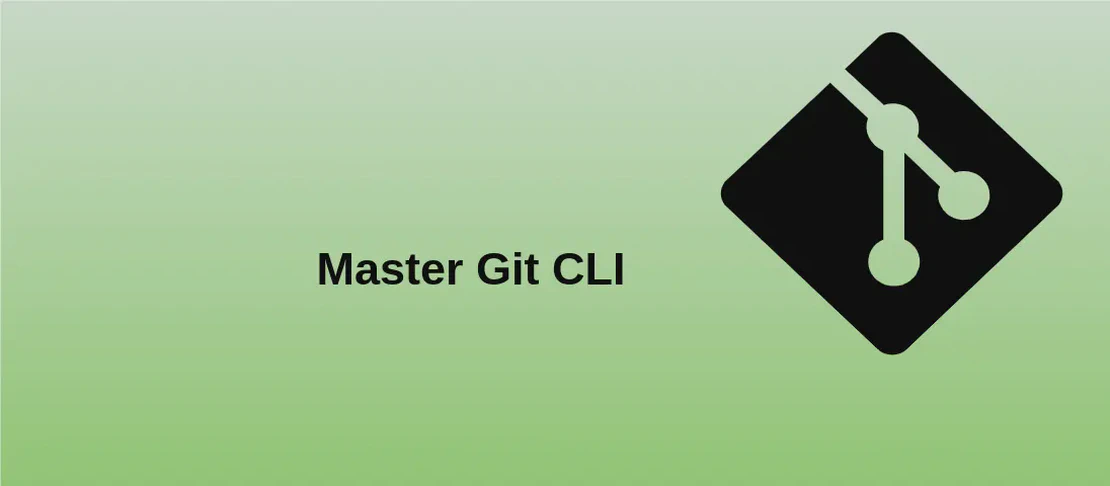
How to Use the Command 'git diff' (with Examples)
The git diff
command is an essential tool for developers working with Git, the distributed version control system. It allows users to view the differences between various states of the codebase. This command helps in identifying changes between commits, branches, working directory, and staging area. Understanding how to use git diff
can greatly improve your ability to manage and troubleshoot code changes effectively.
Use Case 1: Show Unstaged Changes
Code:
git diff
Motivation:
As you work on your code, you might frequently make changes that are yet to be added to the staging area. It’s important to review these unstaged changes to ensure that your modifications align with your intentions before staging and committing. Using git diff
, you can conveniently see what changes have been made without prior staging.
Explanation of Arguments:
git diff
: This command by default displays all unstaged changes in your working directory compared to the last commit. It helps you verify what modifications are pending for staging.
Example Output:
diff --git a/file1.txt b/file1.txt
index e69de29..d95f3ad 100644
--- a/file1.txt
+++ b/file1.txt
@@ -0,0 +1 @@
+Added line to file1
The output shows a new line added to file1.txt
that hasn’t been staged yet.
Use Case 2: Show All Uncommitted Changes (Including Staged Ones)
Code:
git diff HEAD
Motivation:
When you have both staged and unstaged changes, it’s crucial to distinguish between them as you prepare to commit. By comparing your current workspace against the HEAD, you get a comprehensive overview of all modifications since the last commit, allowing for careful review and management of the changes.
Explanation of Arguments:
HEAD
: This refers to the last commit, enabling a complete view of differences between the current state of your workspace (both staged and unstaged changes) and the most recent commit.
Example Output:
diff --git a/file1.txt b/file1.txt
--- a/file1.txt
+++ b/file1.txt
@@ -0,0 +1 @@
+Modified line in file1
diff --git a/file2.txt b/file2.txt
new file mode 100644
--- /dev/null
+++ b/file2.txt
@@ -0,0 +1 @@
+Added file2 with initial content
This output illustrates a modification in file1.txt
and the addition of a new file file2.txt
, encompassing both staged and unstaged changes.
Use Case 3: Show Only Staged (Added, But Not Yet Committed) Changes
Code:
git diff --staged
Motivation:
Once you’ve staged your changes, it’s useful to verify exactly what will be included in your next commit. git diff --staged
presents this information, allowing you to catch any potentially unwanted changes before they are permanently recorded in your history.
Explanation of Arguments:
--staged
: This option tellsgit diff
to compare the changes you have staged against the last commit, providing visibility into modifications lined up for the next commit.
Example Output:
diff --git a/file1.txt b/file1.txt
--- a/file1.txt
+++ b/file1.txt
@@ -0,0 +1 @@
+Ready to commit line in file1
The result shows only the changes that are currently staged, ensuring readiness for the upcoming commit.
Use Case 4: Show Changes from All Commits Since a Given Date/Time
Code:
git diff 'HEAD@{2 weeks ago}'
Motivation:
Tracking changes over a specific period can help in retrospective analysis and understanding the evolution of your codebase. Developers working over extended periods can use this command to review incremental changes made since a particular past date.
Explanation of Arguments:
'HEAD@{2 weeks ago}'
: This expression constructs a reference point relative to the current HEAD, determined by the specified time, showing all changes that have occurred since that time.
Example Output:
diff --git a/file1.txt b/file1.txt
index abc1234..567defg 100644
--- a/file1.txt
+++ b/file1.txt
@@ -1 +1,2 @@
-Old content line
+Updated content line
+New content added
The output here might depict content evolution in file1.txt
over the past two weeks.
Use Case 5: Show Diff Statistics
Code:
git diff --stat commit
Motivation:
While the regular git diff
outputs detailed lines of differences, sometimes a summary of changes is all you need, particularly when you want to understand the scale of changes rather than the specifics. This can help in evaluating the impact of a particular commit.
Explanation of Arguments:
--stat
: This parameter provides summary statistics about the changes, such as the number of files modified, insertions, and deletions.
Example Output:
file1.txt | 3 ++-
file2.txt | 1 +
2 files changed, 3 insertions(+), 1 deletion(-)
Such an output gives a quick overview of changes in terms of affected files and lines.
Use Case 6: Output a Summary of File Creations, Renames, and Mode Changes
Code:
git diff --summary commit
Motivation:
When files are created, renamed, or their access modes are altered, it helps to have a summarized view of these specific changes without going through every line of code. This summary can be crucial in understanding structural changes to your project directories.
Explanation of Arguments:
--summary
: This options produces a concise report on high-level file operations (creation, renaming, mode changes) since a specified commit.
Example Output:
create mode 100644 newfile.txt
rename oldname.txt => newname.txt
This output distinctly shows newly created files and files that have been renamed.
Use Case 7: Compare a Single File Between Two Branches or Commits
Code:
git diff branch_1..branch_2 -- path/to/file
Motivation:
In a multi-branch development environment, you often need to compare specific files across branches to ensure consistency or prepare for merges. This command helps by isolating differences in a targeted file between the selected branches or commits.
Explanation of Arguments:
branch_1..branch_2
: Specifies the two points (branches, tags, or commits) between which the comparison is done.--
: Separates the branches from the file path, ensuring the intended file is compared.
Example Output:
diff --git a/path/to/file b/path/to/file
index 123abc4..567def8 100644
--- a/path/to/file
+++ b/path/to/file
@@ -1,3 +1,3 @@
-Original line in branch_1
+Updated line in branch_2
This depicts changes made to path/to/file
between branch_1
and branch_2
.
Use Case 8: Compare Different Files from the Current Branch to Another Branch
Code:
git diff branch:path/to/file2 path/to/file
Motivation:
Sometimes, you need to compare two entirely different files that exist within different branches. This command allows for such a specific comparison, which is helpful for identifying changes when files might have similar roles but are located individually per branch.
Explanation of Arguments:
branch:path/to/file2
: Specifies the path to the file on the designated branch for comparison.path/to/file
: The corresponding file path on the current branch.
Example Output:
diff --git a/file_on_other_branch b/file_current_branch
--- a/file_on_other_branch
+++ b/file_current_branch
@@ -1,5 +1,5 @@
-Differences in branch file
+Differences in current branch file
This highlights the disparities between two files across different branches.
Conclusion:
These examples reveal the versatility and necessity of the git diff
command as a comprehensive tool for code comparison in version control workflows. Understanding each use case empowers developers to manage changes efficiently, ensuring code integrity and collaboration success. By mastering these functionalities, you’re better equipped to keep track of every modification, ensuring you remain informed and in control within your development environment.