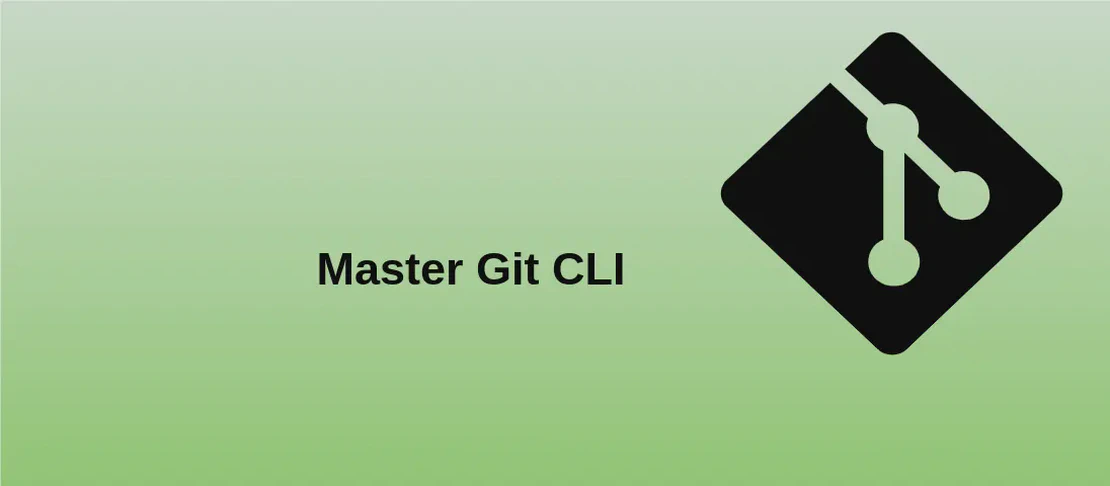
How to Use the Command 'git diff-index' (with examples)
The git diff-index
command is a powerful utility in Git that allows developers to compare their working directory with a specific commit or tree object. This command is useful for identifying changes in the codebase that are yet to be committed or for inspecting modifications in the directory against an index. It’s an invaluable tool for developers who need to keep track of changes in their files and ensure consistency and accuracy in their version control practices.
Use case 1: Compare the working directory with a specific commit
Code:
git diff-index commit
Motivation:
This use case is ideal when you want to see what changes have been made in your current working directory relative to a particular commit. It’s especially useful when you’re reviewing modifications before creating a new commit, ensuring that the changes align with your expectations and project requirements.
Explanation:
git
: This is the command-line interface for Git, a distributed version control system.diff-index
: This subcommand is used to compare the contents of the working directory (or index) against a particular commit or tree object.commit
: Replace this placeholder with the SHA-1 hash of the commit you want to compare with. This identifies the specific point in the repository history that you are using as a reference.
Example Output:
:100644 100644 bcd1234... 0123456... M path/to/file.txt
:100644 100644 234fac6... 7890abc... M path/to/another_file.txt
The output indicates modifications in path/to/file.txt
and path/to/another_file.txt
with their respective status and hash values.
Use case 2: Compare a specific file or directory in the working directory with a commit
Code:
git diff-index commit path/to/file_or_directory
Motivation:
This example is beneficial when you want to focus on changes within a specific file or directory rather than the entire working directory. It allows developers to narrow down their inspection to specific areas of interest, making it easier to manage and understand changes.
Explanation:
git
: Git is used for managing source code and tracking changes.diff-index
: This command enables a comparison between working state and a given commit.commit
: Represents the commit hash to compare against.path/to/file_or_directory
: This specifies the file or directory you’re interested in, allowing targeted comparisons.
Example Output:
:100644 100644 abc1234... def4567... M path/to/specific_file.txt
The output shows that the specified file (path/to/specific_file.txt
) has modifications compared to the given commit.
Use case 3: Compare the working directory with the index (staging area) to check for staged changes
Code:
git diff-index --cached commit
Motivation:
This scenario is frequently employed to check for modifications that have been staged for the next commit but not yet committed. It ensures that any staged changes are only those intended for inclusion in the next commit, thus reducing potential errors.
Explanation:
git
: Git is a system to track changes in text files.diff-index
: Used to perform comparisons.--cached
: This flag tells Git to compare the index (staging area) against the specified commit.commit
: This indicates which commit you’re comparing the staged changes against.
Example Output:
:100644 100644 4567def... 8901ghi... M staged_file.txt
This shows that staged_file.txt
has staged changes compared to the commit.
Use case 4: Suppress output and return an exit status to check for differences
Code:
git diff-index --quiet commit
Motivation:
This approach is particularly useful for automation scripts or continuous integration pipelines where you need to determine if differences exist without cluttering output logs with detailed change summaries.
Explanation:
git
: The command-line client for interacting with Git repositories.diff-index
: This is used for performing the diff operation.--quiet
: Suppresses the output of difference details; it returns an exit status instead.commit
: The commit hash you are checking against to find differences.
Example Output:
Nothing, as the --quiet
option suppresses output. An exit status of 0
means no differences, whereas a non-zero status indicates that changes are present.
Conclusion:
In summary, the git diff-index
command provides developers with several flexible options for comparing their working directories, staged changes, and specific files against commits within a Git repository. By adopting these use cases, developers can better manage and maintain their coding projects, optimizing version control and code review processes. Whether you’re focusing on specific files or need a big-picture view, git diff-index
is an essential tool in any developer’s toolkit.