(no output on success, just sets configuration)
Use case 6: Revert a global configuration entry to its default value
Code:
git config --global --unset alias.unstage
Motivation:
Reverting configurations to their defaults is crucial when certain personalized settings no longer serve a purpose or cause conflict. This command simplifies the reset process without manually editing configuration files, ensuring user’s environments quickly return to their initial state.
Explanation:
git config
: Calls the configuration management tool.--global
: Indicates that the action pertains to global configurations.--unset
: Removes the specified configuration entry.alias.unstage
: The specific configuration entry being reset.
Example output:
(no output on success, just unsets configuration)
Use case 7: Edit the local Git configuration
Code:
git config --edit
Motivation:
Manually editing the configuration files allows for precise, bulk changes and the adjustment of configurations not easily manipulated through the command line. This is an invaluable resource for advanced users looking to configure options beyond standard documentation or for quick, multi-entry modifications.
Explanation:
git config
: Calls the configuration management tool.--edit
: Opens the local.git/config
file for editing in the user’s default text editor.
Example output:
(opens the configuration file in the default editor)
Use case 8: Edit the global Git configuration
Code:
git config --global --edit
Motivation:
Editing the global configuration file directly provides comprehensive access to all user-wide settings, allowing for holistic view and modification of the developer’s Git environment. This can be essential for resolving variable dependencies, updating out-of-date configurations, or streamlining workflows through cohesive settings.
Explanation:
git config
: Calls the configuration management tool.--global
: Specifies the target as global configurations.--edit
: Opens the~/.gitconfig
(or$XDG_CONFIG_HOME/git/config
) file for editing.
Example output:
(opens the configuration file in the default editor)
Conclusion:
The git config
command is fundamental for tailoring the Git experience to suit individual developer needs or organizational standards. Understanding each use case, from setting personal information to adjusting command behaviors with aliases, allows for more organized and efficient version control. Whether working across multiple repositories or needing specific configurations for a single project, these commands foster a controlled, customized, and optimized development environment.