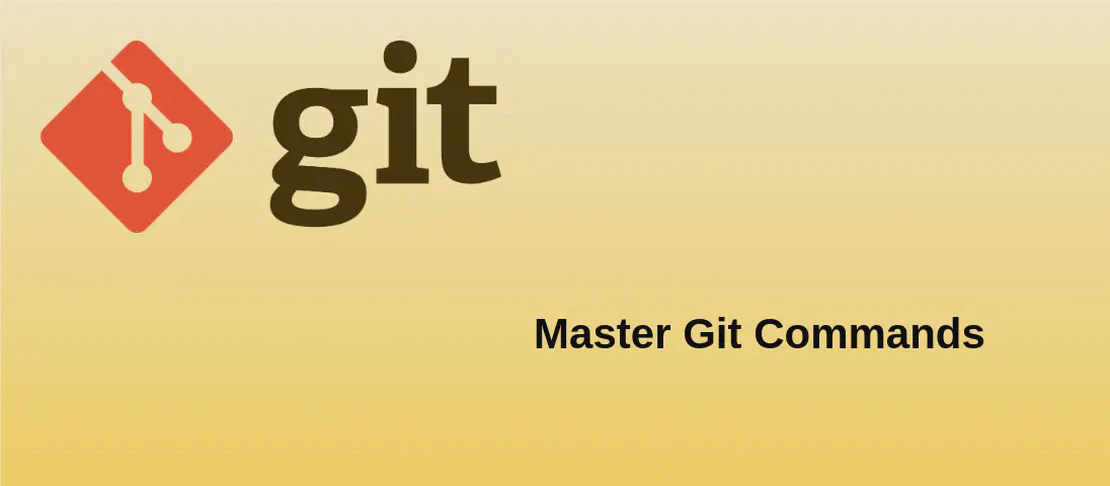
How to use the command 'git create-branch' (with examples)
The git create-branch
command is part of the git-extras
suite, which provides additional functionality for easier and more efficient Git use. This particular command simplifies the process of creating branches both locally and on remote repositories. In software development and version control, branches are used to isolate work on a specific feature or bug fix, which allows developers to collaborate without interfering with the main codebase until the feature or fix is finalized. This article illustrates different scenarios of using the git create-branch
command, providing practical examples and detailed explanations.
Use case 1: Create a local branch
Code:
git create-branch branch_name
Motivation:
Creating a local branch is a fundamental task in any Git workflow. It allows developers to isolate their work in a separate branch without affecting the main branch. This is particularly useful when working on a new feature, as it lets you freely experiment and develop without risk to the stable version of your codebase. By simply creating a local branch, you can test your changes, make commits, and even discard your work if necessary, without impacting others.
Explanation:
git create-branch
: This is the command that initiates the creation of a new branch.branch_name
: This is a placeholder for the name of the branch you want to create. Replace it with a descriptive name for your branch to indicate the work or feature associated.
Example Output:
After executing this command, the terminal will typically show confirmation of the branch creation. You’ll be switched to the new branch, with output similar to:
Switched to a new branch 'branch_name'
Use case 2: Create a branch locally and on origin
Code:
git create-branch --remote branch_name
Motivation:
Sometimes, the branch you’re working on needs to be available to other team members. In such cases, creating it only locally is not sufficient. Creating a branch on both local and origin ensures that your branch is available on a remote repository from the onset, facilitating team collaboration. This is essential when multiple developers need to pull changes from the same branch, or when you need to run continuous integration/continuous deployment pipelines.
Explanation:
git create-branch
: Initiates creation of a branch.--remote
: This option tells Git to not only create the branch locally but also push it to the remote ‘origin’, typically the default remote pointing to the main repository.branch_name
: The name of the branch for identification and future reference.
Example Output:
Executing this command will create the branch locally and push it to the origin. You’ll see output confirming the creation and the push, like:
Switched to a new branch 'branch_name'
Branch 'branch_name' set up to track remote branch 'branch_name' from 'origin'.
Use case 3: Create a branch locally and on upstream (through forks)
Code:
git create-branch --remote upstream branch_name
Motivation:
In open-source projects and collaborative environments where forks are used, upstream
typically refers to the original repository from which the fork was made. Creating a branch on both the local copy and the upstream ensures that changes are synchronized and can be pushed directly to the authoritative repository. This use case is particularly valuable when contributing to an upstream project, as it streamlines the process of proposing changes to the original project that are housed in another repository.
Explanation:
git create-branch
: This initializes the creation of a new branch.--remote upstream
: This specifies that the branch should be created on both the local repository and the identified remote, ‘upstream’. It’s a more advanced command used where the workflow involves interaction with a parent repository beyond the immediate origin.branch_name
: Denotes the identifier for the branch being created.
Example Output:
Upon execution, this command will prepare the branch and align it with the upstream repository, demonstrating both local creation and remote arrangement:
Switched to a new branch 'branch_name'
Branch 'branch_name' set up to track remote branch 'branch_name' from 'upstream'.
Conclusion:
The git create-branch
command under git-extras
provides a streamlined approach to branching, offering ease of creating branches locally, on origin, and upstream depending on the workflows in place. Whether you’re working alone, committing to team projects, or interacting with open-source projects, each use case demonstrates how you can optimize your branching strategy for efficient version control management.