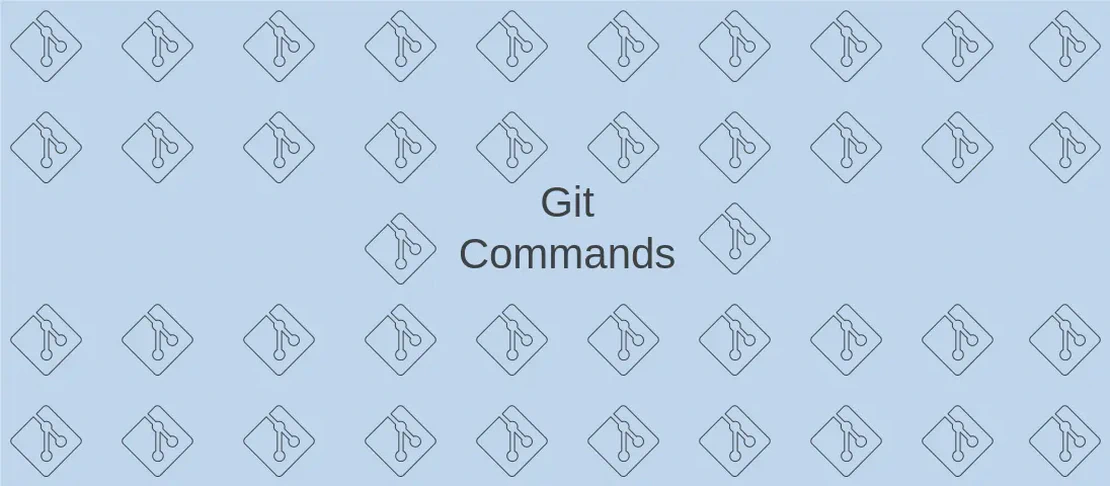
How to Use the Command 'git describe' (with Examples)
The git describe
command is a powerful tool in Git that provides a human-readable name to a commit, which is especially useful when dealing with long strings of SHA hashes. This command uses a system to label a commit based on the closest annotated tag reachable from it, which helps in maintaining a more meaningful identification of commits, facilitating easier navigation and comprehension within a project’s repository. Essentially, it enhances the traceability of code changes by combining tags, commit counts, and abbreviated hashes into an understandable form.
Use Case 1: Create a Unique Name for the Current Commit
Code:
git describe
Motivation:
Using the git describe
command without any additional options helps in creating a unique identifier for the current commit. This identifier is formulated based on the closest annotated tag, the number of commits since that tag, and an abbreviated version of the commit hash. It’s particularly helpful when you need to communicate or document a specific commit to collaborate with team members or when tracking changes across builds and deployments.
Explanation:
git describe
: Invokes the command to generate a human-readable name for the current commit by default. It automatically identifies the closest annotated tag from the current checked-out commit, counts subsequent commits, and appends an abbreviated commit hash to create a unique descriptor.
Example Output:
v1.2.3-10-gabcd123
This output indicates the closest annotated tag is v1.2.3
, there are 10 additional commits after this tag, and the abbreviated commit hash is gabcd123
.
Use Case 2: Create a Name with 4 Digits for the Abbreviated Commit Hash
Code:
git describe --abbrev=4
Motivation:
There are moments when it becomes essential to maintain a stable yet shorter identifier for a commit. By specifying the --abbrev
parameter, you control the length of the abbreviated commit hash, which can help in scenarios where space is limited or when you require consistent hash lengths across various outputs for comparison reasons.
Explanation:
--abbrev=4
: This option requests that the abbreviated commit hash consist of 4 digits. The abbreviation length can be tailored to suit specific needs, which can be integral when ensuring unique short hash representations in larger projects.
Example Output:
v1.2.3-10-gabcd
In this output, the commit descriptor is similar to the prior example but with a shortened hash length restricted to four characters.
Use Case 3: Generate a Name with the Tag Reference Path
Code:
git describe --all
Motivation:
Simply identifying a commit from a branch’s local perspective might not be sufficient in certain complex workflow scenarios. Employing the --all
option traces through all references, including branches and remote-tracking branches, offering more context about where in the repository the commit resides. This is useful in extensive repositories with multiple active branches, allowing users to differentiate where exactly a commit exists.
Explanation:
--all
: Directsgit describe
to search through all references, not just annotated tags, providing a descriptor that includes branch names and other reference paths necessary to reach the commit.
Example Output:
heads/master-10-gabcd123
This output indicates the commit is on the master
branch, followed by 10 subsequent commits since a key event, and ends with the abbreviated commit hash.
Use Case 4: Describe a Git Tag
Code:
git describe v1.0.0
Motivation:
In many development workflows, it becomes necessary to verify details anchored to specific tags, especially when tags mark milestones, releases, or specific states of the project. Using git describe
with an explicit tag name makes it convenient to get details about that particular tag and its correlation to other commits in a comprehensible form.
Explanation:
v1.0.0
: By providing a specific tag name togit describe
, the command produces context about this tag relative to other commits. In essence, it allows users to audit and understand the position of a tag in the entire commit history.
Example Output:
v1.0.0
This output simply reaffirms the selected tag v1.0.0
is the latest reference point within the available tagged history.
Use Case 5: Create a Name for the Last Commit of a Given Branch
Code:
git describe branch_name
Motivation:
Users often need to target specific branches directly when generating descriptors, whether for reviewing branch-specific changes, preparing a branch for merging, or managing branch-based deployments and builds. This command option ensures that the naming contextualizes a commit within the scope of a specified branch directly.
Explanation:
branch_name
: By specifying a branch name in thegit describe
command, it calculates and provides a descriptor based on the last commit of that branch, rather than the branch currently checked out or the HEAD state.
Example Output:
v1.2.3-5-gfghj456
This descriptor suggests that the branch’s latest commit follows the tag v1.2.3
by 5 additional commits and highlights it with an abbreviated SHA.
Conclusion:
The git describe
command is a diverse utility in Git’s toolbelt that helps transform cryptic commit hashes into tangible and understandable references. Whether for improving branch documentation, supporting team communication, or enhancing build traceability, mastering its use across different contexts can significantly streamline version control management.