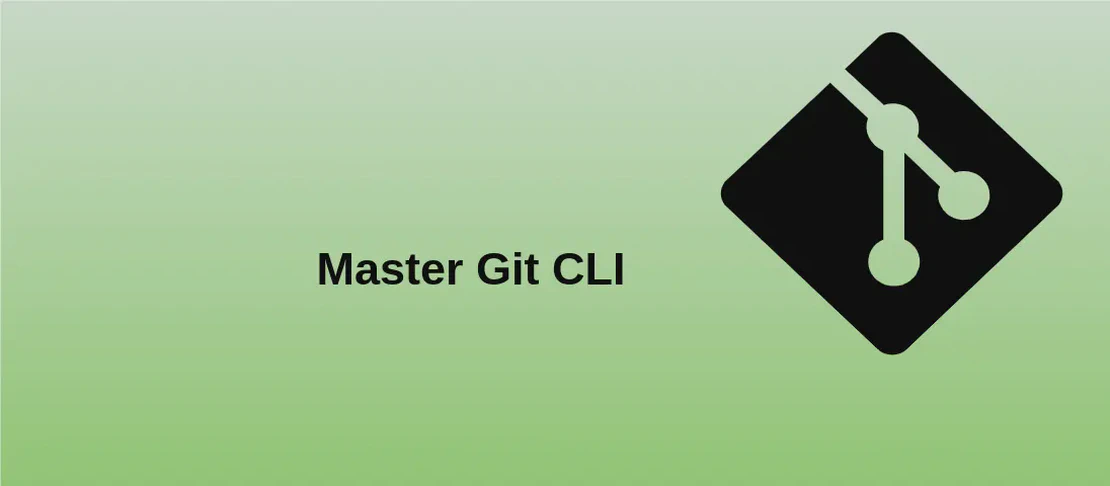
How to Use the Command 'git force-clone' (with examples)
The git force-clone
command is a powerful tool, particularly useful when working with Git repositories. It offers the basic functionality of git clone
but goes a step further by forcefully resetting an existing repository in a target directory to match the remote repository. This functionality is especially helpful in scenarios where maintaining a clean and updated working environment is crucial. Embedded in git-extras
, this aptly named command helps maintain consistency between your local repositories and their remote counterparts.
Use case 1: Clone a Git repository into a new directory
Code:
git force-clone remote_repository_location path/to/directory
Motivation:
You might use this command when you need to start working on a project from scratch, where you want to ensure that the directory into which you’re cloning is new and free from previous data conflicts. By using git force-clone
, you are guaranteed a clean and specific projection of the remote repository in your local system, similar to a simplified version of git clone
.
Explanation:
remote_repository_location
: This argument specifies the URL or location of the remote Git repository that you are cloning. It’s essential because it tellsgit force-clone
where to fetch the data from.path/to/directory
: This represents the destination path on your local machine where you want the repository to be cloned. As part of the basic functionality, if the directory does not exist,git force-clone
will create it for you.
Example output:
Cloning into 'path/to/directory'...
remote: Enumerating objects: 100, done.
remote: Counting objects: 100% (100/100), done.
remote: Compressing objects: 100% (70/70), done.
Receiving objects: 100% (100/100), 1.23 MiB | 3.45 MiB/s, done.
Resolving deltas: 100% (40/40), done.
Use case 2: Clone a Git repository into a new directory, checking out a specific branch
Code:
git force-clone -b branch_name remote_repository_location path/to/directory
Motivation:
This command is useful when you need to work with a specific branch of a project right from the start. This scenario is common when you are working in a team where different members are responsible for different features or bug fixes, and each branch represents specific work in isolation.
Explanation:
-b branch_name
: This flag tellsgit force-clone
which branch to checkout after cloning. It’s crucial when you want your starting environment to reflect the state of a particular branch from the get-go.remote_repository_location
: The URL or path to the remote repository from which the code should be cloned.path/to/directory
: The specified local path where the repository will be created or reset.
Example output:
Cloning into 'path/to/directory'...
remote: Enumerating objects: 150, done.
remote: Counting objects: 100% (150/150), done.
remote: Compressing objects: 100% (110/110), done.
Receiving objects: 100% (150/150), 2.34 MiB | 4.56 MiB/s, done.
Resolving deltas: 100% (50/50), done.
Checking out files: 100% (80/80), done.
Switched to branch 'branch_name'
Use case 3: Clone a Git repository into an existing directory of a Git repository, performing a force-reset to resemble it to the remote and checking out a specific branch
Code:
git force-clone -b branch_name remote_repository_location path/to/directory
Motivation:
This scenario is ideal when you want to ensure an existing local repository mirrors the exact structure and content of a remote repository. Perhaps you’ve made some modifications you’re unsure of, or the existing data might contain inconsistencies. git force-clone
diligently resets the directory, streamlining the updates and minimizing errors.
Explanation:
-b branch_name
: Specifies which branch of the repository you wish to focus on, particularly if the intended tasks are branch-specific.remote_repository_location
: This is where the command fetches information and data from. It is the repository’s URL or local path.path/to/directory
: Reflects the directory path in your local machine, which can already house a Git repository that gets reset to match the remote.
Example output:
Clearing existing repository at 'path/to/directory'...
Cloning into 'path/to/directory'...
remote: Enumerating objects: 200, done.
remote: Counting objects: 100% (200/200), done.
remote: Compressing objects: 100% (150/150), done.
Receiving objects: 100% (200/200), 3.45 MiB | 5.67 MiB/s, done.
Resolving deltas: 100% (60/60), done.
Checking out files: 100% (120/120), done.
Switched to branch 'branch_name'
Conclusion:
The git force-clone
command is a highly versatile tool in the Git toolkit, allowing users to efficiently manage their local repositories with precision and accuracy. By using the command in varying scenarios—such as cloning fresh repositories, focusing on specific branches, or resetting existing local repositories—it empowers users to maintain consistent, up-to-date, and clean working environments. Through its capability to force-reset directories, it especially proves essential in complex workflows that demand meticulous synchronization with remote repositories.